C# Access Modifiers - C# Modifiers
C# Access Modifiers
- In C# Access Modifiers or specifiers are the keywords that are used to specify accessibility or scope of variables and functions.
- In C# there are five types of access specifiers:
- Public
- Private
- Protected
- Internal
Public Access Specifier
- It makes data accessible publicly and it does not restrict data to the declared block.
- It specifies that access is not restricted.
- It is accessible for all classes.
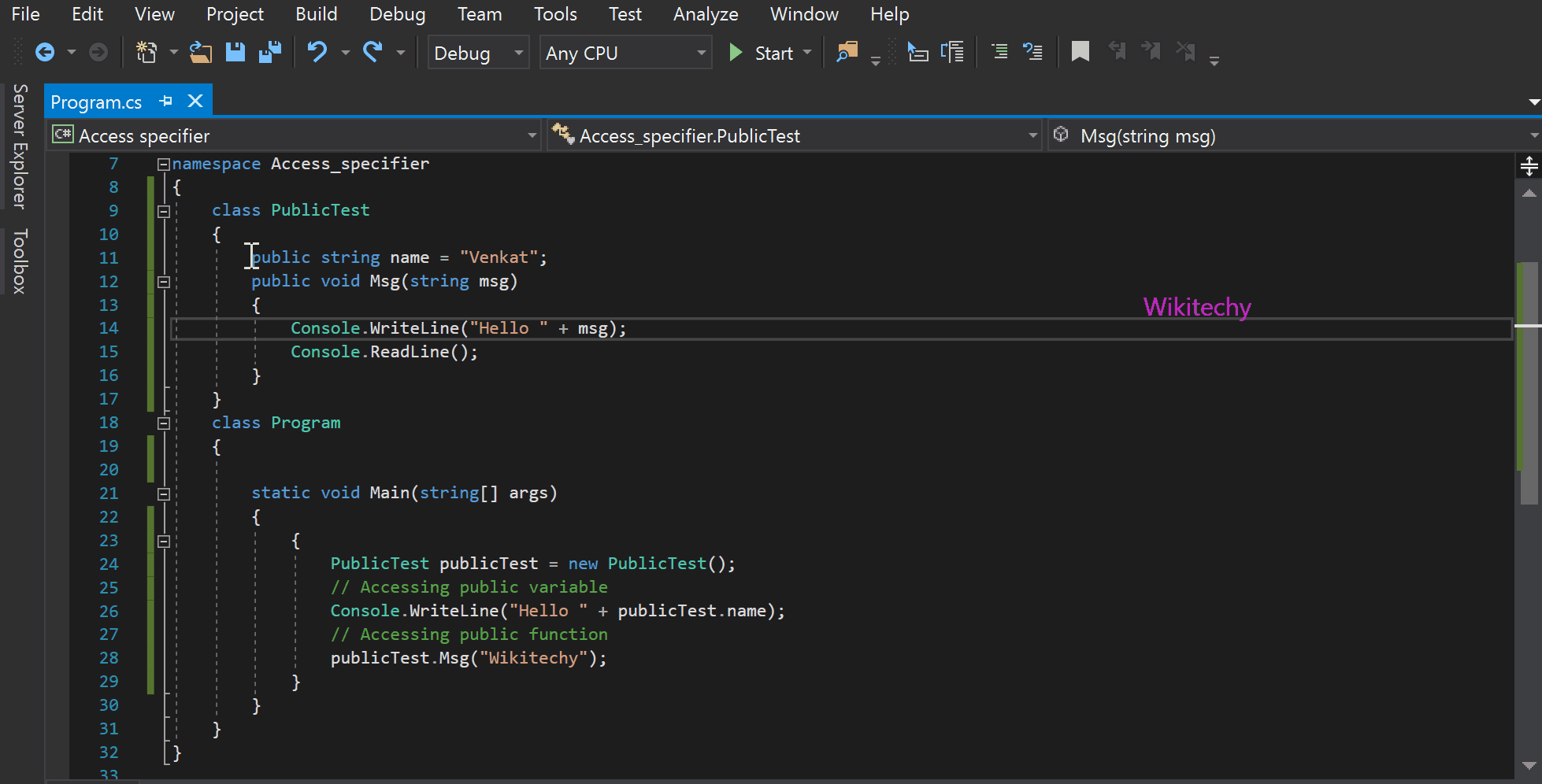
Sample Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Access_specifier
{
class PublicTest
{
public string name = "Venkat";
public void Msg(string msg)
{
Console.WriteLine("Hello " + msg);
Console.ReadLine();
}
}
class Program
{
static void Main(string[] args)
{
{
PublicTest publicTest = new PublicTest();
// Accessing public variable
Console.WriteLine("Hello " + publicTest.name);
// Accessing public function
publicTest.Msg("Wikitechy");
}
}
}
}
Output
Hello Venkat
Hello Wikitechy
Private Access Specifier
- Private Access Specifier is used to specify private accessibility to the function or variable.
