C# Combobox - c# - c# tutorial - c# net
How to use Combobox in C# ?
- In general, Windows form represents a combo box control.
- In C# controls, ComboBox is a combination of TextBox with a drop-down.
- This drop-down list presents certain choices.
- The user can type anything into the ComboBox.
- Only one list item is displayed at one time in a ComboBox and other available items are loaded in a drop down list.
- The ComboBox class represents a control that encapsulates other controls.
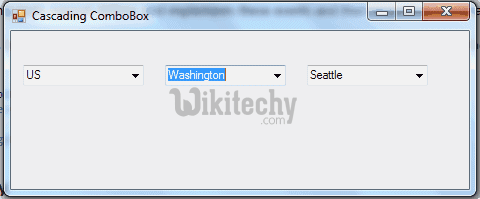
Combobox
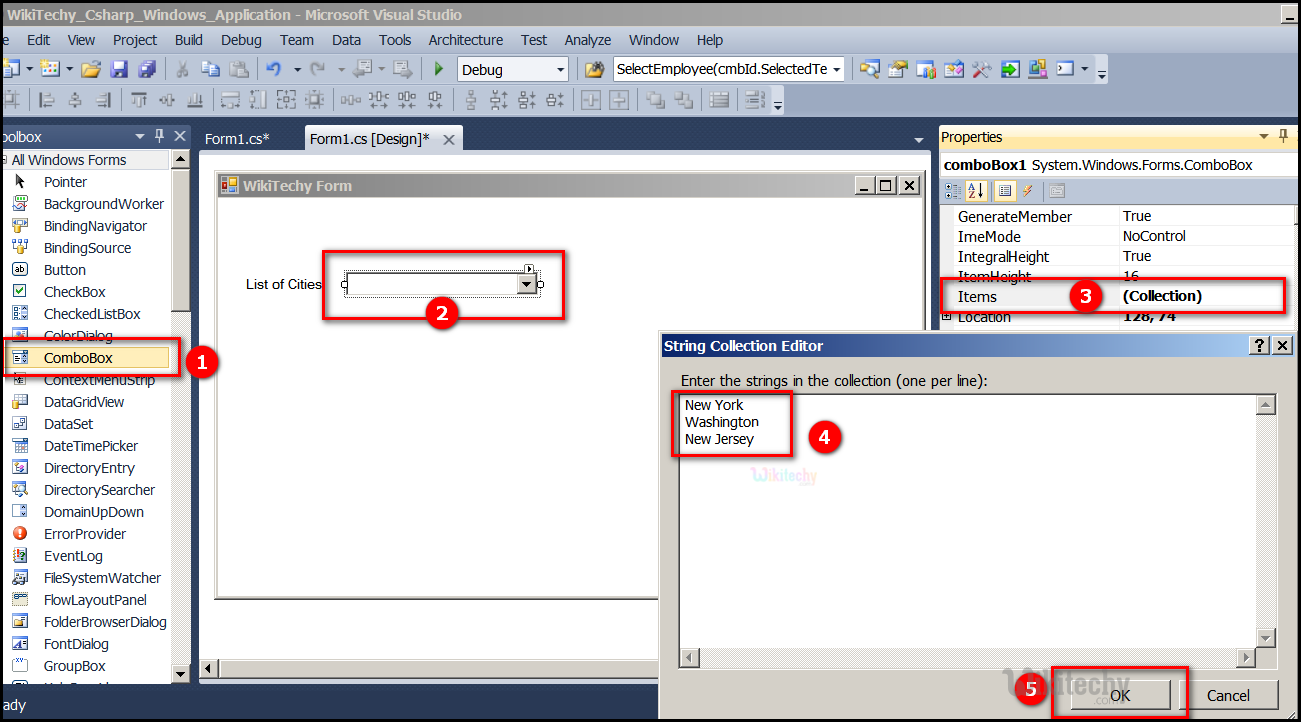
- Go to tool box and select Combobox, here it is displays an editable with a drop-down list of the permitted values.
- Go to tool box and click on the combobox option the drop down list will be open.
