python tutorial - Python interview programming questions - learn python - python programming
python interview questions :91
How to Parsing Brackets?
- Given a string consists of different types of brackets.
- For example, " ([])" and "[]{}" are balanced but "([)]" and "](){" are not.You can assume these are the only characters in the string: ()[]{}.
- If the strings are only consists of ( and ), it should not affect our solution. For example: " (())" or " (()("
- The key to the solution is to use "stack".
def isBalanced(test):
st = []
for b in test:
if b == '(':
st.append(b)
elif b == ')':
if st[-1] != '(':
return False
else:
st.pop()
if b == '{':
st.append(b)
elif b == '}':
if st[-1] != '{':
return False
else:
st.pop()
if b == '[':
st.append(b)
elif b == ']':
if st[-1] != '[':
return False
else:
st.pop()
# openings popped off yet, return False
if st:
return False
# passed the check, return True
return True
if __name__ == '__main__':
test = ['[](){}', '([)]' , '({[]})', '[()]{{}}', '[[())]]', '(({})[])', '()(()', '(()(']
bal = True
for t in test:
bal = isBalanced(t)
print("%s is balanced? %s" %(t, bal))
click below button to copy the code. By Python tutorial team
OUTPUT
[](){} is balanced? True
([)] is balanced? False
({[]}) is balanced? True
[()]{{}} is balanced? True
[[())]] is balanced? False
(({})[]) is balanced? True
()(() is balanced? False
(()( is balanced? False
python interview questions :92
How to Printing full path?
- Print all pull paths for the files under the directory where the running python script (path.py) is.
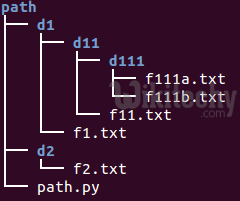
Learn python - python tutorial - python path - python examples - python programs
import os
script_location = os.path.dirname(os.path.abspath(__file__))
for dirpath, dirs, files in os.walk(script_location):
for f in files:
print(os.path.join(dirpath,f))
click below button to copy the code. By Python tutorial team
Output:
/home/k/TEST/PYTHON/path/path.py
/home/k/TEST/PYTHON/path/d1/f1.txt
/home/k/TEST/PYTHON/path/d1/d11/f11.txt
/home/k/TEST/PYTHON/path/d1/d11/d111/f111a.txt
/home/k/TEST/PYTHON/path/d1/d11/d111/f111b.txt
/home/k/TEST/PYTHON/path/d2/f2.txt
python interview questions :93
What is the index access method called?
- In Python [1:3] syntax on the second line .
test = (1,2,3,4,5)
print(test[1:3])
click below button to copy the code. By Python tutorial team
- The second parameter ("3") seems to be the index - 1 (3 - 1 = 2)
python interview questions :94
What is a qualified/unqualified name in Python?
- It is the path from top-level module down to the object itself.
- See PEP 3155, Qualified name for classes and functions.
- If you have a nested package named foo.bar.baz with a class Spam, the method ham on that class will have a fully qualified name of foo.bar.baz.Spam.ham. ham is the unqualified name.
- A qualified name lets you re-import the exact same object, provided it is not an object that is private to a local (function) namespace.
python interview questions :95
What is the difference between ''' and “”"?
- Use double quotes around strings that are used for interpolation
- single quotes for small symbol-like strings
- Use triple double quotes for docstrings and raw string literals for regular expressions .
Example:
LIGHT_MESSAGES = {
'English': "There are %(number_of_lights)s lights.",
'Pirate': "Arr! Thar be %(number_of_lights)s lights."
}
def lights_message(language, number_of_lights):
"""Return a language-appropriate string reporting the light count."""
return LIGHT_MESSAGES[language] % locals()
def is_pirate(message):
"""Return True if the given message sounds piratical."""
return re.search(r"(?i)(arr|avast|yohoho)!", message) is not None
click below button to copy the code. By Python tutorial team
python interview questions :96
What is the difference between json.load() and json.loads() functions in Python?
- load() loads JSON from a file or file-like object
- loads() loads JSON from a given string or unicode object
json.load(fp[, encoding[, cls[, object_hook[, parse_float[, parse_int[, parse_constant[, object_pairs_hook[, **kw]]]]]]]])
click below button to copy the code. By Python tutorial team
- Deserialize fp is to a Python object using this conversion table.
json.loads(s[, encoding[, cls[, object_hook[, parse_float[, parse_int[, parse_constant[, object_pairs_hook[, **kw]]]]]]]])
click below button to copy the code. By Python tutorial team
- Deserialize s (a str or unicode instance containing a JSON document) to a Python object using this conversion table.
python interview questions :97
Explain Redux syntax ?
- Create Store function from redux, takes reducer returned by combine Reducers and return a state accessible to the components defined in the Providers.
import allReducers from './YOUR_REDUCER_DIRECTORY'
var store = createStore(allReducers);
<Provider store = store>
<YOUR_COMPONENT i.e. HEADER/>
</Provider>
click below button to copy the code. By Python tutorial team
python interview questions :98
What are metaclasses? What do you use them for?
- A metaclass is the class of a class. Like a class defines how an instance of the class behaves, a metaclass defines how a class behaves. A class is an instance of a metaclass.
- While in Python you can use arbitrary callables for metaclasses (like Jerub shows), the more useful approach is actually to make it an actual class itself. The type is the usual metaclass in Python.
- In case you're wondering, yes, type is itself a class, and it is its own type. You won't be able to recreate something like type purely in Python, but Python cheats a little. To create your own metaclass in Python you really just want to subclass type.
- A metaclass is most commonly used as a class-factory. Like you create an instance of the class by calling the class, Python creates a new class (when it executes the 'class' statement) by calling the metaclass.
- Combined with the normal __init__ and __new__ methods, metaclasses therefore allow you to do 'extra things' when creating a class, like registering the new class with some registry, or even replace the class with something else entirely.
python interview questions :99
How to do Regular expression using findall?
- We can take only the words composed of digits from a given string using re.findall():
s = """Solar system:
Planets 8
Dwarf Planets: 5
Moons: Known = 149 | Provisional = 24 | Total = 173
Comets: More than 3400
Asteroids: More than 715000"""
import re
d = re.findall('\d+',s)
print d
click below button to copy the code. By Python tutorial team
Output:
['8', '5', '149', '24', '173', '3400', '715000']
python interview questions :100
How can we copy an object in Python?
- With new_list = old_list, we don't actually have two lists. The assignment just copies the reference to the list, not the actual list, so both new_list and old_list refer to the same list after the assignment.
Example:
>>> a = range(5)
>>> a
[0, 1, 2, 3, 4]
>>> b = a
>>> b
[0, 1, 2, 3, 4]
>>> id(a) == id(b)
True
>>> a.pop()
4
>>> b
[0, 1, 2, 3]
>>> a.remove(3)
>>> b
[0, 1, 2]
>>> del a[2]
>>> b
[0, 1]
click below button to copy the code. By Python tutorial team
- To copy the list, we can use slice:
>>> a = range(5)
>>> b = a[::]
>>> b
[0, 1, 2, 3, 4]
>>> id(a) == id(b)
False
>>> a.remove(4)
>>> b
[0, 1, 2, 3, 4]