C# Controls Datagridview Add Image Column - c# - c# tutorial - c# net
What is Datagridview ?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
Add Image Column:
- In DataGrideViewImage, Gets or sets the image displayed in the cells of this column when the cell's Value property is not set and the cell's ValueIsIcon property is set to false.
- Here in the below table is representing to Windows Forms:
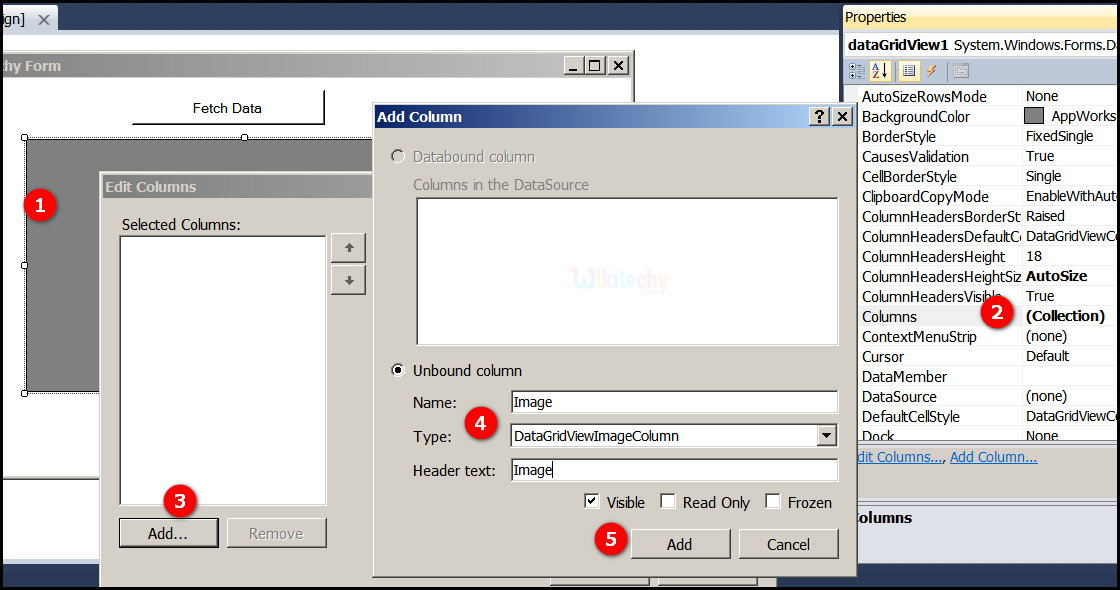
- Go to tool box and click on the DataGridview option the form will be open.
- When we click on the Collections, the String Collection Editor window will pop up where we can type strings. column collection is representing a collection of DataColumn objects for a DataTable.
- Click on "Add" button another Add column windows form will be open.
- After click on text box, and then select the DataGrideViewImage Column.
- And then click on the "Add" button, "Image" name is adding in Selected Columns windows form.
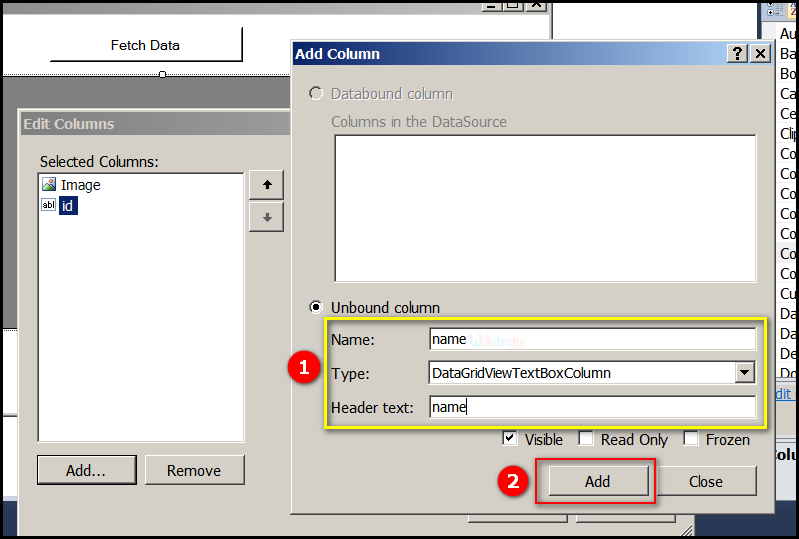
- Next adding name in the Name text box, Click on type box and then select the DataGrideViewTextBoxColumn in type text. After add name in the Header text box.
- Click on the "Add" button, "name" name is adding in Selected Columns windows form.
