python tutorial - Python programming interview questions - learn python - python programming
python interview questions :181
Explain delegation in Python ?
- Delegation is an object oriented technique (also called a design pattern). Let's say you have an object x and want to change the behaviour of just one of its methods.
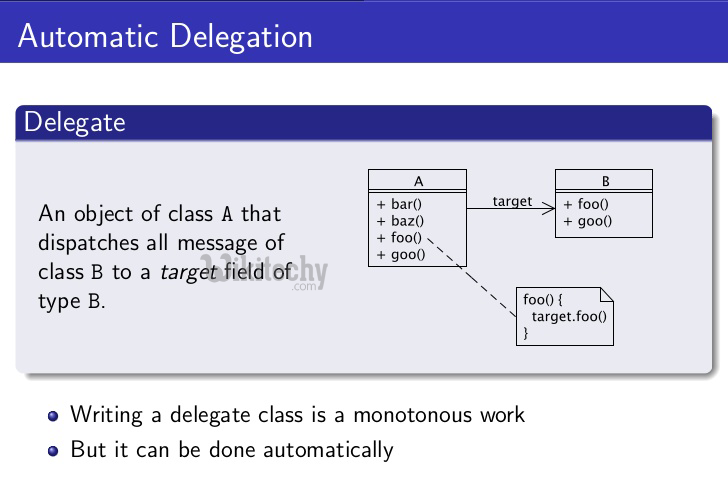
Learn python - python tutorial - metaclasses-python - python examples - python programs
- You can create a new class that provides a new implementation of the method you're interested in changing and delegates all other methods to the corresponding method of x.
- The example shows a class that captures the behavior of the file and converts data from lower to uppercase.
class upcase:
def __init__(self, out):
self._out = out
def write(self, s):
self._outfile.write(s.upper())
def __getattr__(self, name):
return getattr(self._out, name)
click below button to copy the code. By Python tutorial team
- The write() method that is used in the upcase class converts the string to the uppercase before calling another method. The delegation is being given using the self.__outfile object.
python interview questions :182
What is the function of “self”?
- Self” is a variable that represent the instance of the object to itself. In most of the object oriented programming language, this is passed as to the methods as a hidden parameters that is defined by an object. But, in python it is declare it and pass it explicitly.
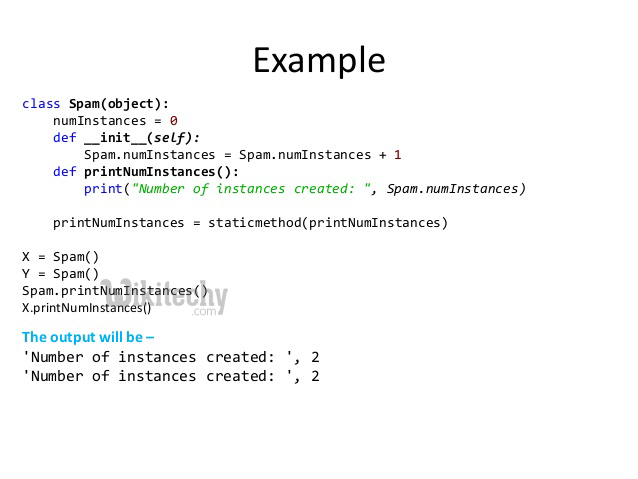
Learn python - python tutorial - advanced-python-static - python examples - python programs
- It is the first argument that gets created in the instance of the class A and the parameters to the methods are passed automatically. It refers to separate instance of the variable for individual objects.
- This is the first argument that is used in the class instance and the “self” method is defined explicitly to all the methods that are used and present. The variables are referred as “self.xxx”.
python interview questions :183
How is “self” explicitly defined in a method?
“Self” is a reference variable and an instance attribute that is used instead of the local variable inside the class.
- The function or the variable of the self like self.x or self.meth() can be used in case the class is not known. There are no variables declared as local.
- It doesn’t have any syntax and it allow the reference to be passed explicity or call the method for the class that is in use.
- The use of writebaseclass.methodname(self,
) shows that the method of _init_() can be extended to the base class methods. - This also solves the problem that is syntactic by using the assignment and the local variables.