- It is most accessible and restrictive only within the body of class in which it is declared.
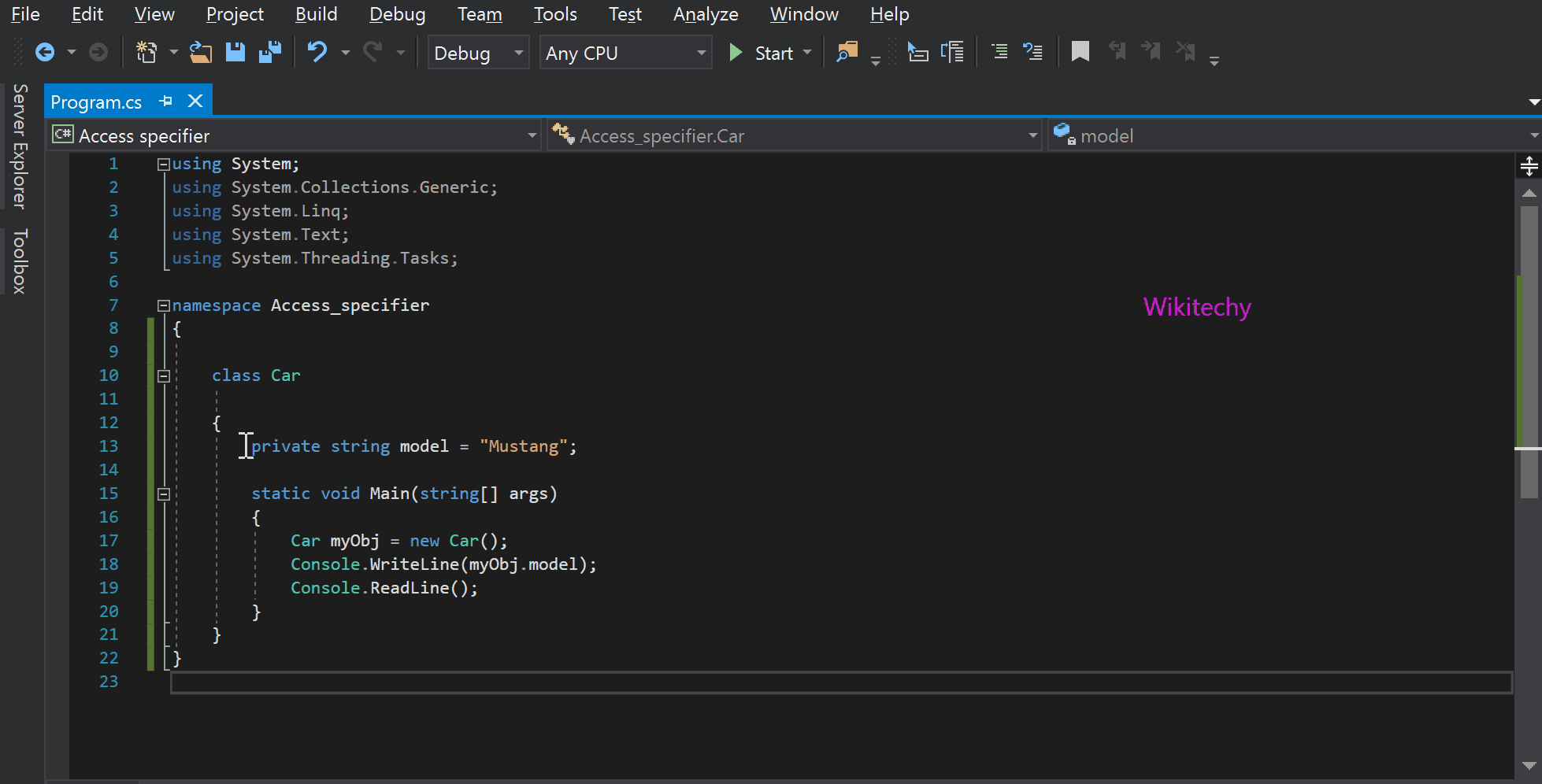
Sample Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Access_specifier
{
class Car
{
private string model = "Mustang";
static void Main(string[] args)
{
Car myObj = new Car();
Console.WriteLine(myObj.model);
Console.ReadLine();
}
}
}
Output
Mustang
Protected Access Specifier
- Protected is accessible within the class and has limited scope.
