C# Clone | C# String Clone - c# - c# tutorial - c# net
What is C# Clone ?
- In strings, Clone() method creates and returns a copy of string object.
- In C#, the string Clone() method returns a reference to this instance of string.
- The Clone() and ICloneable method interfaces are available in C programming. But using these features is unclear and not recommended.
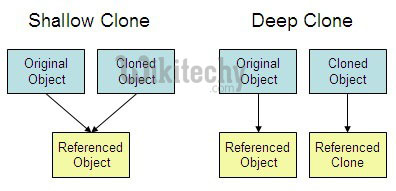
clone object in c#
- Clone() usually provides a shallow copy of an object (i.e. see Array.
- Clone() ), it copies the references but not the referenced objects. ... This is because they are reference types.
- Using Clone() (in a proper manner) can avoid this.
- On modifying a cloned object , the original will not get modified.
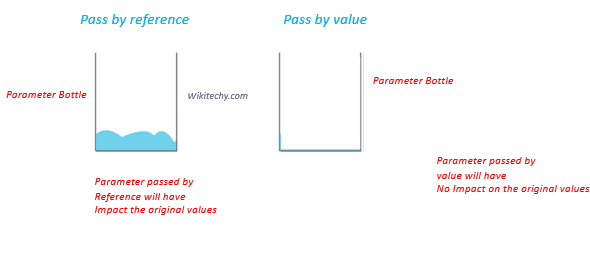
pass by reference vs pass by value in c#
Syntax:
Object
Syntax Explanation:
- Object: It returns the instance of the String.
- String: String returns a new String as the same content of argument String.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace wikitechy_string_clone
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
string str1 = null;
string str2 = null;
str1 = "www.wikitechy.com";
str2 = string.Copy(str1);
MessageBox.Show(str2);
}
}
}
Code Explanation:
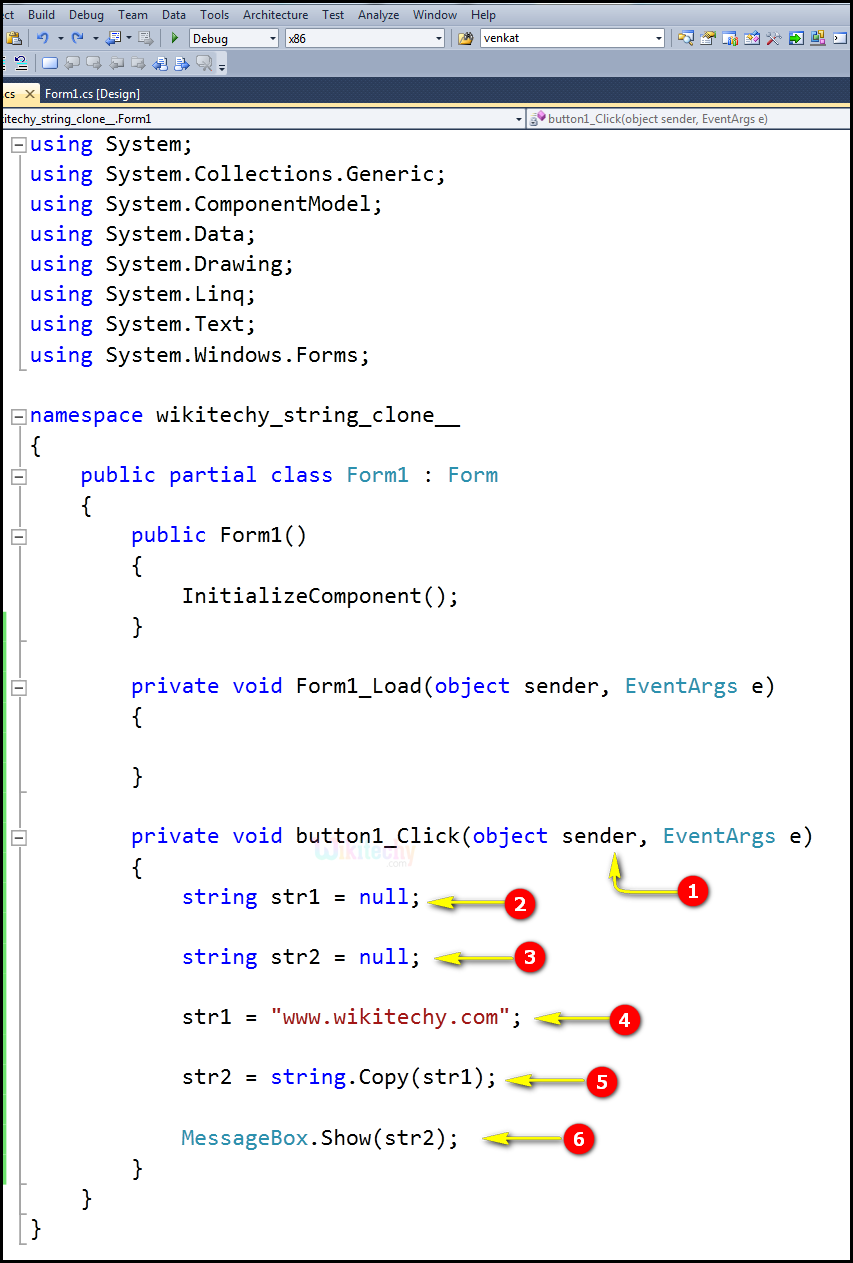
- Here private void button1_Click(object sender, EventArgs e) click event handler for button1 specifies, which is a normal Button control in Windows Forms.The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e).
- string str1 = null specifies to first Parameter String, its specifies the first null value.
- string str2 = null specifies to second Parameter String, its specifies the second null value.
- str1 is specifies to assigns text value of www.wikitechy.com.
- Copies contents www.wikitechy.com of str1 to an array of characters. we can also specify the starting character of a string and number of characters we want to copy to the str2.
- MessageBox.Show(str2); displays a message box with the specified string"str2" which presents a message to the user.
Sample C# examples - Output :
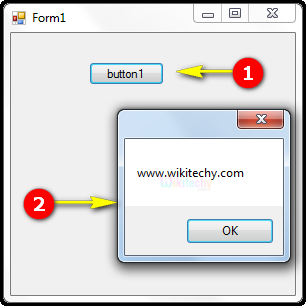
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- In this output table displays the www.wikitechy.comchecked in the message box.