C# Jagged array - c# - c# tutorial - c# net
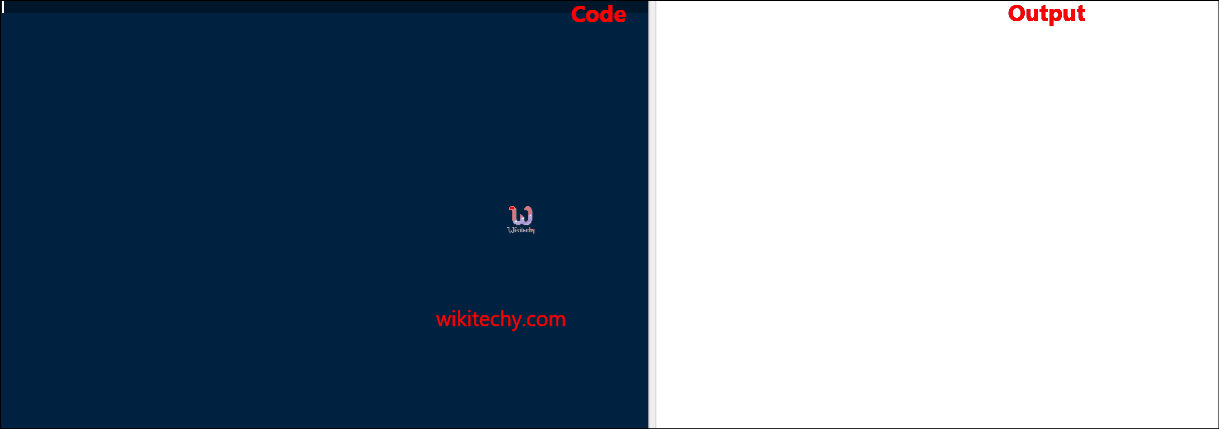
C# Jagged Array
What is jagged array in C# ?
- A jagged array is an array whose elements are arrays.
- The elements of a jagged array can be of different dimensions and sizes.
- A jagged array is sometimes called an "array-of-arrays".
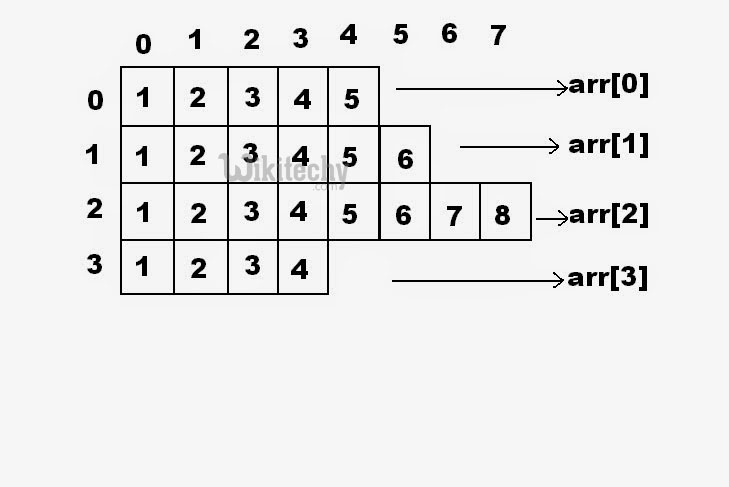
Syntax:
DataType[][] VariableName;
Syntax Explanation:
- datatype:
- Datatype is used to specify the type of elements in the array.
