C# control events - Events in c# - c# - c# tutorial - c# net
C# Events
- The Event is something special that is going to happen where Microsoft launches the events for the developer.
- Microsoft wants to aware the developer about the feature of the existing or new products, in this event.
- For this, Microsoft utilize Email or other advertisement options to aware the developer about the Event.
- At this case, Microsoft will work as a publisher who raises the Event and notifies the developers about it.
- Here, developers will work as the subscriber of the Event who handles the Event.
- At C#, Event can be publisher, subscriber, notification and a handler.
- Generally, the User Interface uses the events and here we will take an example of Button control in Windows.
- In C#, Button performs multiple events such as click, mouseover, etc.
- The custom class contains the Event through which we will notify the other subscriber class about the other things which is going to happen.
- In this case, we will define the Event and inform the other classes about the Event, which contains the event handler.
- In C# and .NET both support the events with the delegates and it is an encapsulated delegate.
- Events and delegates give the notification to the client application, when the state of the application changes.
- Events and delegates both are tightly coupled for dispatching the events, and event handling require the implementation of the delegates.
- The sending event class is known as the publisher, and the receiver class or handling the Event is known as a subscriber.
Key Points about the Events are
- At C#, event handler will take the two parameters as input and return the void.
- The first parameter of the Event is also known as the source, which will publish the object.
- The subscriber will determine what response we have to give, where the publisher will decide when we have to raise the Event.
- Generally, we used the Event for the single user action like clicking on the button.