- This tells a way to the interpreter the values that are to be used for the instance variables and local variables.
- The use of explicit self.var solves the problem mentioned above.
python interview questions :184
What is the use of join() for a string rather than list or tuple method?
- The functions and the methods that are used for the functionality uses the string module. This string module is represented as by using the join function in it:
", ".join(['1', '2', '4', '8', '16']) that results in "1, 2, 4, 8, 16"
click below button to copy the code. By Python tutorial team
- The string variable that is used provide a fixed string literal to allow the names that are used to be bounded to the strings. join() is a string method that is used to provide a separator string to use the function over the sequence of the string and insert the function to an adjacent elements.
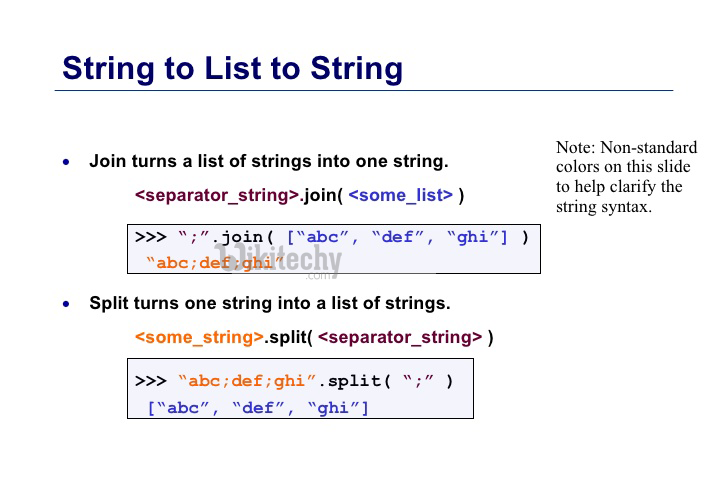
Learn python - python tutorial - list-string - python examples - python programs
- The method uses any number of arguments that follow some rules that has to be put up for the sequence objects that the class defines for itself.
- The join is used for the string module that is used to join the string characters together as it is given in the program. The example is given as:
string.join(['1', '2', '4', '8', '16'], ", ")
click below button to copy the code. By Python tutorial team
python interview questions :185
What is the process of compilation and linking in python?
- The compiling and linking allows the new extensions to be compiled properly without any error and the linking can be done only when it passes the compiled procedure
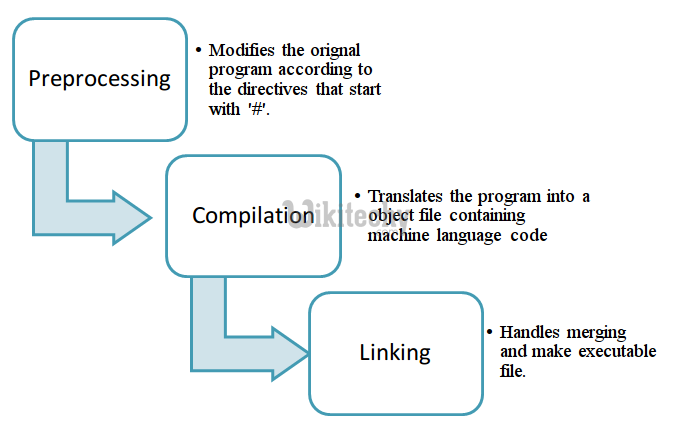
Learn python - python tutorial - link-process - python examples - python programs
- If the dynamic loading is used then it depends on the style that is being provided with the system.
- The python interpreter can be used to provide the dynamic loading of the configuration setup files and will rebuild the interpreter.
The steps that is required in this as: Create a file with any name and in any lanugage that is supported by the compiler of your system. For example comp.c
- Place this file in the Modules/ directory of the distribution which is getting used.
- Add a line in the file Setup.local that is present in the Modules/ directory.
- Run the file using spam comp.o
- After successful run of this rebuild the interpreter by using the make command on the top-level directory.
- If the file is changed then run rebuildMakefile by using the command as ‘make Makefile’.
python interview questions :186
What is the procedure to extract values from the object used in python?