- Go to properties select Item Collection, here it is specifying to holds the list of items that constitute the content of an ItemsControl.
- When we click on the Collections, the String Collection Editor window will pop up where you can type strings. Each line added to this collection will become a ComboBox item. Here we add three items New York, Washington, New jersey.
- After click on "ok" button these items are including in the drop down list of cities.
Common Properties
Height="23"
HorizontalAlignment="Left"
Margin="112,87,0,0"
Name="comboBox1"
VerticalAlignment="Top"
Width="120" />
Read Also
dot net training topics , best dotnet training in chennai , dot net course in chennai with placementSample C# examples - Output :
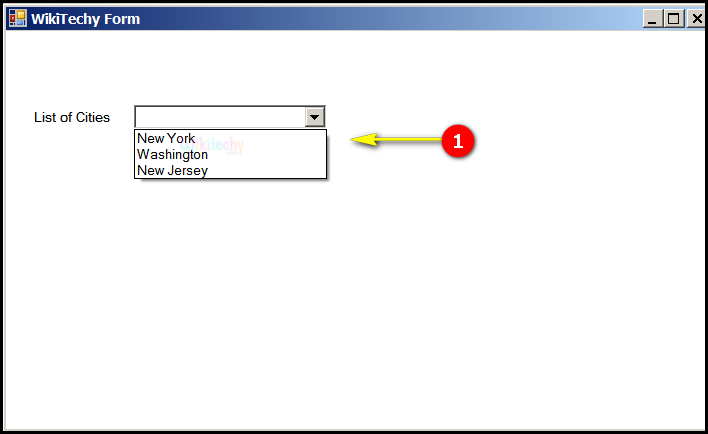
- Here in this output table displays the List of Cities "New York, Washington, New jersey".
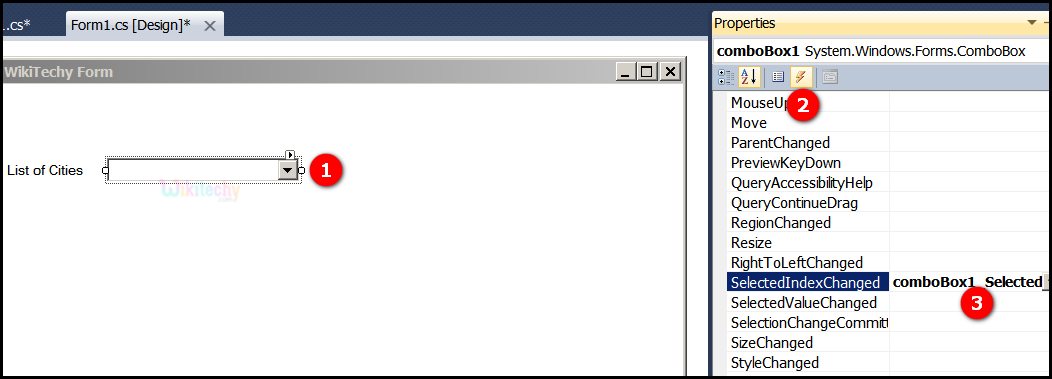
- item collection is specifying to holds the list of items that constitute the content of an ItemsControl.
- To add these event handlers, you go to Events window and double click on CheckedChanged and CheckedStateChanged events.