- In case of inheritance, it is also accessible within sub class or child class.
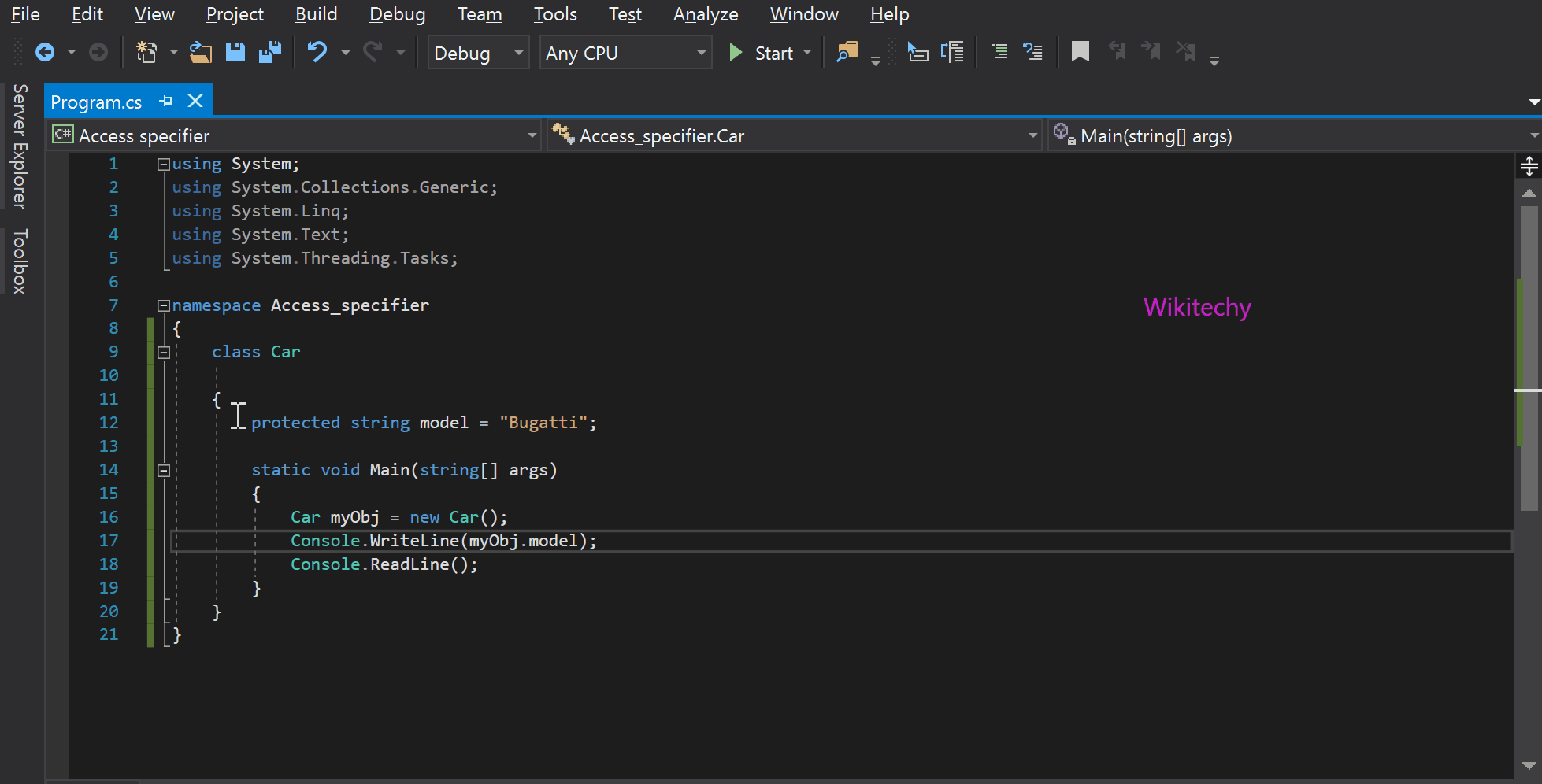
Sample Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Access_specifier
{
class Car
{
protected string model = "Bugatti";
static void Main(string[] args)
{
Car myObj = new Car();
Console.WriteLine(myObj.model);
Console.ReadLine();
}
}
}
Output
Bugatti
Internal Access Specifier
- Internal is used to specify the internal access specifier for the variables and functions.
