python tutorial - Python interview questions google - learn python - python programming
python interview questions :161
Sort Dictionary by Value using only .Sort() ?
- So many things we take for granted in newer versions.
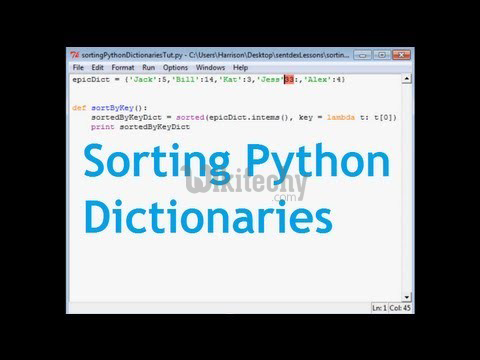
Learn python - python tutorial - sort-dictionary - python examples - python programs
- Anyways, Schwartzian Transform:
>>> l = [(-x[1], x) for x in d.items()]
>>> l.sort()
>>> l2 = [x[1] for x in l]
>>> l2
[('c', 3), ('a', 2), ('b', 1)]
click below button to copy the code. By Python tutorial team
python interview questions :162
What is the canonical way to create network packets in Objective C?
- Using a C struct is the normal approach. For example:
typedef struct {
int a;
char foo[2];
float b;
} MyPacket;
click below button to copy the code. By Python tutorial team
- Would define a type for an int, 2 characters and a float. You can then interpret those bytes as a byte array for writing:
MyPacket p = {.a = 2, .b = 2.7};
p.foo[0] = 'a';
p.foo[1] = 'b';
char *toWrite = (char *)&p; // a buffer of size sizeof(p)
click below button to copy the code. By Python tutorial team
python interview questions :163
Find the 2nd highest element ?
import heapq
res = heapq.nlargest(2, some_sequence)
print res[1] # to get 2nd largest
click below button to copy the code. By Python tutorial team
- If performance is a concern (e.g.: you intend to call this a lot), then you should absolutely keep the list sorted and de-duplicated at all times, and simply the first, second, or nth element (which is o(1)).
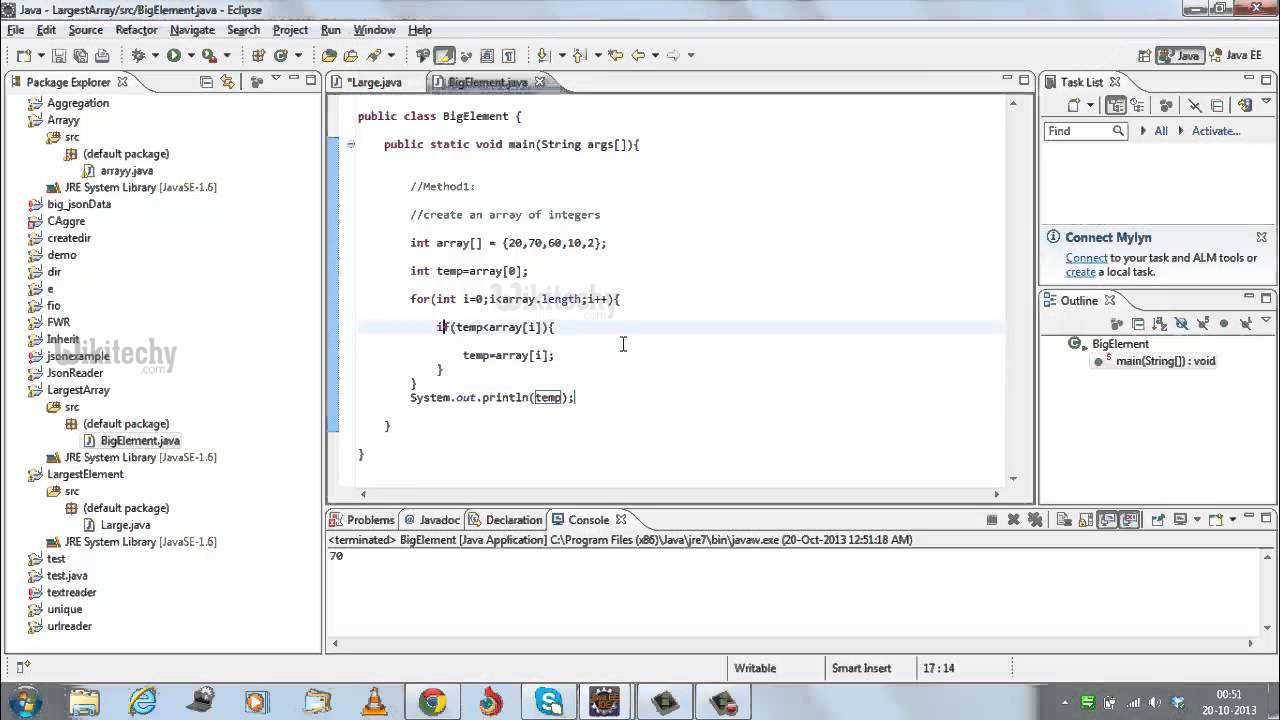
Learn python - python tutorial - max-default - python examples - python programs
- Use the bisect module for this - it's faster than a "standard" sort.