- And choose the SelectedIndexChanged option.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Click(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
MessageBox.Show(comboBox1.SelectedItem.ToString());
}
}
}
Code Explanation:
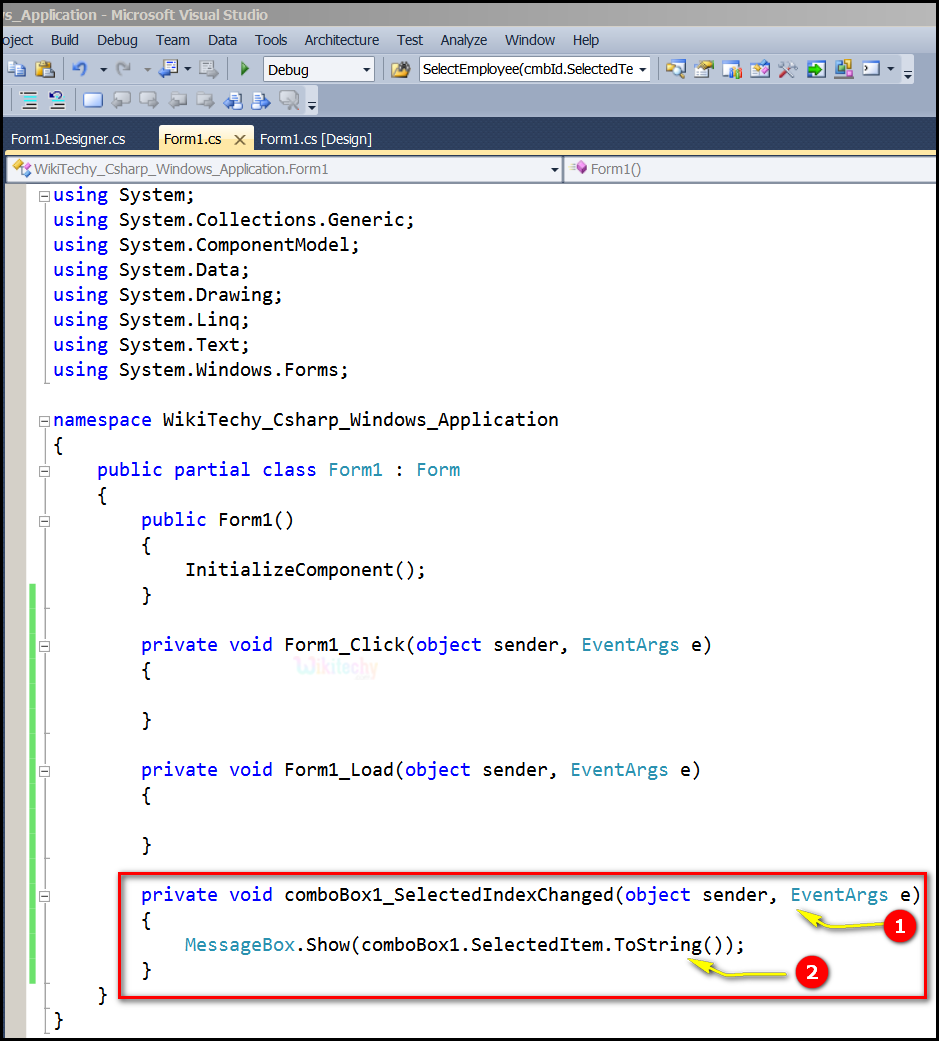
- Here private void comboBox1_SelectedIndexChanged (object sender, EventArgs e) Occurs when the SelectedIndex property has changed. The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void comboBox1_SelectedIndexChanged (object sender, EventArgs e).
- MessageBox.Show(comboBox1.SelectedItem.ToString()); specifies to shows the selected items of the string.
Sample C# examples - Output :
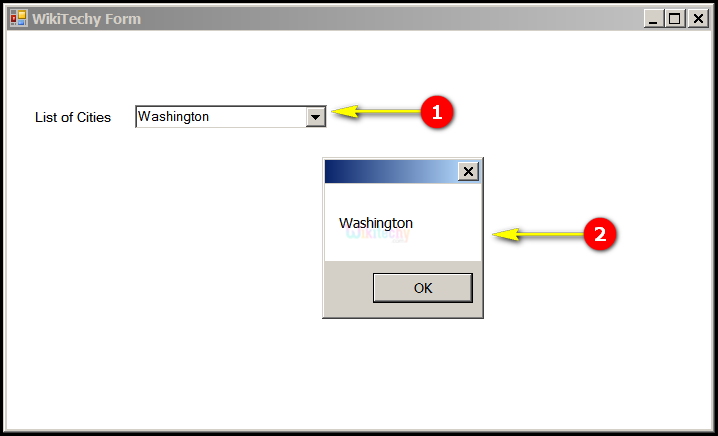
- Here in this output table we display the List of Cities "Washington".
