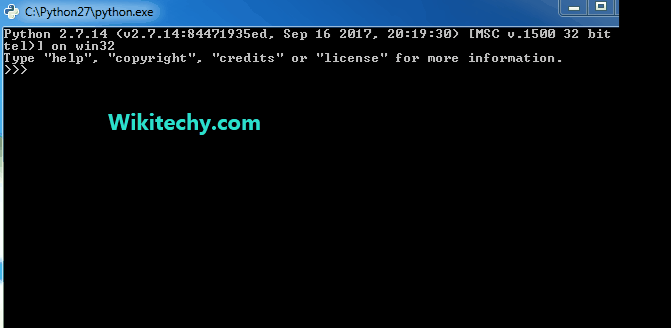
Learn Python - Python tutorial - python string regexp - Python examples - Python programs
The module re provides support for regular expressions in Python. Below are main methods in this module.
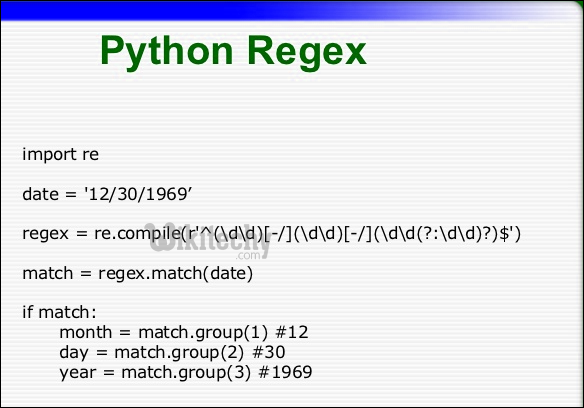
re.search() : This method either returns None (if the pattern doesn’t match), or a re.MatchObject that contains information about the matching part of the string. This method stops after the first match, so this is best suited for testing a regular expression more than extracting data.
python - Sample - python code :
# A Python program to demonstrate working of re.match().
import re
# Lets use a regular expression to match a date string
# in the form of Month name followed by day number
regex = r"([a-zA-Z]+) (\d+)"
match = re.search(regex, "I was born on June 24")
if match != None:
# We reach here when the expression "([a-zA-Z]+) (\d+)"
# matches the date string.
# This will print [14, 21), since it matches at index 14
# and ends at 21.
print "Match at index %s, %s" % (match.start(), match.end())
# We us group() method to get all the matches and
# captured groups. The groups contain the matched values.
# In particular:
# match.group(0) always returns the fully matched string
# match.group(1) match.group(2), ... return the capture
# groups in order from left to right in the input string
# match.group() is equivalent to match.group(0)
# So this will print "June 24"
print "Full match: %s" % (match.group(0))
# So this will print "June"
print "Month: %s" % (match.group(1))
# So this will print "24"
print "Day: %s" % (match.group(2))
else:
print "The regex pattern does not match."
python tutorial - Output :
Match at index 14, 21
Full match: June 24
Month: June
Day: 24
re.match() : This function attempts to match pattern to whole string. The re.match function returns a match object on success, None on failure.
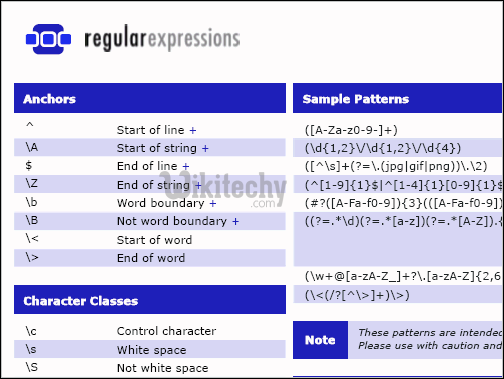
python - Sample - python code :
re.match(pattern, string, flags=0)
pattern : Regular expression to be matched.
string : String where p attern is searched
flags : We can specify different flags
using bitwise OR (|).
python - Sample - python code :
# A Python program to demonstrate working
# of re.match().
import re
# a sample function that uses regular expressions
# to find month and day of a date.
def findMonthAndDate(string):
regex = r"([a-zA-Z]+) (\d+)"
match = re.match(regex, string)
if match == None:
print "Not a valid date"
return
print "Given Data: %s" % (match.group())
print "Month: %s" % (match.group(1))
print "Day: %s" % (match.group(2))
# Driver Code
findMonthAndDate("Jun 24")
print("")
findMonthAndDate("I was born on June 24")

re.findall() : Return all non-overlapping matches of pattern in string, as a list of strings. The string is scanned left-to-right, and matches are returned in the order found (Source : Python Docs).
python - Sample - python code :
# A Python program to demonstrate working of
# findall()
import re
# A sample text string where regular expression
# is searched.
string = """Hello my Number is 123456789 and
my friend's number is 987654321"""
# A sample regular expression to find digits.
regex = '\d+'
match = re.findall(regex, string)
print(match)
python tutorial - Output :
['123456789', '987654321']
Regular expression is a vast topic. It’s a complete library. Regular expressions can do a lot of stuff. You can Match, Search, Replace, Extract a lot of data. For example, below small code is so powerful that it can extract email address from a text. So we can make our own Web Crawlers and scrappers in python with easy.Look at below regex.
python - Sample - python code :
# extract all email addresses and add them into the resulting set
new_emails = set(re.findall(r"[a-z0-9\.\-+_]+@[a-z0-9\.\-+_]+\.[a-z]+",
text, re.I))