- insert lets you insert an element, and bisect will let you find whether you should be inserting at all (to avoid duplicates).
- If it's not, I'd suggest the simpler:
def nth_largest(li, n):.
return sorted(set(li))[-(n+1)]
click below button to copy the code. By Python tutorial team
- If the reverse indexing looks ugly to you, you can do:
def nth_largest(li, n):
return sorted(set(li), reverse=True)[n]
click below button to copy the code. By Python tutorial team
python interview questions :164
Extract text from HTML in python ?
- The question that monkut references doesn't give any Python solution to the exact problem.
- While BeautifulSoup and lxml both can be used to parse html, there is still a big step from there to text that approximates the formatting that is embedded in the html
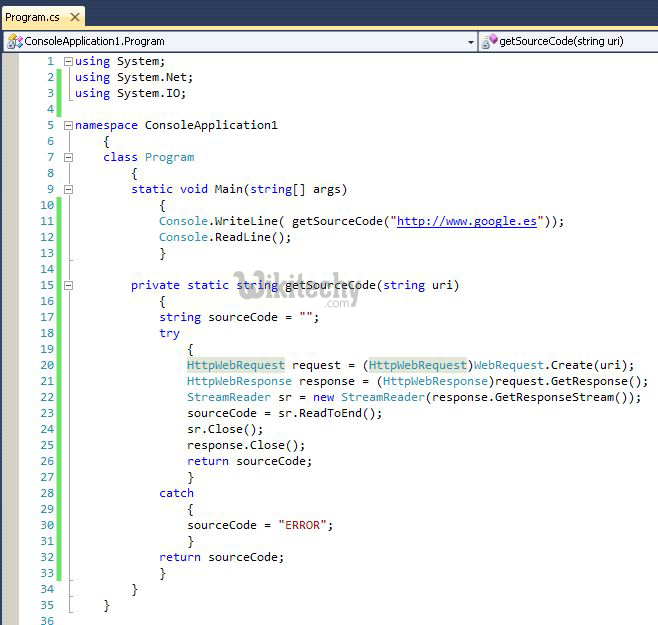
Learn python - python tutorial - source-code - python examples - python programs
- To do this, I have resorted to non-python solutions (which I've blogged about, but will resist linking here-- not sure of the SO etiquette).
- If you are on a *nix system, you can install this html2text package from Germany.
- It can be installed easily on a MacOS with Homebrew ($ brew install html2text) or Macports ($ sudo port install html2text), and on other *nix systems through their package managers.
It has a number of useful options, and I use it like this:
html2text -nobs -ascii -width 200 -style pretty -o filename.txt - < filename.html
click below button to copy the code. By Python tutorial team
- You can also install a text-based browser (e.g. w3m) and use it to produce formatted text from html using the following command-line syntax:
w3m filename.html -dump > file.txt
- You can, of course, access these solutions from Python using the subprocess module or the popular envoy wrapper for subprocess.
- Even after all this effort, you may find that some important information (e.g. <u> tags) are not handled in a way you like, but those are the best current options that I have found
python interview questions :165
C function with pointer of points wrapping in Python?
