C# Reflection | Reflection in C# with Examples
C# Reflection
- In C#, reflection is a process to get metadata of a type at runtime.
- The System.Reflection namespace contains required classes for reflection such as:
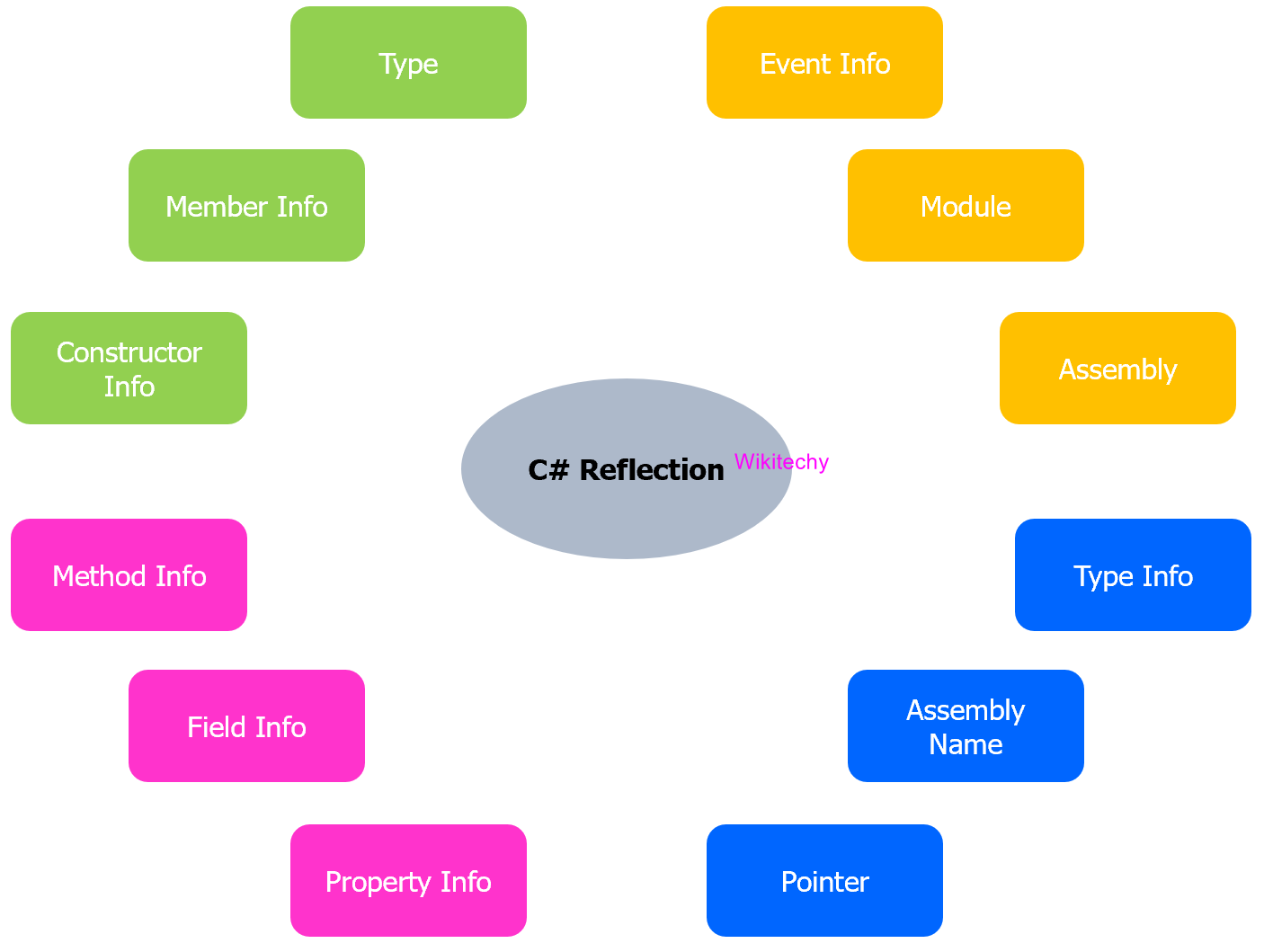
C# Type Class
- In C#, Type class represents type declarations for interface types, class types, array types, enumerations types and value types, etc.
