C# Base - c# - c# tutorial - c# net
What is Base keyword in C# ?
- In C#, base keyword is used to access fields, constructors and methods of base class.
- You can use base keyword within instance method, constructor or instance property accessor only.
- You can't use it inside the static method.
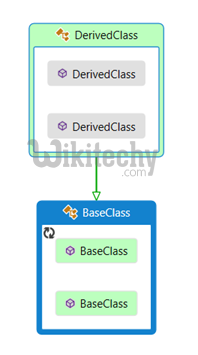
base class and dervied class in csharp
C# base keyword: accessing base class field
- We can use the base keyword to access the fields of the base class within derived class.
- It is useful if base and derived classes have the same fields.
- If derived class doesn't define same field, there is no need to use base keyword.
- Base class field can be directly accessed by the derived class.
- Let's see the simple example of base keyword in C# which accesses the field of base class.
using System;
public class Animal{
public string color = "white";
}
public class Dog: Animal
{
string color = "black";
public void showColor()
{
Console.WriteLine(base.color);
Console.WriteLine(color);
}
}
public class TestBase
{
public static void Main()
{
Dog d = new Dog();
d.showColor();
}
}
C# examples - Output :
white
black
C# base keyword example: calling base class method
- By the help of base keyword, we can call the base class method also.
- It is useful if base and derived classes defines same method. In other words, if method is overridden.
- If derived class doesn't define same method, there is no need to use base keyword. Base class method can be directly called by the derived class method.
- Let's see the simple example of base keyword which calls the method of base class.
using System;
public class Animal{
public virtual void eat(){
Console.WriteLine("eating...");
}
}
public class Dog: Animal
{
public override void eat()
{
base.eat();
Console.WriteLine("eating bread...");
}
}
public class TestBase
{
public static void Main()
{
Dog d = new Dog();
d.eat();
}
}
C# examples - Output :
eating...
eating bread...
C# inheritance: calling base class constructor internally
- Whenever you inherit the base class, base class constructor is internally invoked. Let's see the example of calling base constructor.
using System;
public class Animal{
public Animal(){
Console.WriteLine("animal...");
}
}
public class Dog: Animal
{
public Dog()
{
Console.WriteLine("dog...");
}
}
public class TestOverriding
{
public static void Main()
{
Dog d = new Dog();
}
}
C# examples - Output :
animal...
dog...