Python throws errors and exceptions, when there is a code gone wrong, which may cause program to stop abruptly. Python also provides exception handling method with the help of try-except. Some of the standard exceptions which are most frequent include IndexError, ImportError, IOError, ZeroDivisionError, TypeError and FileNotFoundError. A user can create his own error using exception class.
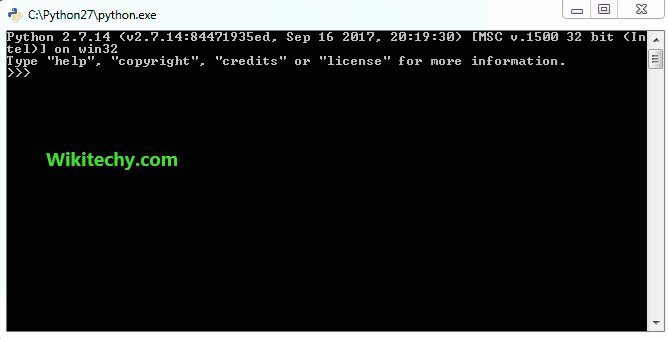
Learn Python - Python tutorial - python userexception - Python examples - Python programs
Creating User-defined Exception
Programmers may name their own exceptions by creating a new exception class. Exceptions need to be derived from the Exception class, either directly or indirectly. Although not mandatory, most of the exceptions are named as names that end in “Error” similar to naming of the standard exceptions in python. For example:
python - Sample - python code :
# A python program to create user-defined exception
# class MyError is derived from super class Exception
class MyError(Exception):
# Constructor or Initializer
def __init__(self, value):
self.value = value
# __str__ is to print() the value
def __str__(self):
return(repr(self.value))
try:
raise(MyError(3*2))
# Value of Exception is stored in error
except MyError as error:
print('A New Exception occured: ',error.value)
click below button to copy the code. By Python tutorial team
Output:
('A New Exception occured: ', 6)
Knowing all about Exception Class
To know more about about class Exception, run the code below
python - Sample - python code :
help(Exception)
Deriving Error from Super Class Exception
Super class Exceptions are created when a module needs to handle several distinct errors. One of the common way of doing this is to create a base class for exceptions defined by that module. Further, various subclasses are defined to create specific exception classes for different error conditions.
python - Sample - python code :
# class Error is derived from super class Exception
class Error(Exception):
# Error is derived class for Exception, but
# Base class for exceptions in this module
pass
class TransitionError(Error):
# Raised when an operation attempts a state
# transition that's not allowed.
def __init__(self, prev, nex, msg):
self.prev = prev
self.next = nex
# Error message thrown is saved in msg
self.msg = msg
try:
raise(TransitionError(2,3*2,"Not Allowed"))
# Value of Exception is stored in error
except TransitionError as error:
print('Exception occured: ',error.msg)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
('Exception occured: ', 'Not Allowed')
How to use standard Exceptions as base class?
Runtime error is a class is a standard exception which is raised when a generated error does not fall into any category. This program illustrates how to use runtime error as base class and network error as derived class. In a similar way, any exception can be derived from the standard exceptions of Python.
python - Sample - python code :
# NetworkError has base RuntimeError
# and not Exception
class Networkerror(RuntimeError):
def __init__(self, arg):
self.args = arg
try:
raise Networkerror("Error")
except Networkerror as e:
print (e.args)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
('E', 'r', 'r', 'o', 'r')