c# Button Control Events… :
- OnClick – when user clicks a button
- OnCommand – When user clicks button
- OnLoad – When page first loads and button loads
- OnInit – When button is initialized
- OnPreReader- just before button is drawn
- OnUnload – When button is unloaded from memory
- OnDisposed – When button is released from memory
- OnDataBinding – When button is bound to a data source
Declaration of the event
Syntax
public event EventHandler CellEvent;
Steps for implementing the Event
- Firstly, the event type of the delegate must be declared, for the declaration of the Event in the class.
public delegate void CellEventHandler(object sender, EventArgs e);
Declaration of the Event
public event CellEventHandler CellEvent;
Invokation of the Event
if (CellEvent != null) CellEvent(this, e);
Hooking up the Event
OurEventClass.OurEvent += new ChangedEventHandler(OurEventChanged);
Detach the Event
OurEventClass.OurEvent -= new ChangedEventHandler(OurEventChanged);
Delegates
- Delegates works as a pointer to a function and it is a reference data type and it holds the reference of the method. System.Delegate class implicitly derived all the delegates.
Syntax
<access modifier> delegate <return type> <delegate_name>(<parameters>)
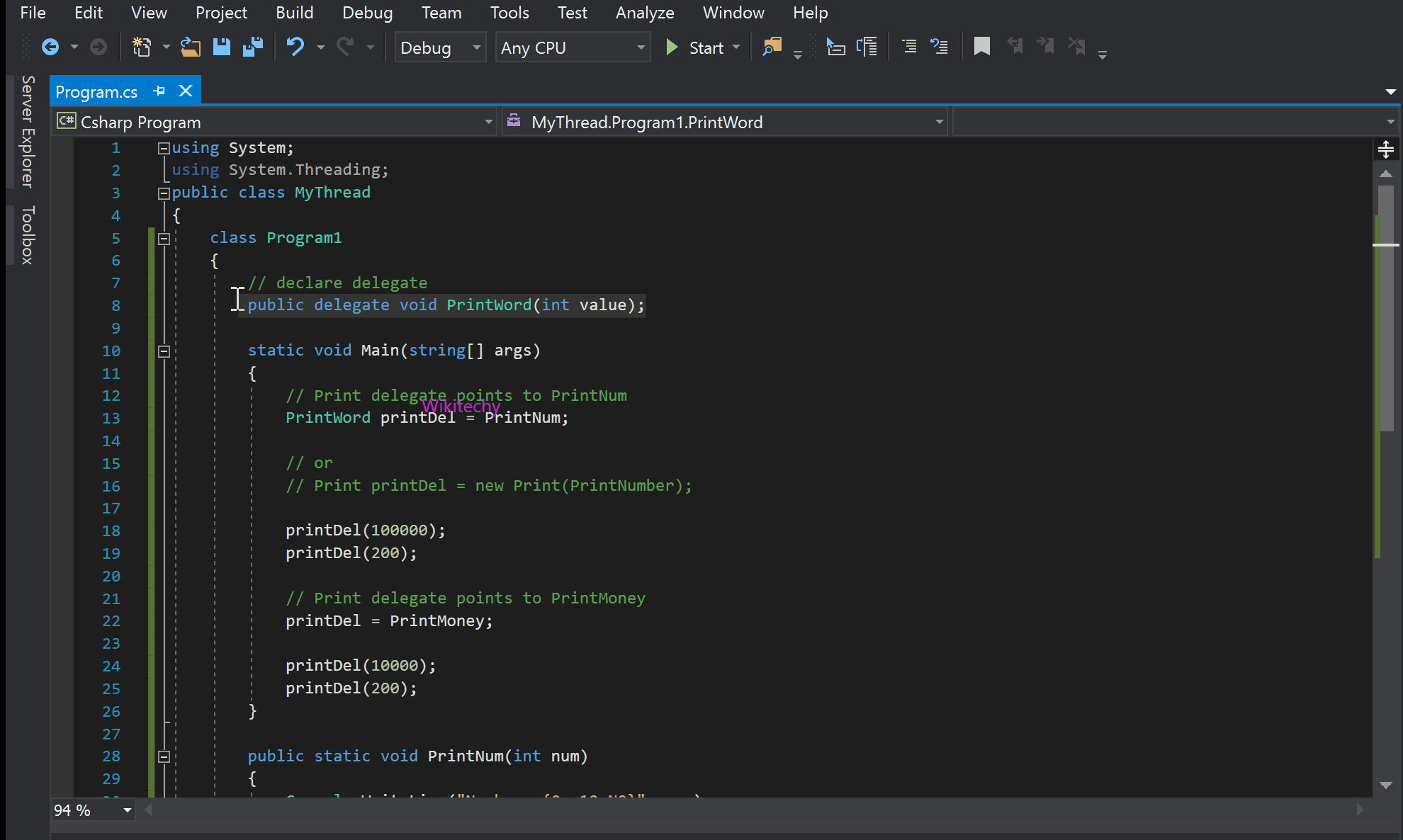
Sample Code
using System;
using System.Threading;
public class MyThread
{
class Program1
{
// declare delegate
public delegate void PrintWord(int value);
static void Main(string[] args)
{
// Print delegate points to PrintNum
PrintWord printDel = PrintNum;
// or
// Print printDel = new Print(PrintNumber);
printDel(100000);
printDel(200);
// Print delegate points to PrintMoney
printDel = PrintMoney;
printDel(10000);
printDel(200);
}
public static void PrintNum(int num)
{
Console.WriteLine("Number: {0,-12:N0}", num);
Console.ReadLine();
}
public static void PrintMoney(int money)
{
Console.WriteLine("Money: {0:C}", money);
Console.ReadLine();
}
}
}
Output
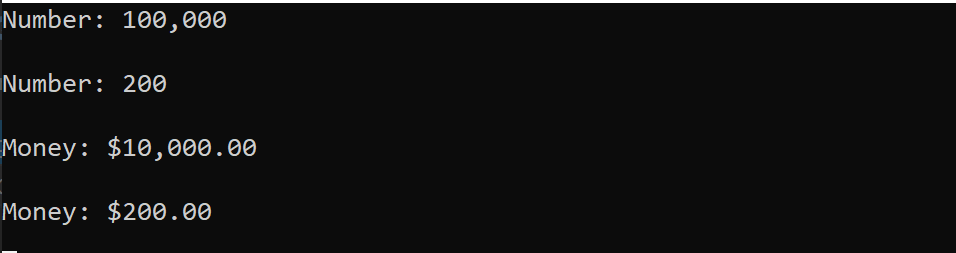