This article illustrates how to automate movements of mouse and keyboard using pyautogui module in python. This module is not preloaded with python. So to install it run the following command:
python - Sample - python code :
pip install pyautogui
Controlling mouse movements using pyautogui module
Python tracks and controls mouse using coordinate system of screen. Suppose the resolution of your screen is 1920X1080 , then your screen’s coordinate system looks like :
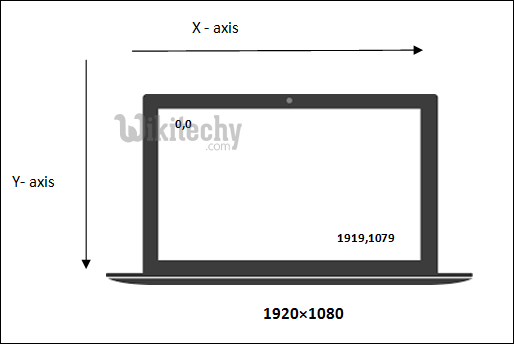
- size(): This function is used to get Screen resolution.
python - Sample - python code :
import pyautogui
print(pyautogui.size())
Save this file with .py extension, and then run the file.
This python code use size() function to output your screen resolution in x,y format:
python tutorial - Output :
(1920, 1080)
Note: Some of the codes provided in this article might not run on wikitechy IDE, since wikitechy IDE doesnot have required modules to run these codes. But these code can be easily run locally on your PC by installing python and following the instructions provided in the article.
- moveTo(): use this function move the mouse in pyautogui module.
python - Sample - python code :
import pyautogui
pyautogui.moveTo(100, 100, duration= 1)
This code uses moveTo() function, which takes x and y coordinates, and an optional duration argument. This function moves your mouse pointer from it’s current location to x,y coordinate, and takes time as specified by duration argument to do so. Save and run this python script to see your mouse pointer magically moving from its current location to coordinates (100, 100) , taking 1 second in this process.
- moveRel() function: moves the mouse pointer relative to its previous position.
python - Sample - python code :
import pyautogui
pyautogui.moveRel(0, 50, duration=1)
This code will move mouse pointer at (0, 50) relative to its original position. For example, if mouse position before running the code was (1000, 1000), then this code will move the pointer to coordinates (1000, 1050) in duration 1 second.
- position(): function to get current position of the mouse pointer.
python - Sample - python code :
import pyautogui
print(pyautogui.position())
python tutorial - Output :
- coordinates where your mouse was residing at the time of executing the program.
- click(): Function used for clicking and dragging the mouse.
python - Sample - python code :
import pyautogui
pyautogui.click(100, 100)
This code performs a typical mouse click at the location (100, 100).
We have two functions associated with drag operation of mouse, dragTo and dragRel. They perform similar to moveTo and moveRel functions, except they hold the left mouse button while moving, thus initiating a drag.
This functionality can be used at various places, like moving a dialog box, or drawing something automatically using pencil tool in MS Paint. To draw a square in paint:
python - Sample - python code :
import time
import time
#a module which has functions related to time.
# It can be installed using cmd command:
# pip install time, in the same way as pyautogui.
import pyautogui
time.sleep(10)
#makes program execution pause for 10 sec
pyautogui.moveTo(1000, 1000, duration=1)
#moves mouse to 1000, 1000.
pyautogui.dragRel(100, 0, duration=1)
#drags mouse 100, 0 relative to its previous position,
# thus dragging it to 1100, 1000
pyautogui.dragRel(0, 100, duration=1)
pyautogui.dragRel(-100, 0, duration=1)
pyautogui.dragRel(0, -100, duration=1)
Before running the code, open MS paint in background with pencil tool selected. Now run the code, quickly switch to MS paint before 10 seconds (since we have given 10 second pause time using sleep() function before running the program).
After 10 seconds, you will see a square being drawn in MS paint, with its top left edge at 1000,1000 and edge length 100 pixels.
- scroll(): scroll function takes no. of pixels as argument, and scrolls the screen up to given number of pixels.
python - Sample - python code :
import pyautogui
pyautogui.scroll(200)
This code scrolls the active screen up to 200 pixels.
- typewrite(): You can automate typing of string by using typewrite() function. just pass the string which you want to type as argument of this function.
python - Sample - python code :
import pyautogui
pyautogui.click(100, 100)
pyautogui.typewrite(“hello Wikitechy!”)
Suppose a text field was present at coordinates 100, 100 on screen, then this code will click the text field to make it active and type “hello Wikitechy!” in it.
- Passing key names: You can pass key names separately through typewrite() function.
python - Sample - python code :
import pyautogui
pyautogui.typewrite([“a”, “left”, “ctrlleft”])
This code is automatic equivalent of typing “a”, pressing left arrow key, and pressing left control key.
- Pressing hotkey combinations: Use hotkey() function to press combination of keys like ctrl-c, ctrl-a etc. Ex:
python - Sample - python code :
import pyautogui
pyautogui.hotkey(“ctrlleft”, “a”)
This code is automatic equivalent of pressing left ctrl and “a”simultaneously. Thus in windows, this will result in selection of all text present on screen.