C# Sorted Dictionary - c# - c# tutorial - c# net
What is Sorted dictionaly in C# ?
- C# SortedDictionary<TKey, TValue> class uses the concept of hashtable.
- It stores values on the basis of key.
- It contains unique keys and maintains ascending order on the basis of key.
- By the help of key, we can easily search or remove elements.
- It is found in System.Collections.Generic namespace.
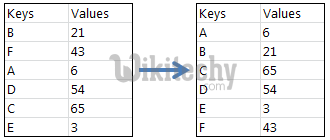
Sorted Dictionary
Sorted Dictionary Classes:
- SortedList
- SortedList<Key, Value>
- SortedDictionary <Key, Value>
Constructors:
Name | Description |
---|---|
SortedDictionary<TKey, TValue>() | Initializes a new instance of the SortedDictionary<TKey, TValue> class that is empty and uses the default IComparer<T> implementation for the key type. |
SortedDictionary<TKey, TValue>(IComparer<TKey>) | Initializes a new instance of the SortedDictionary<TKey, TValue> class that is empty and uses the specified IComparer<T> implementation to compare keys. |
SortedDictionary <TKey, TValue>(IDictionary<TKey, TValue>) |
Initializes a new instance of the SortedDictionary<TKey, TValue> class that contains elements copied from the specified IDictionary<TKey, TValue> and uses the default IComparer<T> implementation for the key type. |
SortedDictionary <TKey, TValue>(IDictionary<TKey, TValue>, IComparer<TKey>) |
Initializes a new instance of the SortedDictionary<TKey, TValue>
class that contains elements copied from the specified IDictionary<TKey, TValue> and uses the specified IComparer<T> implementation to compare keys. |
properties:
Namme | Description |
---|---|
Comparer | Gets the IComparer<T> used to order the elements of the SortedDictionary<TKey, TValue>. |
Count | Gets the number of key/value pairs contained in the SortedDictionary<TKey, TValue>. |
Item[TKey] | Gets or sets the value associated with the specified key. |
Keys | Gets a collection containing the keys in the SortedDictionary<TKey, TValue>. |
Values | Gets a collection containing the values in the SortedDictionary<TKey, TValue>. |
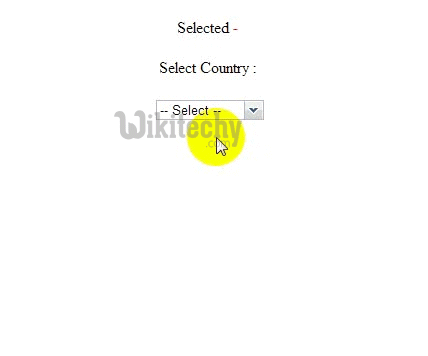
dictionary in csharp
Read Also
dotnet institute in chennai , summer internship with stipend , dot net training and placement institutesC# SortedDictionary<TKey, TValue> example:
- Let's see an example of generic SortedDictionary<TKey, TValue> class that stores elements using Add() method and iterates elements using for-each loop.
- Here, we are using KeyValuePair class to get key and value.
using System;
using System.Collections.Generic;
public class SortedDictionaryExample
{
public static void Main(string[] args)
{
SortedDictionary<string, string> names = new SortedDictionary<string,
string>();
names.Add("1","Sonoo");
names.Add("4","Peter");
names.Add("5","James");
names.Add("3","Ratan");
names.Add("2","Irfan");
foreach (KeyValuePair<string, string> kv in names)
{
Console.WriteLine(kv.Key+" "+kv.Value);
}
}
}
C# examples - Output :
1 Sonoo
2 Irfan
3 Ratan
4 Peter
5 James