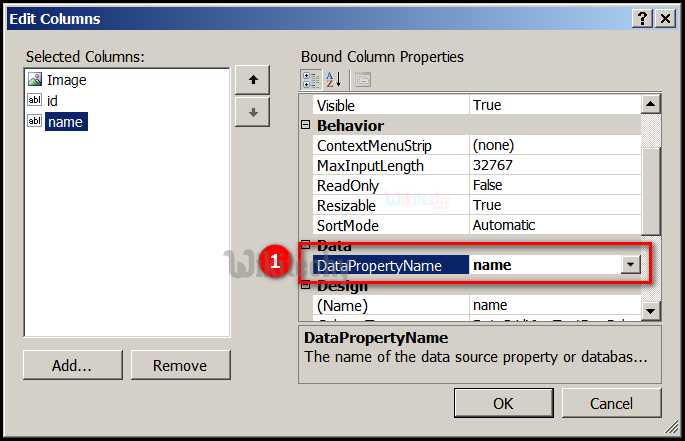
- In above diagram, the DataPropertyName is specifies to name of the property or database column associated with the DataGridViewColumn. Here we are giving name, this name is added in the Selected Columns.
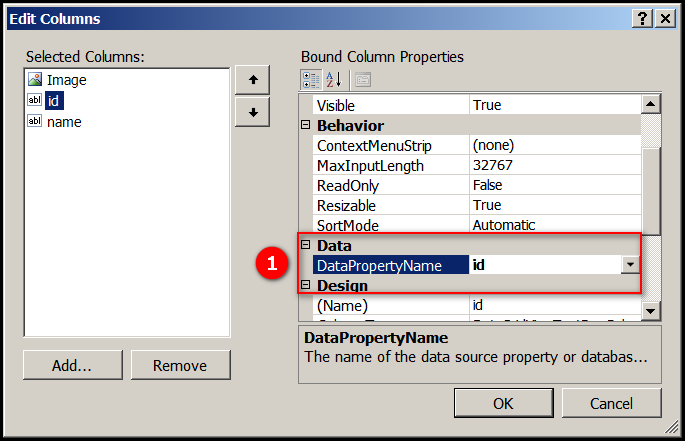
- In above diagram, the DataPropertyName (name of the property or database column associated with the DataGridViewColumn.) Here we are giving id, this id is added in the Selected Columns. Put id name in DataPropertyName and then click on "ok" button.
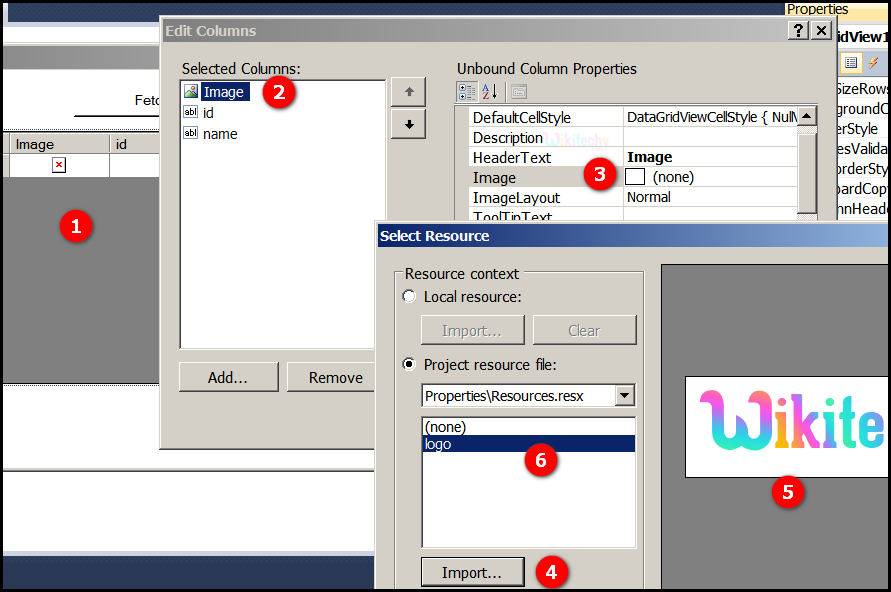
- After click on the ok button image and id table is opened.
- Go to properties click on column collection pop up Edit Columns window will open after choose the image button.
- Go to Unbound Columns properties select Image pop up Select Resource window is open.
- Click on import button after open windows is opened.
- After choose the image wikitechy. This is displayed on window.
- Here logo is the image name and double click on image. The image is stored in the Edit Columns window.