- It is found in System namespaces and it inherits System. Reflection. Member Info class.
Property | Description |
---|---|
Assembly | Gets the Assembly for this type |
AssemblyQualifiedName | Gets the Assembly qualified name for this type |
Attributes | Gets the Attributes associated with the type |
Base Type | Gets the base or parent type. |
Full Name | Gets the fully qualified name of the type. |
Is Abstract | Is used to check if the type is Abstract. |
Is Array | Is used to check if the type is Array. |
Is Class | Is used to check if the type is Class. |
Is Enum | Is used to check if the type is Enum. |
Is Interface | Is used to check if the type is Interface. |
Is Nested | Is used to check if the type is Nested. |
Is Primitive | Is used to check if the type is Primitive. |
Is Pointer | Is used to check if the type is Pointer |
Is Not Public | Is used to check if the type is not Public. |
Is Public | Is used to check if the type is Public. |
Is Sealed | Is used to check if the type is Sealed. |
Is Serializable | Is used to check if the type is Serializable. |
Member Type | Is used to check if the type is Member type of Nested type. |
Module | Gets the module of the type |
Name | Gets the name of the type |
Namespace | Gets the namespace of the type |
C# Type Methods
Method | Description |
---|---|
Get Constructors () | Returns all the public constructors for the Type |
Get Constructors (Binding Flags) | Returns all the constructors for the Type with specified Binding Flags. |
Get Fields () | Returns all the public fields for the Type. |
Get Fields (Binding Flags) | Returns all the public constructors for the Type with specified Binding Flags. |
Get Members (Binding Flags) | Returns all the members for the Type with specified Binding Flags. |
Get Methods (Binding Flags) | Returns all the methods for the Type with specified Binding Flags. |
