C# StreamWriter - c# - c# tutorial - c# net
What is StreamWriter Class in C# ?
- StreamWriter Class is more popular in File Handling and it is very helpful in writing text data in file.
- It is easy to use and provides complete set of constructors and methods to work.
- C# StreamWriter class is used to write characters to a stream in specific encoding.
- It inherits TextWriter class.
- It provides overloaded write() and writeln() methods to write data into file.
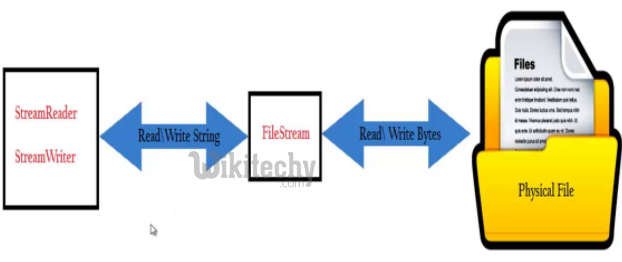
stream writer and stream reader
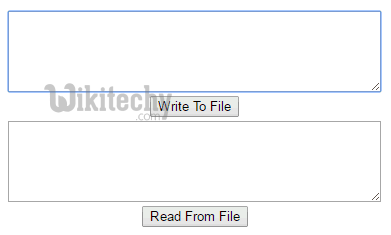
C# StreamWriter
C# StreamWriter example:
- Let's see a simple example of StreamWriter class which writes a single line of data into the file.
using System;
using System.IO;
public class StreamWriterExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\output.txt", FileMode.Create);
StreamWriter s = new StreamWriter(f);
s.WriteLine("wikitechy c#");
s.Close();
f.Close();
Console.WriteLine("File created successfully...");
}
}
C# examples - Output :
File created successfully...
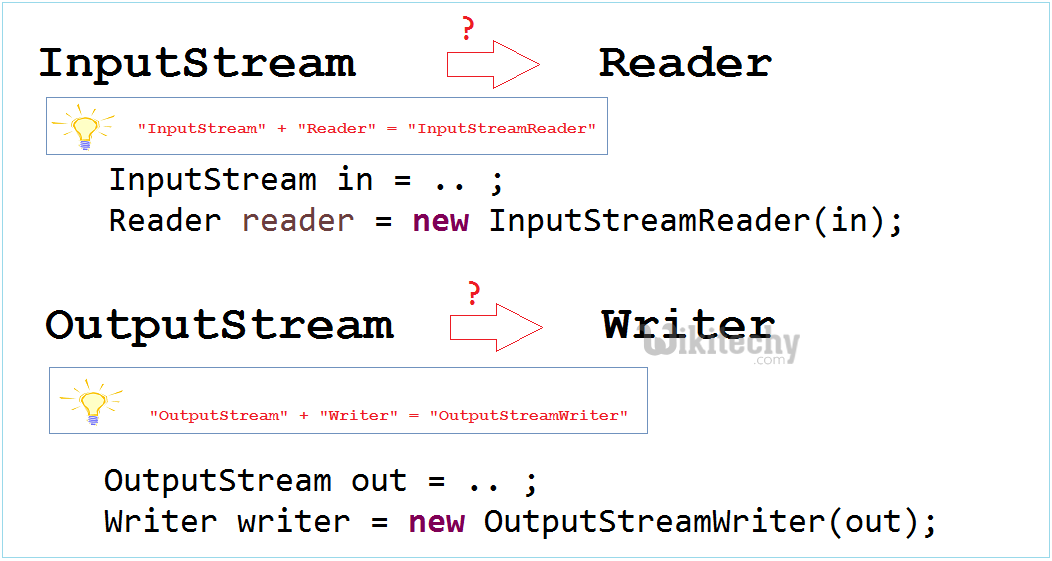
stream writer and stream reader
- Now open the file, you will see the text "hello c#" in output.txt file.
output.txt:
wikitechy c#
SAVE VARIABLE DATA TO FILE:
- Several times you need to save variable data in a file.
- These variable data might be output of your program, log details, exception error etc.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace StreamWriter_VariableData
{
class Program
{
static void Main(string[] args)
{
string file = @"D:\csharpfile.txt";
int a, b, result;
a = 5;
b = 10;
result = a + b;
using (StreamWriter writer = new StreamWriter(file))
{
writer.Write("Sum of {0} + {1} = {2}", a, b, result);
}
Console.WriteLine("Saved");
Console.ReadKey();
}
}
}
- Now open the D:\csharpfile.txt again and you will see the following text in a file.
- Sum of 5 + 10 = 15