C# Swap | C# Program To Swap Two Numbers - c# - c# tutorial - c# net
How to write Swap Program in C# ?
- When the values of two variables are exchanged at runtime it is called swapping of the two values.
- We can swap two numbers without using third variable.
- There are two common ways to swap two numbers without using third variable:
- By + and -
- By * and /
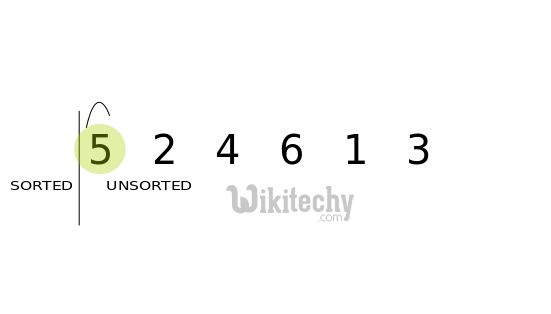
Swap numbers in csharp
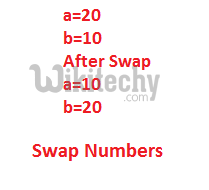
Program 1: Using ∗ and /
- Let's see a simple C# example to swap two numbers without using third variable.
using System;
public class SwapExample
{
public static void Main(string[] args)
{
int a=5, b=10;
Console.WriteLine("Before swap a= "+a+" b= "+b);
a=a*b; //a=50 (5*10)
b=a/b; //b=5 (50/10)
a=a/b; //a=10 (50/5)
Console.Write("After swap a= "+a+" b= "+b);
}
}
C# examples - Output :
Before swap a= 5 b= 10
After swap a= 10 b= 5
Read Also
dotnet summer internship , dot net training center in chennai with placement , dot net jobs in chennai for freshersProgram 2: Using + and -
- Let's see another example to swap two numbers using + and -.
using System;
public class SwapExample
{
public static void Main(string[] args)
{
int a=5, b=10;
Console.WriteLine("Before swap a= "+a+" b= "+b);
a=a+b; //a=15 (5+10)
b=a-b; //b=5 (15-10)
a=a-b; //a=10 (15-5)
Console.Write("After swap a= "+a+" b= "+b);
}
}
C# examples - Output :
Before swap a= 5 b= 10
After swap a= 10 b= 5