Defining a Keyword
In programming, a keyword is a “reserved word” by the language which convey a special meaning to the interpreter. It may be a command or a parameter. Keywords cannot be used as a variable name in the program snippet.
Keywords in Python: Python language also reserves some of keywords that convey special meaning. Knowledge of these is necessary part of learning this language. Below is list of keywords registered by python .
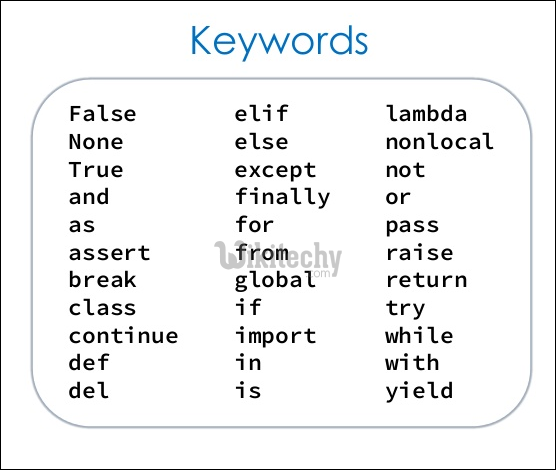
Python in its language defines an inbuilt module “keyword” which handles certain operations related to keywords. A function “iskeyword()” checks if a string is keyword or not. Returns true if a string is keyword, else returns false.
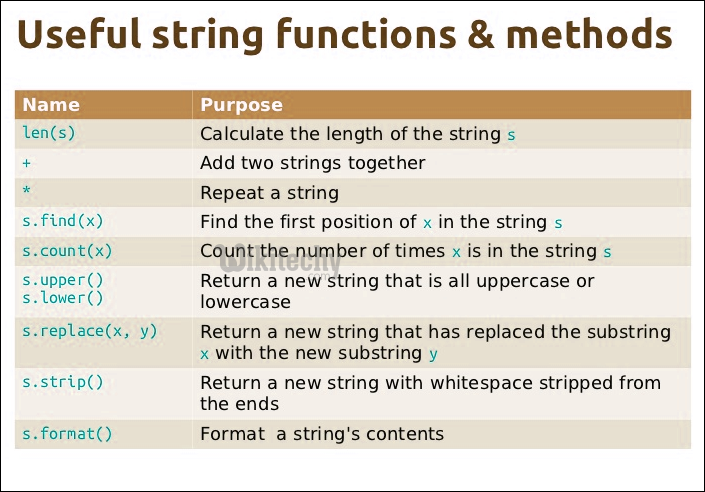
python - Sample - python code :
#Python code to demonstrate working of iskeyword()
# importing "keyword" for keyword operations
import keyword
# initializing strings for testing
s = "for"
s1 = "Wikitechy"
s2 = "elif"
s3 = "elseif"
s4 = "nikhil"
s5 = "assert"
s6 = "shambhavi"
s7 = "True"
s8 = "False"
s9 = "akshat"
s10 = "akash"
s11 = "break"
s12 = "ashty"
s13 = "lambda"
s14 = "suman"
s15 = "try"
s16 = "vaishnavi"
# checking which are keywords
if keyword.iskeyword(s):
print ( s + " is a python keyword")
else : print ( s + " is not a python keyword")
if keyword.iskeyword(s1):
print ( s1 + " is a python keyword")
else : print ( s1 + " is not a python keyword")
if keyword.iskeyword(s2):
print ( s2 + " is a python keyword")
else : print ( s2 + " is not a python keyword")
if keyword.iskeyword(s3):
print ( s3 + " is a python keyword")
else : print ( s3 + " is not a python keyword")
if keyword.iskeyword(s4):
print ( s4 + " is a python keyword")
else : print ( s4 + " is not a python keyword")
if keyword.iskeyword(s5):
print ( s5 + " is a python keyword")
else : print ( s5 + " is not a python keyword")
if keyword.iskeyword(s6):
print ( s6 + " is a python keyword")
else : print ( s6 + " is not a python keyword")
if keyword.iskeyword(s7):
print ( s7 + " is a python keyword")
else : print ( s7 + " is not a python keyword")
if keyword.iskeyword(s8):
print ( s8 + " is a python keyword")
else : print ( s8 + " is not a python keyword")
if keyword.iskeyword(s9):
print ( s9 + " is a python keyword")
else : print ( s9 + " is not a python keyword")