- To extract the value it requires the object type to be defined and according to the object type only the values will be fetched.
The values will be extracted as:
- If the object is a tuple then PyTuple_Size() method is used that returns the length of the values and another method PyTuple_GetItem() returns the data item that is stored at a specific index.
- If the object is a list then PyListSize() is having the same function that is defined for the tuple and PyList_GetItem() that also return the data items at a specified index.
- Strings uses PyString_Size() to return the length of the value and PyString_AsString() that return the pointer to its value.
- To check the type of the object and the extracted values use of methods like PyString_Check(), PyTuple_Check(), PyList_Check(), etc are used.
python interview questions :187
What are the steps required to make a script executable on Unix?
The steps that are required to make a script executable are to:
- First create a script file and write the code that has to be executed in it.
- Make the file mode as executable by making the first line starts with #! this is the line that python interpreter reads.
- Set the permission for the file by using chmod +x file. The file uses the line that is the most important line to be used:
#!/usr/local/bin/python
click below button to copy the code. By Python tutorial team
- This explains the pathname that is given to the python interpreter and it is independent of the environment programs.
- Absolute pathname should be included so that the interpreter can interpret and execute the code accordingly. The sample code that is written:
#! /bin/sh
# Write your code here
exec python $0 ${1+"$@"}
click below button to copy the code. By Python tutorial team
- # Write the function that need to be included.
python interview questions :188
How does global value mutation used for thread-safety?
- The global interpreter lock is used to allow the running of the thread one at a time. This is internal to the program only and used to distribute the functionality along all the virtual machines that are used.
- Python allows the switching between the threads to be performed by using the byte code instructions that are used to provide platform-independence.
- The sys.setcheckinterval() method is used that allow the switching to occur during the implementation of the program and the instruction.
- This provides the understanding in the field of accounting to use the byte code implementation that makes it portable to use.
- The atomicity can be provided such that the shared variables can be given as built-in data types.
python interview questions :189
Write a program to read and write the binary data using python?
- The module that is used to write and read the binary data is known as struct. This module allows the functionality and with it many functionalities to be used that consists of the string class.
- This class contains the binary data that is in the form of numbers that gets converted in python objects for use and vice versa. The program can read or write the binary data is:
import struct
f = open(file-name, "rb")
# This Open() method allows the file to get opened in binary mode to make it portable for # use.
s = f.read(8)
x, y, z = struct.unpack(">hhl", s)
click below button to copy the code. By Python tutorial team
- The ‘>” is used to show the format string that allows the string to be converted in big-endian data form. For homogenous list of data the array module can be used that will allow the data to be kept more organized fashion.
python interview questions 190
What is the process to run sub-process with pipes that connect both input and output?
- The popen2() module is used to run the sub-process but due to some difficulty in processing like creation of deadlock that keep a process blocked that wait for the output from the child and child is waiting for the input.
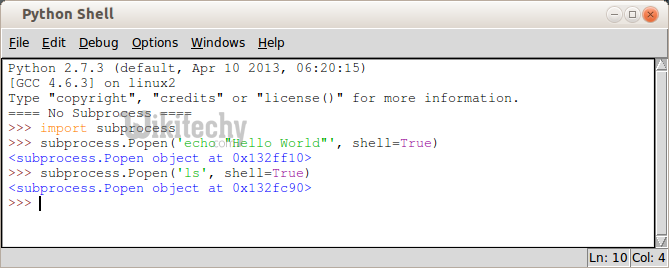
Learn python - python tutorial - python-shells - python examples - python programs
- The dead lock occurs due to the fact that parent and child doesn’t have the synchronization and both are waiting to get the processor to provide the resources to one another.
- Use of popen3() method allow the reading of stdout and stderr to take place where the internal buffer increases and there is no read() takes place to share the resources.
- popen2() take care of the deadlock by providing the methods like wait() and waitpid() that finishes a process first and when a request comes it hands over the responsibility to the process that is waiting for the resources.
- The program is used to show the process and run it.
import popen2
fromchild, tochild = popen2.popen2("command")
tochild.write("input\n")
tochild.flush()
output = fromchild.readline()