- After some code digging I figure it out:
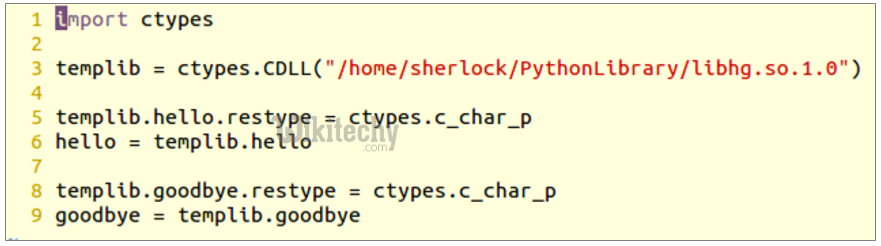
Learn python - python tutorial - c-function - python examples - python programs
- any C/C++ parameter that accepts a pointer to a list of values should be wrapped in python with
MyType=ctypes.ARRAY(/*any ctype*/,len)
MyList=MyType()
click below button to copy the code. By Python tutorial team
- and filled with
MyList[index]=/*that ctype*/
click below button to copy the code. By Python tutorial team
- in mycase the solution was:
from ctypes import *
path="test.dll"
lib = cdll.LoadLibrary(path)
Lines=["line 1","line 2"]
string_pointer= ARRAY(c_char_p,len(Lines))
c_Lines=string_pointer()
for i in range(len(Lines)):
c_Lines[i]=c_char_p(Lines[i].encode("utf-8"))
lib.SetLines(c_Lines,len(lines))
click below button to copy the code. By Python tutorial team
python interview questions :166
Python… Static variables?
- It is declared diffrent area. Ex1 is Like global or static variable
obj = MyClass()
obj2 = MyClass()
print "IS one instance ", id(obj.anArray) == id(obj2.anArray)
click below button to copy the code. By Python tutorial team
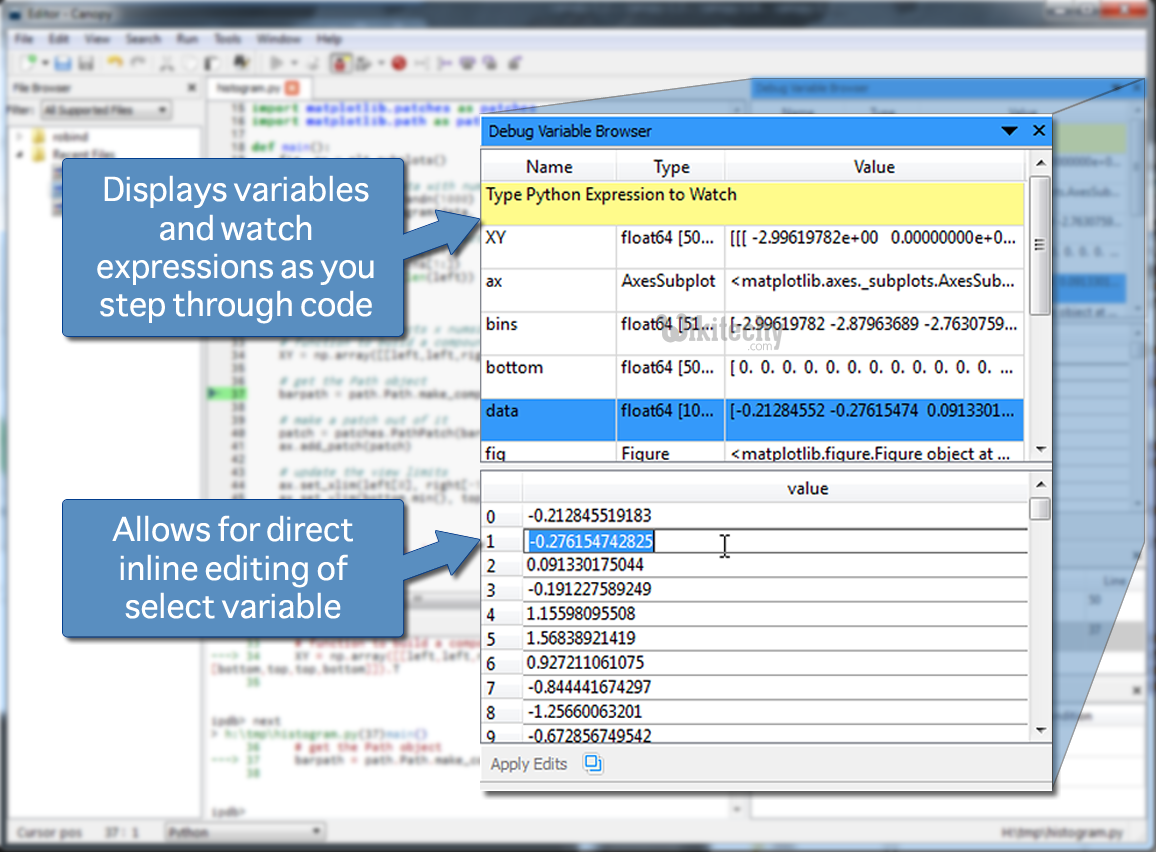
Learn python - python tutorial - python-debugger - python examples - python programs
- Ex2 is local attribute.