- [] []:
- In jagged arrays, the first square bracket can be used as its own one-dimensional array or as a multi-dimensional array.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_Jagged_arrays
{
class Program
{
static void Main(string[] args)
{
int[][] array = new int[][] { new int[] { 0, 0 }, new int[] { 1, 2 },
new int[] { 2, 1 }, new int[] { 3, 1 }, new int[] { 4, 1 } };
int m, n;
for (m = 0; m < 4; m++)
{
for (n = 0; n < 2; n++)
{
Console.WriteLine("array of [{0}][{1}] is: = {2}", m, n, array[m][n]);
}
}
Console.ReadKey();
}
}
}
Code Explanation:
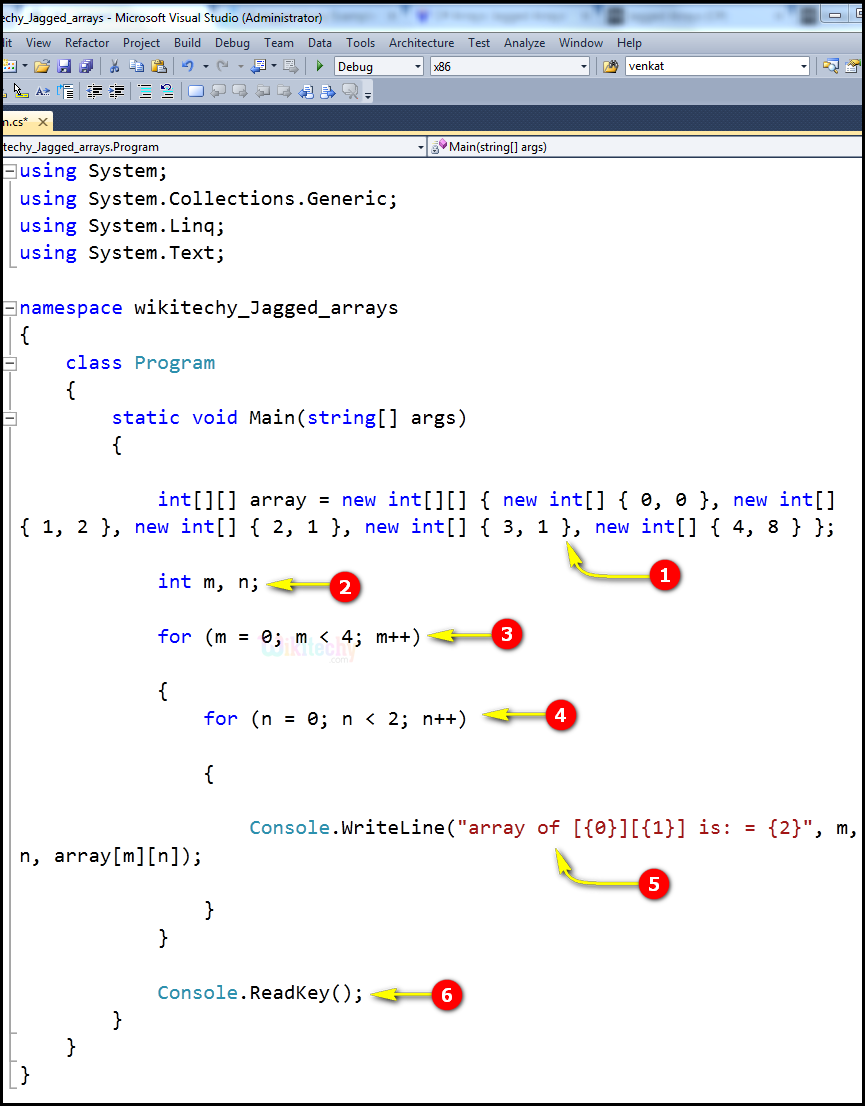
- We declare and initialize a numerical array. And we declare jagged array of 5 array of integers.
- Here int m, n ; is an integer variables.
- for (m = 0; m< 4; m++) specifies integer m = 0, which means the expression, (m < 4), is true. Therefore, the statement is executed, and m gets incremented by 1 and becomes less than 3.
- for (n = 0; n< 2; n++) specifies integer n = 0, which means the expression, (n < 2), is true. Therefore, the statement is executed, and n gets incremented by 1 and becomes less than 2.
- Here we print the value of "m, n, array[m][n]" using the "array of" statement.
- Obtains the next character or function key pressed by the user. The pressed key is displayed in the console window.
Sample C# examples - Output :
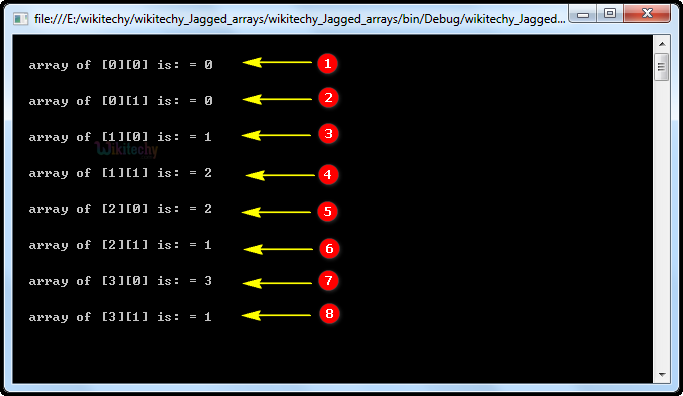
- Here in this output the jagged array elements [0][0] is represented as a console statement. The array of elements [0][0] value as "0"
- Here in this output the jagged array elements [0][1] is represented as a console statement. The array of elements [0][1] value as "0"
- Here in this output the jagged array elements [1][0] is represented as a console statement. The array of elements [1][0] value as "1"
- Here in this output the jagged array elements [1][1] is represented as a console statement. The array of elements [1][1] value as "2"
- Here in this output the jagged array elements [2][0] is represented as a console statement. The array of elements [2][0] value as "2"
- Here in this output the jagged array elements [2][1] is represented as a console statement. The array of elements [2][1] value as "1"
- Here in this output the jagged array elements [3][0] is represented as a console statement. The array of elements [3][0] value as "3"
- Here in this output the jagged array elements [3][1] is represented as a console statement. The array of elements [3][1] value as "1"