C# Program for Palindrome - c# - c# tutorial - c# net
How to write palindrome Program in C# ?
- A palindrome number is a number that is same after reverse.
- For example - 121, 34543, 343, 131, 48984 are the palindrome numbers.
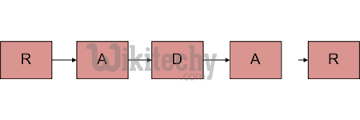
Palindrome number algorithm
- Get the number from user
- Hold the number in temporary variable
- Reverse the number
- Compare the temporary number with reversed number
- If both numbers are same, print palindrome number
- Else print not palindrome number
- Let's see the palindrome program in C#. In this program, we will get an input from the user and check whether number is palindrome or not.
Example:
class Program
{
static void Main(string[] args)
{
string s,revs="";
Console.WriteLine(" Enter string");
s = Console.ReadLine();
for (int i = s.Length-1; i >=0; i--) //String Reverse
{
revs += s[i].ToString();
}
if (revs == s) // Checking whether string is palindrome or not
{
Console.WriteLine("String is Palindrome \n Entered String Was {0} and reverse string is {1}", s, revs);
}
else
{
Console.WriteLine("String is not Palindrome \n Entered String Was {0} and reverse string is {1}", s, revs);
}
Console.ReadKey();
}
}
C# Sample Code - C# Examples:
using System;
public class PalindromeExample
{
public static void Main(string[] args)
{
int n,r,sum=0,temp;
Console.Write("Enter the Number: ");
n = int.Parse(Console.ReadLine());
temp=n;
while(n>0)
{
r=n%10;
sum=(sum*10)+r;
n=n/10;
}
if(temp==sum)
Console.Write("Number is Palindrome.");
else
Console.Write("Number is not Palindrome");
}
}
C# examples - Output :
Enter the Number=121
Number is Palindrome.
Enter the number=113
Number is not Palindrome.