C# Sum - c# - c# tutorial - c# net
How to write Sum Program in C# ?
- The C# Program Gets a Number and Display the Sum of the Digits. The digit sum of a given integer is the sum of all its digits.
- We can write the sum of digits program in C# language by the help of loop and mathematical operation only.
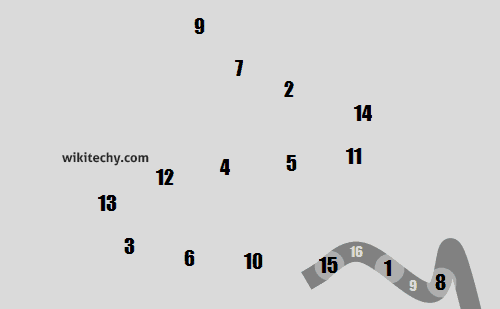
C# Sum
Sum of digits algorithm
- To get sum of each digit by C# program, use the following algorithm:
- Step 1: Get number by user
- Step 2: Get the modulus/remainder of the number
- Step 3: sum the remainder of the number
- Step 4: Divide the number by 10
- Step 5: Repeat the step 2 while number is greater than 0.
- Let's see the sum of digits program in C#
Example 1:
using System;
public class SumExample
{
public static void Main(string[] args)
{
int n,sum=0,m;
Console.Write("Enter a number: ");
n= int.Parse(Console.ReadLine());
while(n>0)
{
m=n%10;
sum=sum+m;
n=n/10;
}
Console.Write("Sum is= "+sum);
}
}
C# examples - Output :
Enter a number: 23
Sum is= 5
Enter a number: 624
Sum is= 12
Read Also
.net training institute near me , dot net full cource , dot net training course fees in chennaiExample 2:
/*
* C# Program to Get a Number and Display the Sum of the Digits
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Program
{
class Program
{
static void Main(string[] args)
{
int num, sum = 0, r;
Console.WriteLine("Enter a Number : ");
num = int.Parse(Console.ReadLine());
while (num != 0)
{
r = num % 10;
num = num / 10;
sum = sum + r;
}
Console.WriteLine("Sum of Digits of the Number : "+sum);
Console.ReadLine();
}
}
}
C# examples - Output :
Enter a Number: 123
Sum of Digits of the Number: 6