- Here in this output we display the Washington massage box
Dynamic loading of data through C# code:
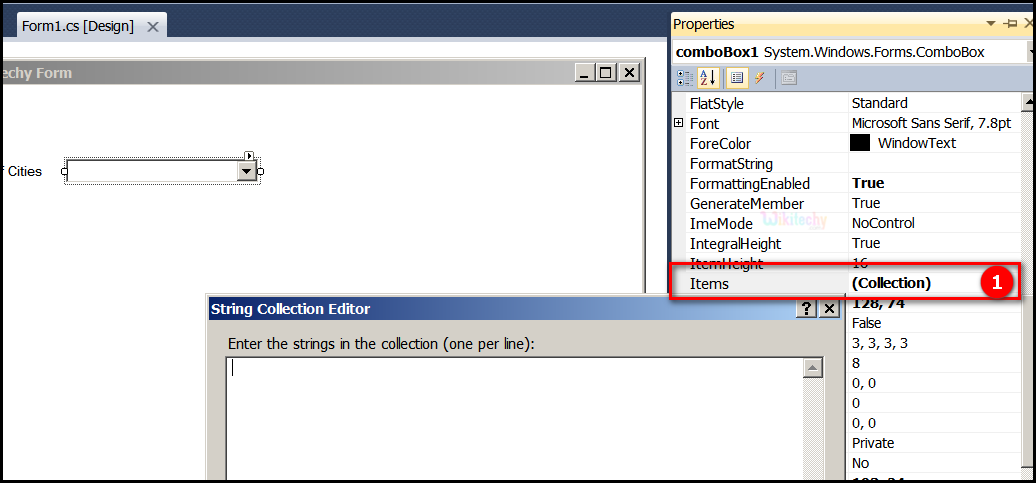
- When we click on the Collections, the String Collection Editor window will pop up where you can type strings. Each line added to this collection will become a ComboBox item.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Click(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
List<string> list=new List<string>();
list.Add("DotNet");
list.Add("Java");
list.Add("SQL Server");
comboBox1.DataSource = list;
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
MessageBox.Show(comboBox1.SelectedItem.ToString());
}
}
}
Code Explanation:
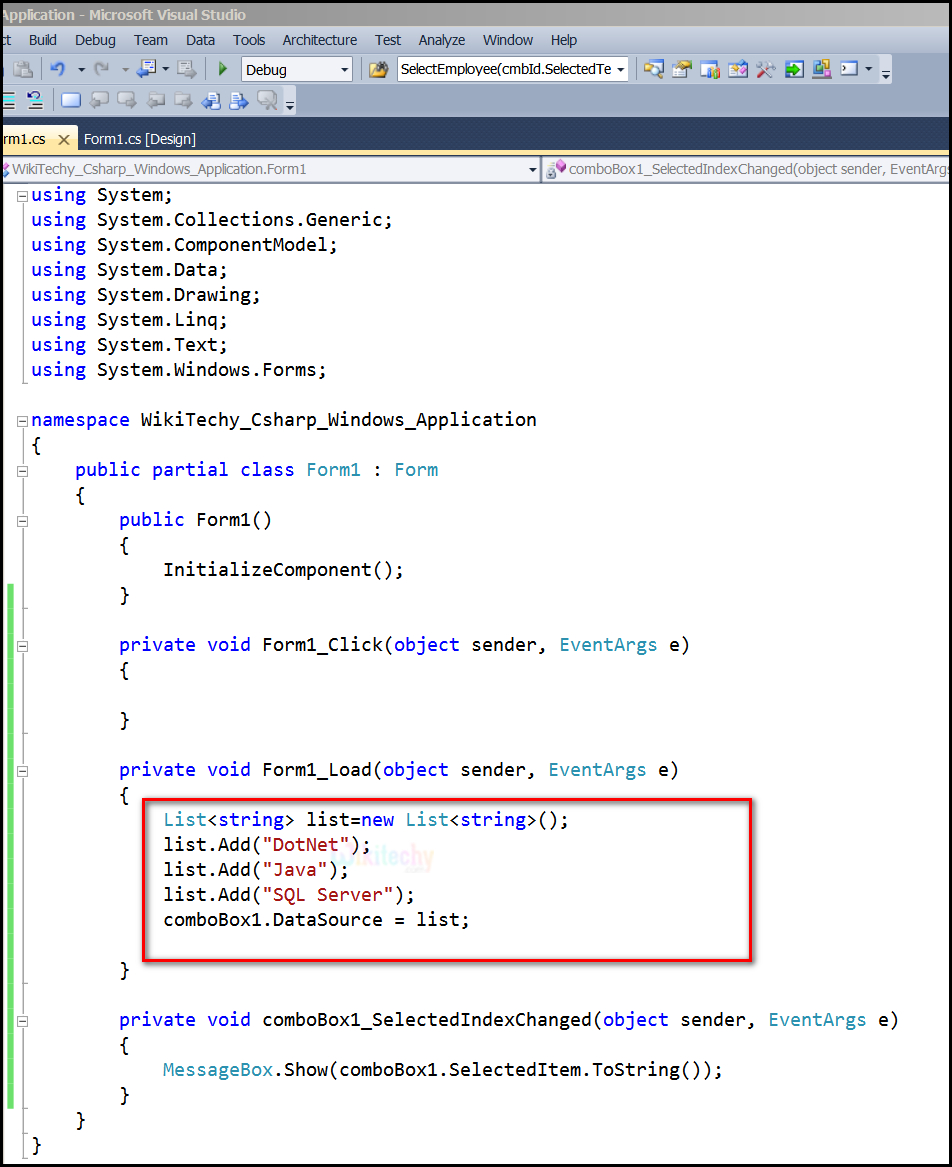
