python tutorial - Python Program to find Area of a Rectangle - learn python - python programming
- To write Python Program to find Area of a Rectangle and Perimeter of a Rectangle with example.
- Before we step into the program, Let see the definitions and formulas behind Perimeter and Area Of a Rectangle.
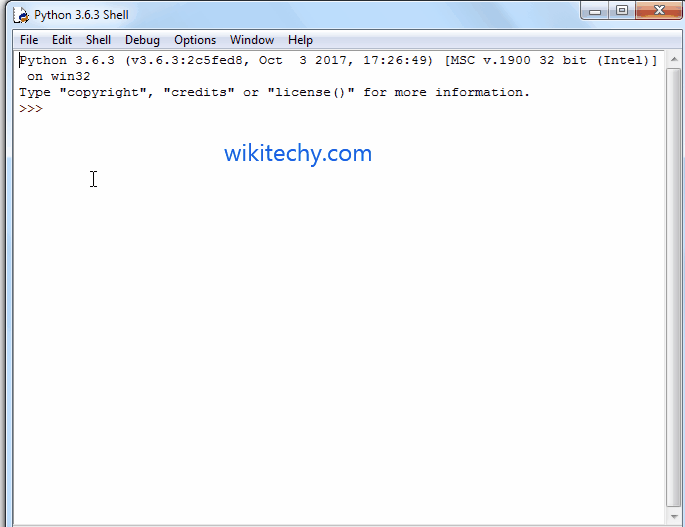
Learn Python - Python tutorial - Python Program to find Area of a Rectangle - Python examples - Python programs
Area of a Rectangle
- If we know the width and height then, we can calculate the area of a rectangle using below formula.
Area = Width * Height
click below button to copy the code. By Python tutorial team
- Perimeter is the distance around the edges. We can calculate perimeter of a rectangle using below formula
Perimeter = 2 * (Width + Height)
click below button to copy the code. By Python tutorial team
Python Program to find Area of a Rectangle and Perimeter of a Rectangle
- This program allows the user to enter width and height of the rectangle. Using those values we will calculate the Area of a rectangle and perimeter of a rectangle.
Sample Code
# Python Program to find Area of a Rectangle
width = float(input('Please Enter the Width of a Rectangle: '))
height = float(input('Please Enter the Height of a Rectangle: '))
# calculate the area
Area = width * height
# calculate the Perimeter
Perimeter = 2 * (width + height)
print("\n Area of a Rectangle is: %.2f" %Area)
print(" Perimeter of Rectangle is: %.2f" %Perimeter)
click below button to copy the code. By Python tutorial team
Output
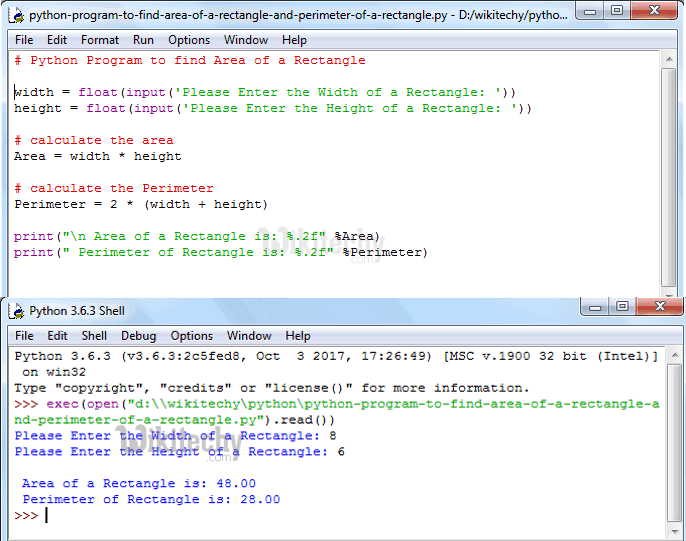
Learn Python - Python tutorial - Python Program to find Area of a Rectangle and Perimeter of a Rectangle - Python examples - Python programs
Analysis
- Following statements will allow the User to enter the Width and Height of a rectangle.
width = float(input('Please Enter the Width of a Rectangle: '))
height = float(input('Please Enter the Height of a Rectangle: '))
click below button to copy the code. By Python tutorial team
- Next, we are calculating the area as per the formula
Area = width * height
click below button to copy the code. By Python tutorial team
- In the next line, We are calculating the Perimeter of a rectangle
Perimeter = 2 * (width + height)
click below button to copy the code. By Python tutorial team
- Following print statements will help us to print the Perimeter and Area of a rectangle
print("\n Area of a Rectangle is: %.2f" %Area)
print(" Perimeter of Rectangle is: %.2f" %Perimeter)
click below button to copy the code. By Python tutorial team
Python Program to find Area of a Rectangle using functions
- This program allows the user to enter the width and height of a rectangle.
- We will pass those values to the function arguments to calculate the area of a rectangle.
Sample Code
# Python Program to find Area of a Rectangle using Functions
def Area_of_a_Rectangle(width, height):
# calculate the area
Area = width * height
# calculate the Perimeter
Perimeter = 2 * (width + height)
print("\n Area of a Rectangle is: %.2f" %Area)
print(" Perimeter of Rectangle is: %.2f" %Perimeter)
Area_of_a_Rectangle(6, 4)
click below button to copy the code. By Python tutorial team
Analysis
- First, We defined the function with two arguments using def keyword.
- It means, User will enter the width and height of a rectangle.
- Next, We are Calculating the perimeter and Area of a rectangle as we described in our first example.
Output
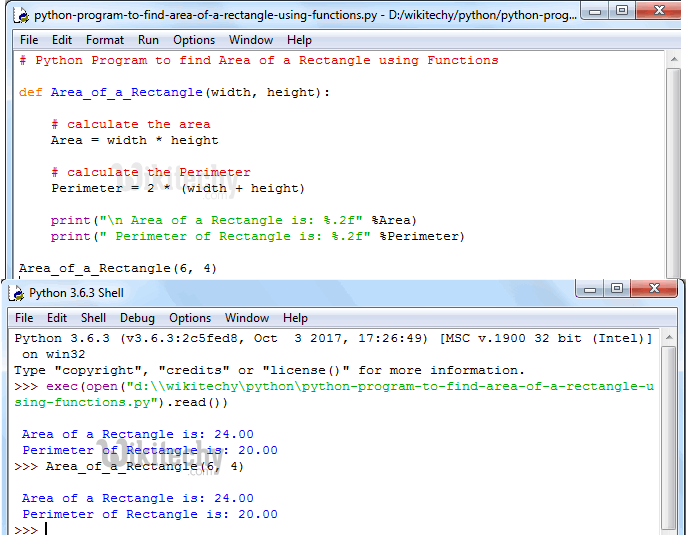
Learn Python - Python tutorial - Python Program to find Area of a Rectangle using functions - Python examples - Python programs
NOTE: We can call the function with arguments in .py file directly or else we can call it from the python shell. Please don’t forget the function arguments