obj = MyClass()
obj2 = MyClass()
print "IS one instance ", id(obj.anArray) == id(obj2.anArray)
click below button to copy the code. By Python tutorial team
python interview questions :167
What is the equivalent of PHP “==”?
- The question says it.
- Here's a little explanation.
- In PHP. "==" works like this
2=="2" (Notice different type)
// True
click below button to copy the code. By Python tutorial team
- While in python:
2=="2"
// False
2==2
// True
click below button to copy the code. By Python tutorial team
- The equivalent for python "==" in php is "==="
2===2
//True
2==="2"
//False
click below button to copy the code. By Python tutorial team
python interview questions :168
How to find number of rows of a huge csv file in pandas?
- I need to get a line count of a large file (hundreds of thousands of lines) in python.
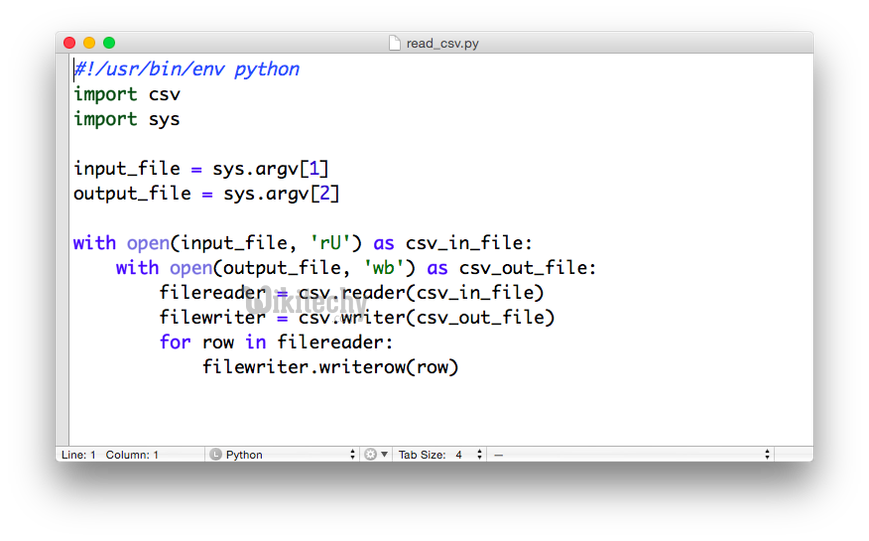
Learn python - python tutorial - read-csv-python - python examples - python programs
- At the moment I do:
def file_len(fname):
with open(fname) as f:
for i, l in enumerate(f):
pass
return i + 1
click below button to copy the code. By Python tutorial team
python interview questions :169
What is an InstrumentedList in Python?
- Yes, SQLAlchemy uses it to implement a list-like object which is aware of insertions and deletions of related objects to an object (via one-to-many and many-to-many relationships).
python interview questions :170
Scope of “library” methods ?
- I would recommend passing the locals() to the function, like so.
def file_len(fname):
with open(fname) as f:
for i, l in enumerate(f):
pass
return i + 1
click below button to copy the code. By Python tutorial team
- If you don't want that, you can use the inspect module or sys getframe to do the same thing, as other have suggested.