- List<string> list=new List<string>(); specifies to list of string objects.
- In this example list.Add("DotNet"); list.Add("Java"); list.Add("SQL Server"); specifies to add the string of "List of Cities" .
- comboBox1.DataSource = list; used to specify the data source of comboBox and the table which will be bind to. When we run the code a window will show with a comboBox that containing all the returning rows.
Selected Index
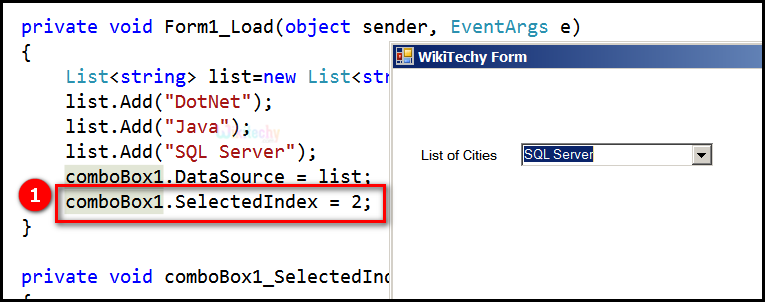
- comboBox1.SelectedIndex =2; Gets or Sets the index specifying the currently selected item is 2
Sample C# examples - Output :
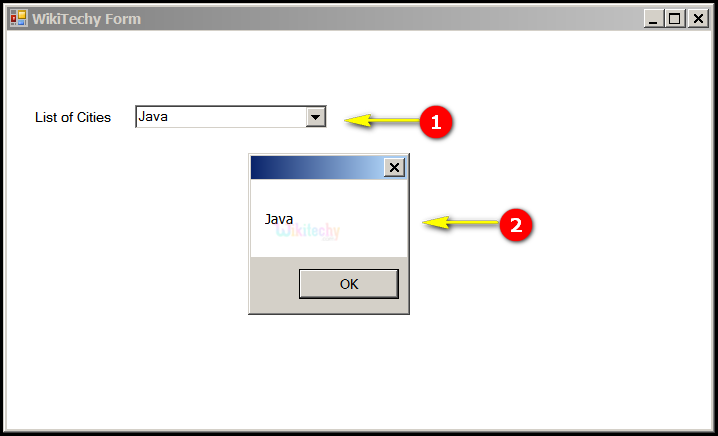
- Here in this output table we display the List of Cities "Java".
- Here in this output table we display the Java massage box.