C# static constructor | C# static constructor - c# - c# tutorial - c# net
What is static constructor in C# ?
- C# static constructor is used to initialize static fields. It can also be used to perform any action that is to be performed only once.
- It is invoked automatically before first instance is created or any static member is referenced.
Points to remember for C# Static Constructor
- C# static constructor cannot have any modifier or parameter.
- C# static constructor is invoked implicitly. It can't be called explicitly.
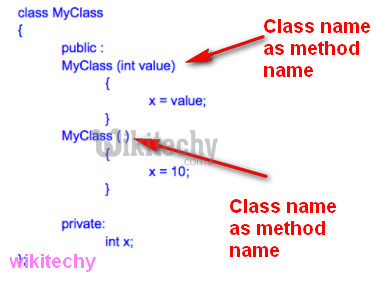
Constructor
C# Static Constructor example
- Let's see the example of static constructor which initializes the static field rateOfInterest in Account class.
using System;
public class Account
{
public int id;
public String name;
public static float rateOfInterest;
public Account(int id, String name)
{
this.id = id;
this.name = name;
}
static Account()
{
rateOfInterest = 9.5f;
}
public void display()
{
Console.WriteLine(id + " " + name+" "+rateOfInterest);
}
}
class TestEmployee{
public static void Main(string[] args)
{
Account a1 = new Account(101, "Sonoo");
Account a2 = new Account(102, "Mahesh");
a1.display();
a2.display();
}
}
C# examples - Output :
101 Sonoo 9.5
102 Mahesh 9.5