if keyword.iskeyword(s10):
print ( s10 + " is a python keyword")
else : print ( s10 + " is not a python keyword")
if keyword.iskeyword(s11):
print ( s11 + " is a python keyword")
else : print ( s11 + " is not a python keyword")
if keyword.iskeyword(s12):
print ( s12 + " is a python keyword")
else : print ( s12 + " is not a python keyword")
if keyword.iskeyword(s13):
print ( s13 + " is a python keyword")
else : print ( s13 + " is not a python keyword")
if keyword.iskeyword(s14):
print ( s14 + " is a python keyword")
else : print ( s14 + " is not a python keyword")
if keyword.iskeyword(s15):
print ( s15 + " is a python keyword")
else : print ( s15 + " is not a python keyword")
if keyword.iskeyword(s16):
print ( s16 + " is a python keyword")
else : print ( s16 + " is not a python keyword")
click below button to copy the code. By Python tutorial team
python tutorial - Output :
for is a python keyword
wikitechy is not a python keyword
elif is a python keyword
elseif is not a python keyword
nikhil is not a python keyword
assert is a python keyword
shambhavi is not a python keyword
True is a python keyword
False is a python keyword
akshat is not a python keyword
akash is not a python keyword
break is a python keyword
ashty is not a python keyword
lambda is a python keyword
suman is not a python keyword
try is a python keyword
vaishnavi is not a python keyword
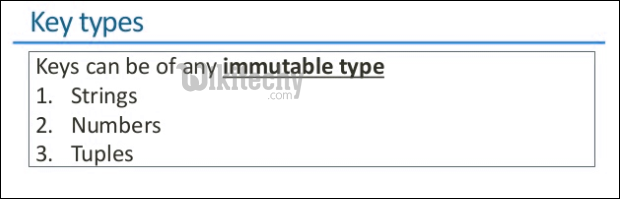
How to print list of all keywords
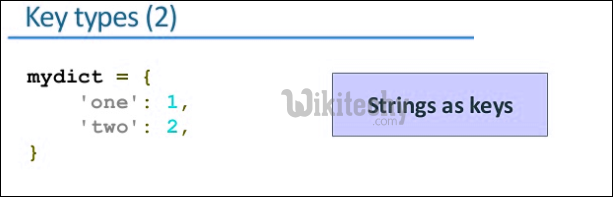
Sometimes, remembering all the keywords can be a difficult task while assigning variable names. Hence a function “kwlist()” is provided in “keyword” module which prints all the 33 python keywords.
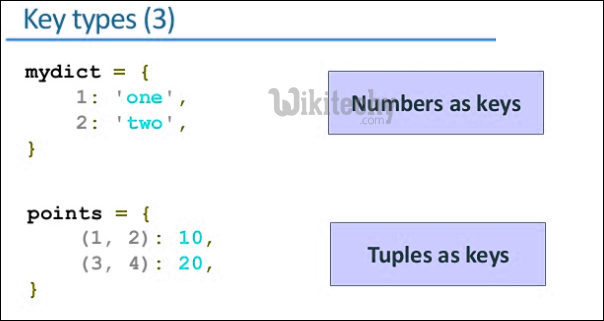
python - Sample - python code :
#Python code to demonstrate working of iskeyword()
# importing "keyword" for keyword operations
import keyword
# printing all keywords at once using "kwlist()"
print ("The list of keywords is : ")
print (keyword.kwlist)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
The list of keywords is :
['False', 'None', 'True', 'and', 'as', 'assert', 'break', 'class',
'continue', 'def', 'del', 'elif', 'else', 'except', 'finally',
'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda',
'nonlocal', 'not', 'or', 'pass', 'raise', 'return',
'try', 'while', 'with', 'yield']