python tutorial - Python scripting interview questions and answers for freshers - learn python - python programming
python interview questions :231
What is the most efficent way to implement concurrency in Python?
- I would say Google app engine is the easiest option since you don't have to worry about all this things. But if it's a big project, it might not be the cheapest solution.
- Again, it really depends on the size of your project and what you really want to do.
- You need to give more details if you want to get a precise answer.
- There is also a few packages available on the python package index.

Learn python - python tutorial - implement-concurrency-in-python - python examples - python programs
python interview questions :232
obj.count = 8 or setattr(obj, 'count', 8) ?
- They indeed do the same thing, but you cannot use a variable with the attribute setting syntax, while you can do that with setattr().
- In other words, this works:
foo = 'count'
setattr(x, foo, 2)
click below button to copy the code. By Python tutorial team
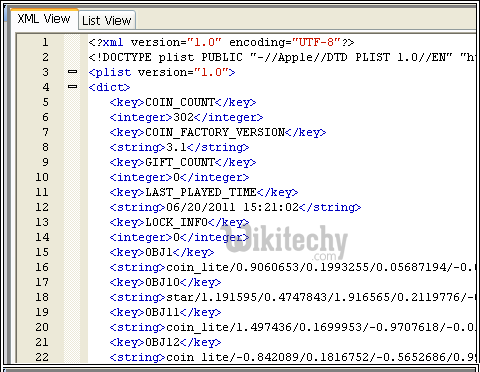
Learn python - python tutorial - python-list-view - python examples - python programs
- but this doesn't:
foo = 'count'
x.foo = 2
click below button to copy the code. By Python tutorial team
- Use setattr() for dynamic attribute setting, where the name of the attribute is taken from a variable. Use object.attributename = value for static attributes, where you know the name beforehand.
- In addition, the attribute access syntax is limited to proper Python identifiers, while setattr() can use any string. This means that you can use:
setattr(x, '3 little piggies', 42)
click below button to copy the code. By Python tutorial team
- where attempting that with static attributes will not work because Python identifiers do not allow for spaces, and cannot start with digits.
python interview questions :233
Name few methods for matching and searching the occurrences of a pattern in a given text String ?
There are 4 different methods in “re” module to perform pattern matching. They are:
- match() - matches the pattern only to the beginning of the String.
- search() - scan the string and look for a location the pattern
- matches findall() - finds all the occurrences of match and return them as a list
- finditer() - finds all the occurrences of match and return them as an iterator.
python interview questions :234
Explain split(), sub(), subn() methods ?
To modify the strings, Python’s “re” module is providing 3 methods. They are:
- split() - uses a regex pattern to “split” a given string into a list.
- sub() - finds all substrings where the regex pattern matches and then replace them with a different string
- subn() - it is similar to sub() and also returns the new string along with the no. of
- replacements.
python interview questions :235
How to display the contents of text file in reverse order?
Convert the given file into a list. reverse the list by using reversed()
for line in reversed(list(open(“file-name”,”r”))):
print(line)
click below button to copy the code. By Python tutorial team
python interview questions :236
What is JSON? How would convert JSON data into Python data?
JSON - stands for JavaScript Object Notation. It is a popular data format for storing data in NoSQL databases. Generally JSON is built on 2 structures.
- A collection of <name, value> pairs.
- An ordered list of values.
As Python supports JSON parsers, JSON-based data is actually represented as a dictionary in Python. You can convert json data into python using load() of json module.
python interview questions :237
Name few Python modules for Statistical, Numerical and scientific computations?
- numPy - this module provides an array/matrix type, and it is useful for doing computations on arrays.
- scipy - this module provides methods for doing numeric integrals, solving differential equations, etc
- pylab - is a module for generating and saving plots
- matplotlib - used for managing data and generating plots.
python interview questions :238
What is TkInter?
- TkInter is Python library. It is a toolkit for GUI development. It provides support for various GUI tools or widgets (such as buttons, labels, text boxes, radio buttons, etc) that are used in GUI applications.
- The common attributes of them include Dimensions, Colors, Fonts, Cursors, etc.
python interview questions :239
Name and explain the three magic methods of Python that are used in the construction and initialization of custom Objects ?
The 3 magic methods of Python that are used in the construction and initialization of custom Objects are:
- new - this method can be considered as a “constructor”. It is invoked to create an instance of a class with the statement say, myObj = MyClass()
- init__ - It is an “initializer”/ “constructor” method. It is invoked whenever any arguments are passed at the time of creating an object. myObj = MyClass(‘Pizza’,25)
- del- this method is a “destructor” of the class. Whenever an object is deleted,invocation of del__ takes place and it defines behaviour during the garbage collection.
Note: new , del are rarely used explicitly.
python interview questions :240
Is Python object oriented? what is object oriented programming?
Yes. Python is Object Oriented Programming language. OOP is the programming paradigm based on classes and instances of those classes called objects. The features of OOP are:
- Encapsulation,
- Data Abstraction,
- Inheritance,
- Polymorphism.