Get Properties () | Returns all the public properties for the Type. |
Get Properties (Binding Flags) | Returns all the properties for the Type with specified BindingFlags. |
Get Type () | Gets the current Type. |
Get Type (String) | Gets the Type for the given name. |
C# Reflection Example: Get Type
using System;
public class ReflectionExample
{
public static void Main()
{
int a = 10;
Type type = a.GetType();
Console.WriteLine(type);
}
}
Output
System.Int32
C# Reflection Example: Get Assembly
using System;
using System.Reflection;
public class ReflectionExample
{
public static void Main()
{
Type t = typeof(System.String);
Console.WriteLine(t.Assembly);
}
}
Output

C# Reflection Example: Print Constructors
using System;
using System.Reflection;
public class ReflectionExample
{
public static void Main()
{
Type t = typeof(System.String);
Console.WriteLine("Constructors of {0} type...", t);
ConstructorInfo[] ci = t.GetConstructors(BindingFlags.Public | BindingFlags.Instance);
foreach (ConstructorInfo c in ci)
{
Console.WriteLine(c);
}
}
}
Output
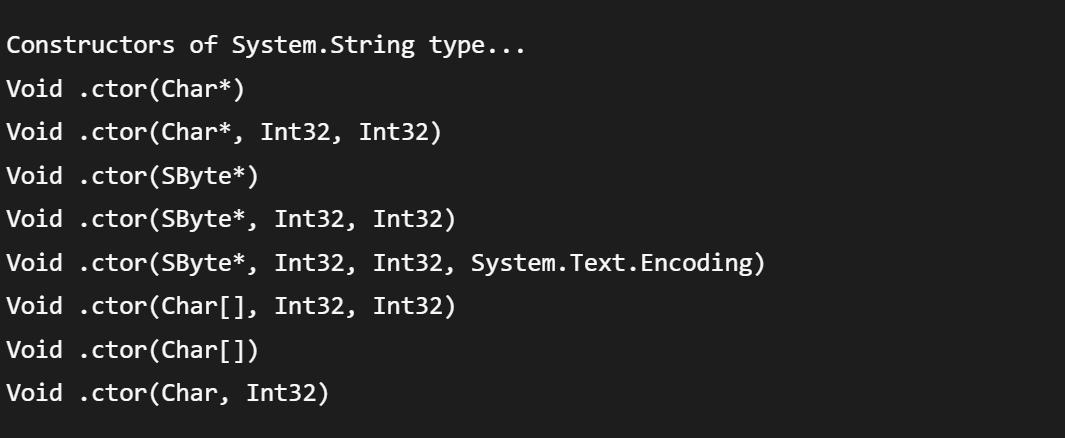
C# Reflection Example: Print Methods
using System;
using System.Reflection;
public class ReflectionExample
{
public static void Main()
{
Type t = typeof(System.String);
Console.WriteLine("Methods of {0} type...", t);
MethodInfo[] ci = t.GetMethods(BindingFlags.Public | BindingFlags.Instance);
foreach (MethodInfo m in ci)
{
Console.WriteLine(m);
}
}
}
Output
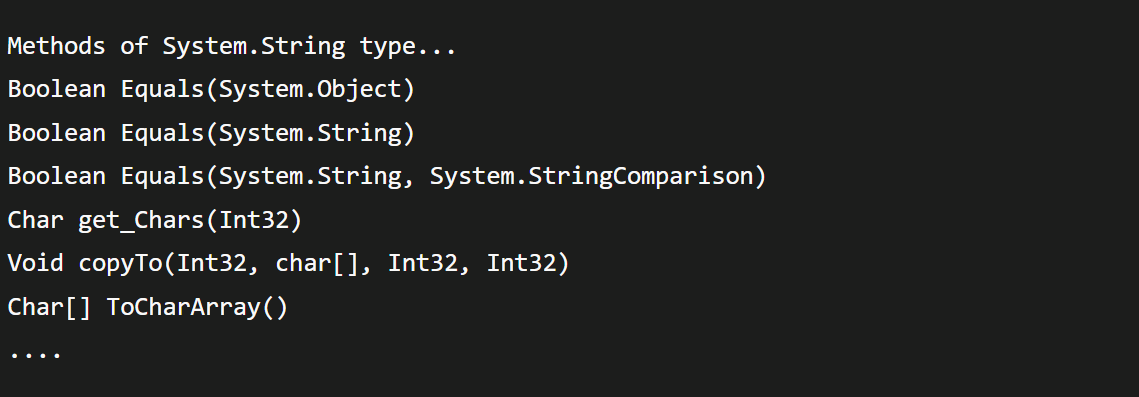
C# Reflection Example: Print Fields
using System;
using System.Reflection;
public class ReflectionExample
{
public static void Main()
{
Type t = typeof(System.String);
Console.WriteLine("Fields of {0} type...", t);
FieldInfo[] ci = t.GetFields(BindingFlags.Public | BindingFlags.Static | BindingFlags.NonPublic);
foreach (FieldInfo f in ci)
{
Console.WriteLine(f);
}
}
}
Output
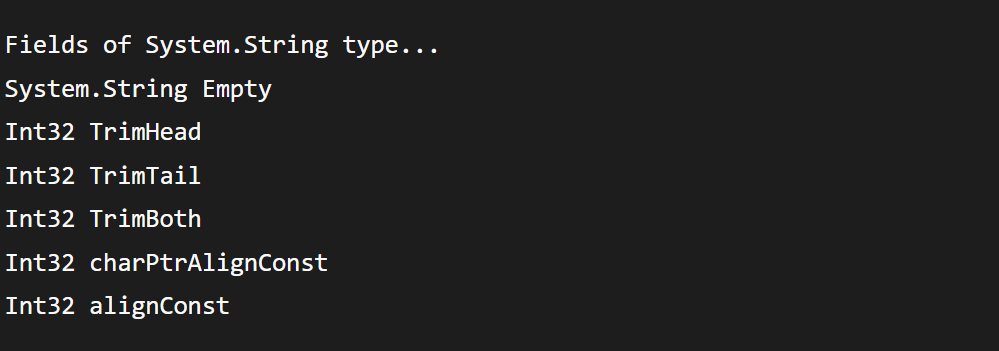