- This access specifier is accessible only within files in the same assembly.
- It is only accessible within its own assembly, but not from another assembly.
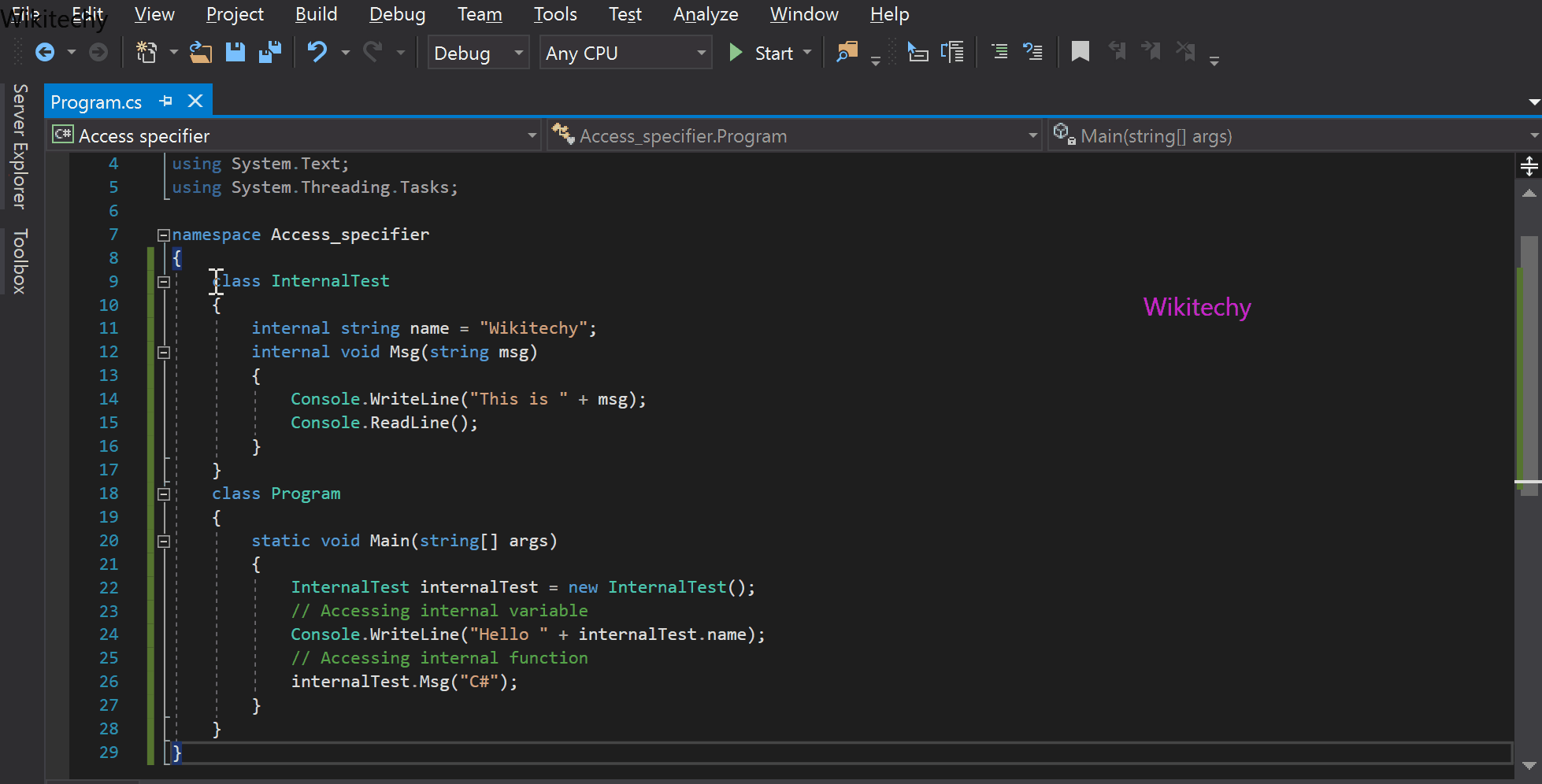
Sample Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Access_specifier
{
class InternalTest
{
internal string name = "Wikitechy";
internal void Msg(string msg)
{
Console.WriteLine("This is " + msg);
Console.ReadLine();
}
}
class Program
{
static void Main(string[] args)
{
InternalTest internalTest = new InternalTest();
// Accessing internal variable
Console.WriteLine("Hello " + internalTest.name);
// Accessing internal function
internalTest.Msg("C#");
}
}
}
Output
Hello Wikitechy
This is C#