C# Operator Overloading - Operator Overloading in C#
C# Operator Overloading
- C# operator overloading is generally defined as a process of implementing popular Object-oriented programming concepts such as Polymorphism, which means one name having different forms and implementations.
- It allows the object or the variables to take different kinds of forms while executing the code.
- Operator overloading is mainly used when you want the method property not to be similar to the given arguments, rather want a different order of execution when one name can be used with different types of execution methods and properties.
- It can be achieved in a program with different types of parameters and their count.
Operator Overloading Techniques in C#
- In C#, operator overloading can be done using different forms of operators.
- But before proceeding to the techniques, let's consider the validations of operators and how are they used while performing operator overloading.
- The operator is usually is a keyword that is used while implementing operator overloading and it is important to note that the return type of the overloaded operator cannot be kept void.
- It means that while performing operator overloading, the preference is always in the over-defined implementations' hands.
- There is no preference given to predefined implementations and the overloaded methods should always have a different set of arguments.
- So, their order and number should not be the same.
- In the case of user-defined methods, the operator's precedence and syntax cannot be changed, but they are similar to any other method.
- The left one is called a member in binary operators, when the right is an object called a parameter.
- In C# there is a special function known as the operator function and this function or method must always be static and public.
- In operator functions the outer and the reference parameters are usually not allowed as arguments.
Syntax
public static return_type operator op (argument list)
- Here, the op is the operator used to overload, and the operator is the only needed keyword.
- In the case of a unary operator, we would pass only one argument and two-argument for the binary operator.
- It is also important to note that at least one argument should be user-defined or a struct type or a class.
Operators | Overloadability |
---|---|
+, -, *, %, &, |, <<, >> | All C# binary operators can be overloaded. |
+, -, !, ~, ++, --, true, false | All C# unary operators can be overloaded. |
==, !=, <, >, <=, >= | All relational operators can be overloaded, but only as pairs |
&&, | | | They can’t be overloaded. |
[] Array index operator | They can’t be overloaded. |
() Conversion Operator | They can’t be overloaded. |
+=, -=, *=, /=, %= | These compound assignment operators can be overloaded. But in C#, theses operators are automatically overloaded when the respective binary operator is overloaded. |
=, ., ?:, ->, new, is as, sizeof | These operators can’t be overloaded in C#. |
Syntax
public static classname operator op (parameters)
{
// Code
}
For Unary Operator
public static classname operator op (t)
{
// Code
}
For Binary Operator
public static classname operator op (t1, t2)
{
// Code
}
Types of operators overloading methods in C#
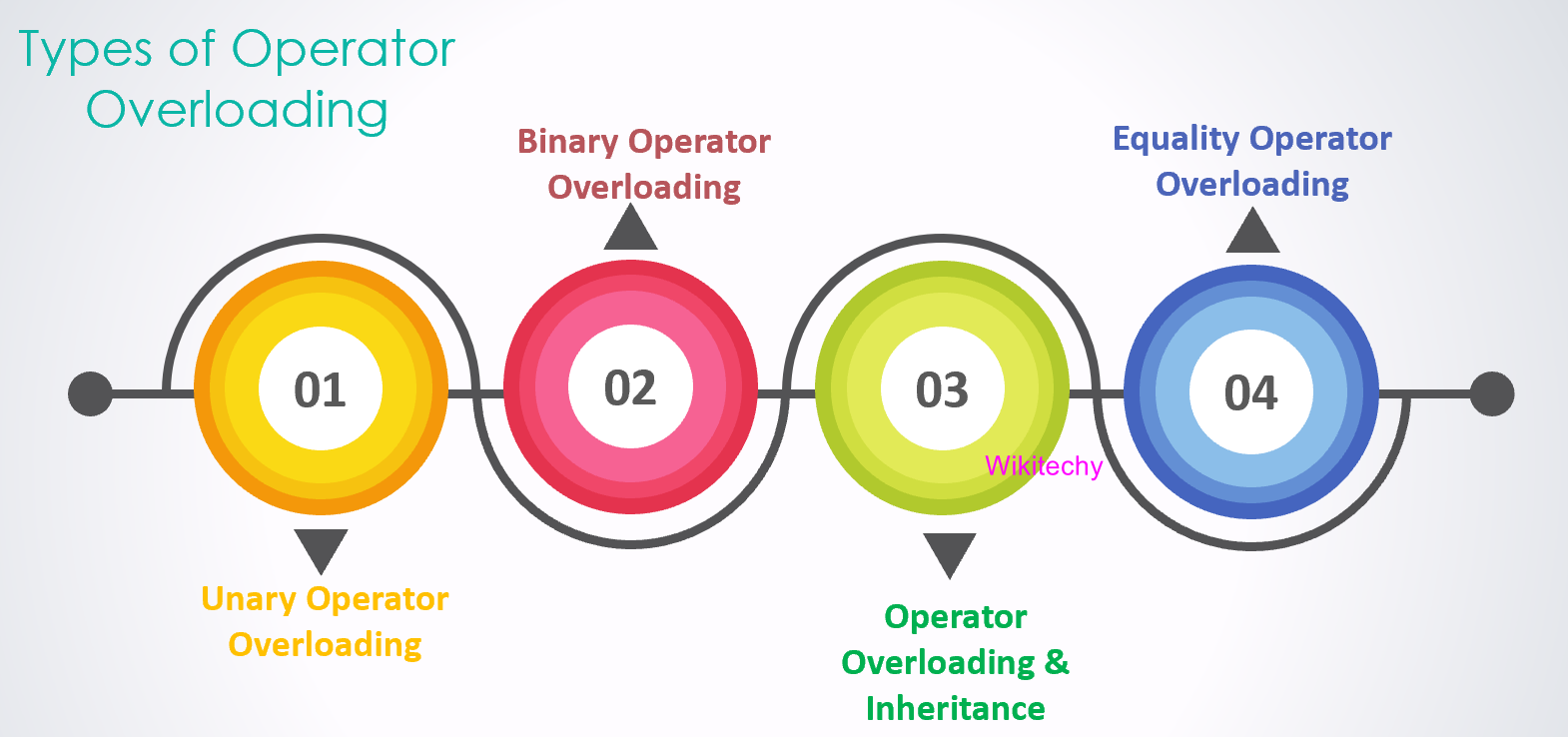
Unary Operator Overloading
- Here, the return type can be anything, but it should not be void for the operators like +, -, ~ and . (dot).
