C# TextWriter - c# - c# tutorial - c# net
What is TextWriter Class in C# ?
- The TextWriter class represents a writer that can write sequential series of characters.
- You can use this class to write text in a file. It is an abstract base class of StreamWriter and StringWriter, which write characters to streams and string respectively.
- It is used to write text or sequential series of characters into file.
- It is found in System.IO namespace.
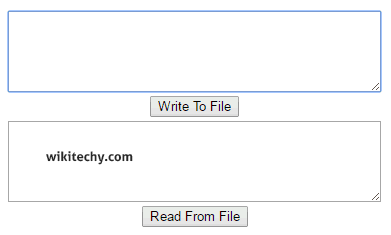
C# TextWriter
Constructors:
S.No | Name | Description |
---|---|---|
1 | TextWriter() | Initializes a new instance of the TextWriter class. |
2 | TextWriter(IFormatProvider) | Initializes a new instance of the TextWriter class with the specified format provider. |
Properties:
Name | Description |
---|---|
Encoding | When overridden in a derived class, returns the character encoding in which the output is written. |
FormatProvider | Gets an object that controls formatting. |
NewLine | Gets or sets the line terminator string used by the current TextWriter. |
Methods:
Name | Description |
---|---|
Close() | Closes the current writer and releases any system resources associated with the writer. |
CreateObjRef(Type) | Creates an object that contains all the relevant information required to generate a proxy used to communicate with a remote object.(Inherited from MarshalByRefObject.) |
Dispose() | Releases all resources used by the TextWriter object. |
Dispose(Boolean) | Releases the unmanaged resources used by the TextWriter and optionally releases the managed resources. |
Equals(Object) | Determines whether the specified object is equal to the current object.(Inherited from Object.) |
Finalize() | Allows an object to try to free resources and perform other cleanup operations before it is reclaimed by garbage collection.(Inherited from Object.) |
Flush() | Clears all buffers for the current writer and causes any buffered data to be written to the underlying device. |
FlushAsync() | Asynchronously clears all buffers for the current writer and causes any buffered data to be written to the underlying device. |
GetHashCode() | Serves as the default hash function. (Inherited from Object.) |
GetLifetimeService() | Retrieves the current lifetime service object that controls the lifetime policy for this instance.(Inherited from MarshalByRefObject.) |
GetType() | Gets the Type of the current instance.(Inherited from Object.) |
InitializeLifetimeService() | Obtains a lifetime service object to control the lifetime policy for this instance.(Inherited from MarshalByRefObject.) |
C# TextWriter Example
- Let's see the simple example of TextWriter class to write two lines data.
using System;
using System.IO;
namespace TextWriterExample
{
class Program
{
static void Main(string[] args)
{
using (TextWriter writer = File.CreateText("e:\\f.txt"))
{
writer.WriteLine("Hello C#");
writer.WriteLine("C# File Handling by wikitechy");
}
Console.WriteLine("Data written successfully...");
}
}
}
C# examples - Output :
Data written successfully...
f.txt:
Hello C#
C# File Handling by wikitechy