python tutorial - Python Program to find Area of a Right Angled Triangle - learn python - python programming
- To write Python Program to find Area of a Right Angled Triangle with example.
- Before we step into the program, Let see the definition and formula behind Area of a Right Angled Triangle
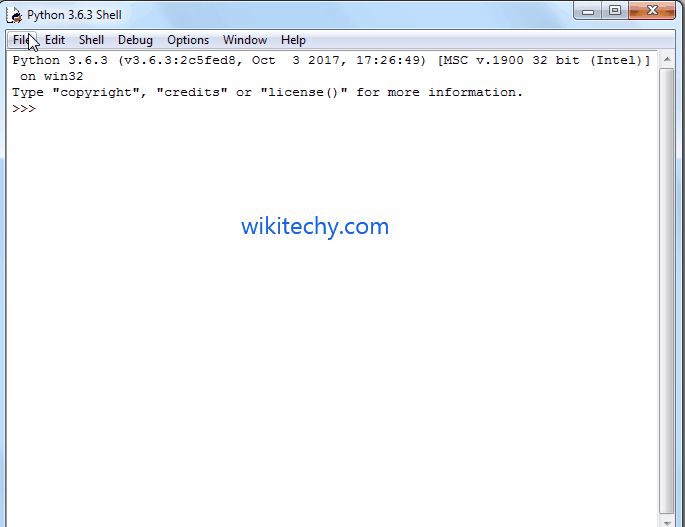
Learn Python - Python tutorial - Python Program to find Area of a Right Angled Triangle - Python examples - Python programs
Area of a Right Angled Triangle
- If we know the width and height then, we can calculate the area of a right angled triangle using below formula.
Area = (1/2) * width * height
click below button to copy the code. By Python tutorial team
- Using Pythagoras formula we can easily find the unknown sides in the right angled triangle.
c² = a² + b²
click below button to copy the code. By Python tutorial team
- Perimeter is the distance around the edges. We can calculate perimeter using below formula
Perimeter = a + b+ c
click below button to copy the code. By Python tutorial team
Python Program to find Area of a Right Angled Triangle
- This program allows the user to enter width and height of the right angled triangle.
- Using those values we will calculate the Area and perimeter of the right angled triangle.
Sample Code
# Python Program to find Area of a Right Angled Triangle
import math
width = float(input('Please Enter the Width of a Right Angled Triangle: '))
height = float(input('Please Enter the Height of a Right Angled Triangle: '))
# calculate the area
Area = 0.5 * width * height
# calculate the Third Side
c = math.sqrt((width*width) + (height*height))
# calculate the Perimeter
Perimeter = width + height + c
print("\n Area of a right angled triangle is: %.2f" %Area)
print(" Other side of right angled triangle is: %.2f" %c)
print(" Perimeter of right angled triangle is: %.2f" %Perimeter)
click below button to copy the code. By Python tutorial team
Output
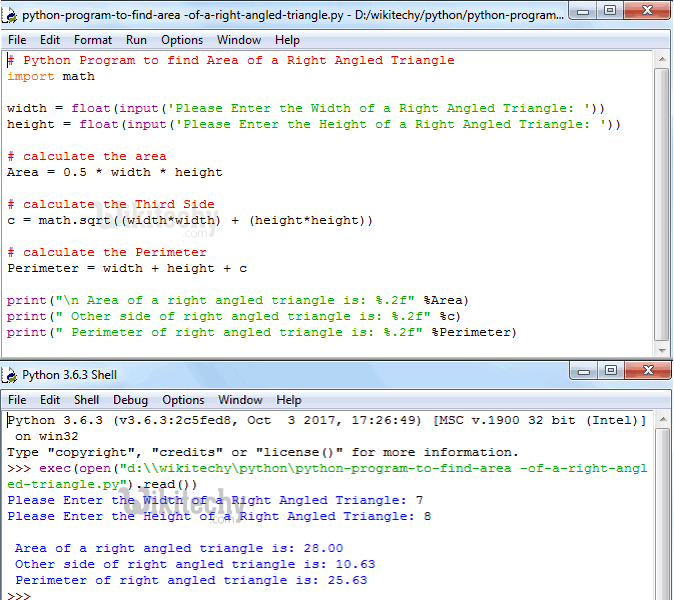
Learn Python - Python tutorial - Python Program to find Area of a Right Angled Triangle - Python examples - Python programs
Analysis
- First, We imported the math library using the following statement. This will allow us to use the mathematical functions like math.sqrt function
import math
click below button to copy the code. By Python tutorial team
- Following statements will allow the User to enter the Width and Height of a right angled triangle.
width = float(input('Please Enter the Width of a Right Angled Triangle: '))
height = float(input('Please Enter the Height of a Right Angled Triangle: '))
click below button to copy the code. By Python tutorial team
- Next, we are calculating the area (The value of 1/2 = 0.5). So we used 0.5 * width*height as the formula
Area = 0.5 * width * height
click below button to copy the code. By Python tutorial team
- In the next line, We are calculating the other side of a right-angled triangle using the Pythagoras formula C²=a²+b² , Which is similar to C = √a²+b²
c = math.sqrt((width*width) + (height*height))
click below button to copy the code. By Python tutorial team
- Here we used sqrt() function to calculate the square root of the a²+b². sqrt() is the math function, which is used to calculate the square root.
- In the next line, We are calculating the perimeter using the formula
Perimeter = width + height + c
click below button to copy the code. By Python tutorial team
- Following print statements will help us to print the Perimeter, Other side and Area of a right angled Triangle
print("\n Area of a right angled triangle is: %.2f" %Area)
print(" Other side of right angled triangle is: %.2f" %c)
print(" Perimeter of right angled triangle is: %.2f" %Perimeter)
click below button to copy the code. By Python tutorial team
Python Program to find Area of a Right Angled Triangle using functions
- This program allows the user to enter the width and height of a right angled triangle.
- We will pass those values to the function arguments to calculate the area of a right angled triangle.
Sample Code
# Python Program to find Area of a Right Angled Triangle using Functions
import math
def Area_of_a_Right_Angled_Triangle(width, height):
# calculate the area
Area = 0.5 * width * height
# calculate the Third Side
c = math.sqrt((width * width) + (height * height))
# calculate the Perimeter
Perimeter = width + height + c
print("\n Area of a right angled triangle is: %.2f" %Area)
print(" Other side of right angled triangle is: %.2f" %c)
print(" Perimeter of right angled triangle is: %.2f" %Perimeter)
Area_of_a_Right_Angled_Triangle(9, 10)
click below button to copy the code. By Python tutorial team
Output

Learn Python - Python tutorial - Python Program to find Area of a Right Angled Triangle using functions - Python examples - Python programs
Analysis
- First, We defined the function with two arguments using def keyword.
- It means, User will enter the width and height of a right angled triangle.
- Next, We are Calculating the Area of a right angled triangle as we described in our first example.
NOTE: We can call the function with arguments in .py file directly or else we can call it from the python shell. Please don’t forget the function arguments