- The return type of this operator should be either a boolean or an integer and here important to note that the Boolean operators roll out true and false and hence can be overloaded only as pairs.
- A compilation error would occur if not done because a class generally declares this operator without declaring the other.
Syntax
public static return_type operator op (Type t) { // Statements }
Sample Code
using System;
class Complex
{
private int x;
private int y;
public Complex()
{
}
public Complex(int i, int j)
{
x = i;
y = j;
}
public void ShowXY()
{
Console.WriteLine("{0} {1}", x, y);
}
public static Complex operator -(Complex c)
{
Complex temp = new Complex();
temp.x = -c.x;
temp.y = -c.y;
return temp;
}
}
class MyClient
{
public static void Main()
{
Complex c1 = new Complex(10, 20);
c1.ShowXY(); // displays 10 & 20
Complex c2 = new Complex();
c2.ShowXY(); // displays 0 & 0
c2 = -c1;
c2.ShowXY(); // displays -10 & -20
}
}
Output
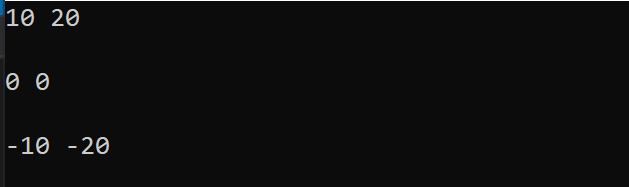
Binary Operator Overloading
- We must seek two arguments, to overload a binary operator and we need to ensure that one of the operators should be of type class or struct where the operator is defined.
- A binary operator cannot return void, but it can return all other types of values while implementing overloading.
Syntax
public static return_type operator op (Type1 t1, Type2 t2) { //Statements }
Sample Code
using System;
class Complex
{
private int x;
private int y;
public Complex()
{
}
public Complex(int i, int j)
{
x = i;
y = j;
}
public void ShowXY()
{
Console.WriteLine("{0} {1}", x, y);
Console.ReadLine();
}
public static Complex operator +(Complex c1, Complex c2)
{
Complex temp = new Complex();
temp.x = c1.x + c2.x;
temp.y = c1.y + c2.y;
return temp;
}
}
class MyClient
{
public static void Main()
{
Complex c1 = new Complex(10, 20);
c1.ShowXY(); // displays 10 & 20
Complex c2 = new Complex(20, 30);
c2.ShowXY(); // displays 20 & 30
Complex c3 = new Complex();
c3 = c1 + c2;
c3.ShowXY(); // dislplays 30 & 50
}
}
Output
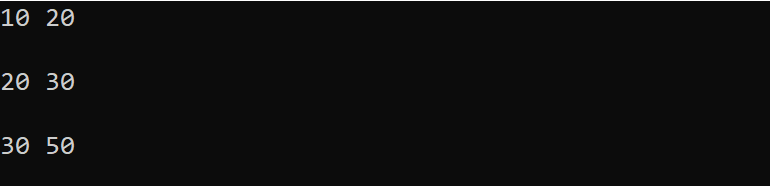
Operator overloading & Inheritance
- If you declare the overloaded operators as static, they will inherit the derived class.
- This happens because the operator's declaration requires it to be struct or class where the operator is being declared and it facilitates the operator's signature.
- Thus, it is not possible for an operator previously declared in the derived class to hide an operator present in the parent class already.
- The new modifier is not an option to consider since they are not allowed while declaring the operator.
Sample Code
using System;
class Complex
{
private int x;
private int y;
public Complex()
{
}
public Complex(int i, int j)
{
x = i;
y = j;
}
public void ShowXY()
{
Console.WriteLine("{0} {1}", x, y);
Console.ReadLine();
}
public static Complex operator +(Complex c1, Complex c2)
{
Complex temp = new Complex();
temp.x = c1.x + c2.x;
temp.y = c1.y + c2.y;
return temp;
}
}
class MyComplex : Complex
{
private double x;
private double y;
public MyComplex(double i, double j)
{
x = i;
y = j;
}
public MyComplex()
{
}
public new void ShowXY()
{
Console.WriteLine("{0} {1}", x, y);
Console.ReadLine();
}
}
class MyClient
{
public static void Main()
{
MyComplex mc1 = new MyComplex(1.5, 2.5);
mc1.ShowXY();
MyComplex mc2 = new MyComplex(3.5, 4.5);
mc2.ShowXY();
MyComplex mc3 = new MyComplex();
//mc3 = mc1 + mc2;
//mc3.ShowXY();
}
}
Output

Equality Operator Overloading
- In C#, we might have already been familiar with all the user-defined classes that inherit Syste.object from the Syste.object.Equals() method by default.
- The reference-based comparison is provided by the Equals () method but, there is a possibility that this method can override the methods residing inside the class defined by the user.
- Hence, value-based comparison can be easily achieved by using this method and this is how the Equality operator is operated.
- We need to follow the following code snippet, to overload this operator.
Example