Read Also
ASPNET Training in chennai , dot net training center in chennai with placement , dot net online courses in indiaSample Output
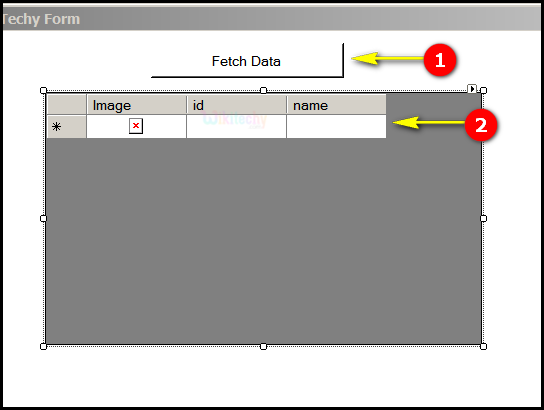
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Image, id, name.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
}
}
}
Code Explanation:
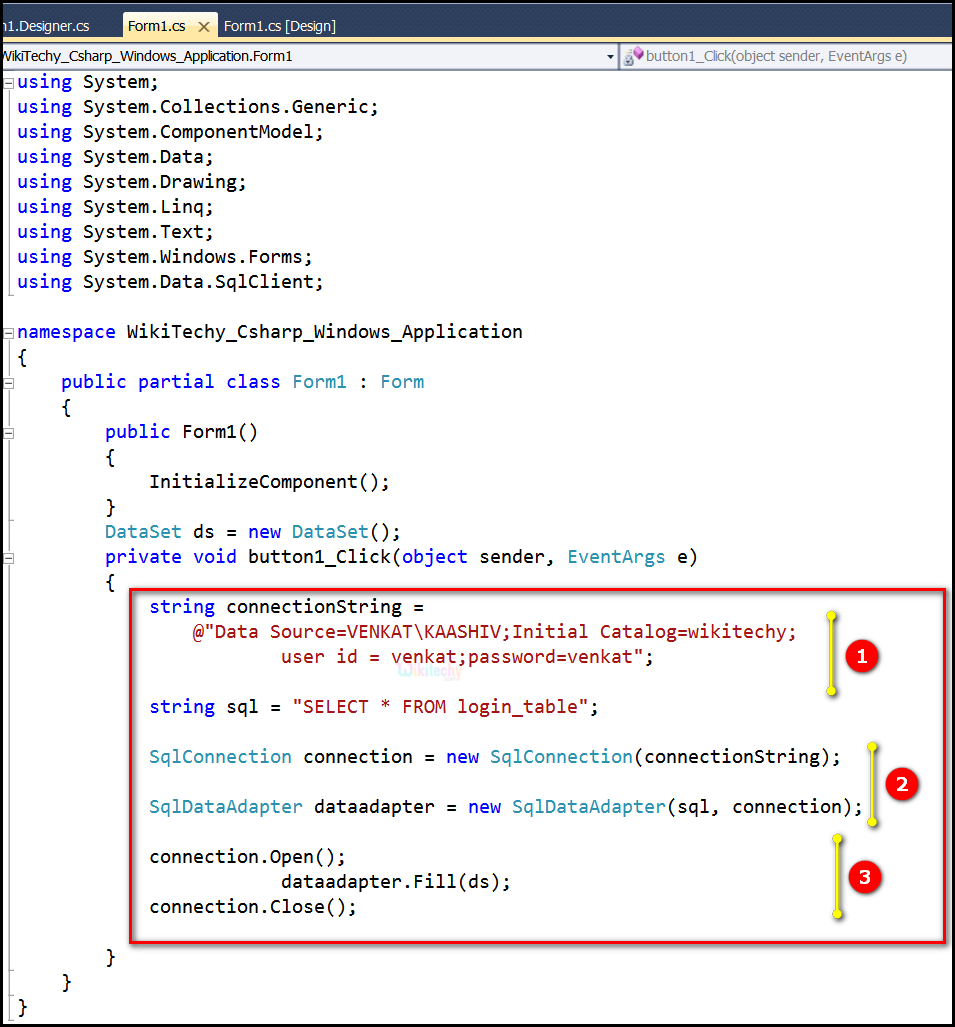
- string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;password=venkat"; connection string specifies that includes the source database name here the name is VENKAT\KAASHIV, and other parameters needed to establish the initial catalog connection is wikitechy. The default value is user id and password is venkat.In this example string sql = "SELECT * FROM login_table"; specifies to select the login_table in SQL server.
- SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource. SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection); specifies to initializes a new instance of the SqlDataAdapter class with a SelectCommand (sql) and a SqlConnection object(connection).
- connection.Open(); specifies to Open the SqlConnection.dataadapter.Fill(ds); specifies to fetches the data from User and fills in the DataSet ds.connection.Close(); it is used to close the Database Connection.
Sample Output
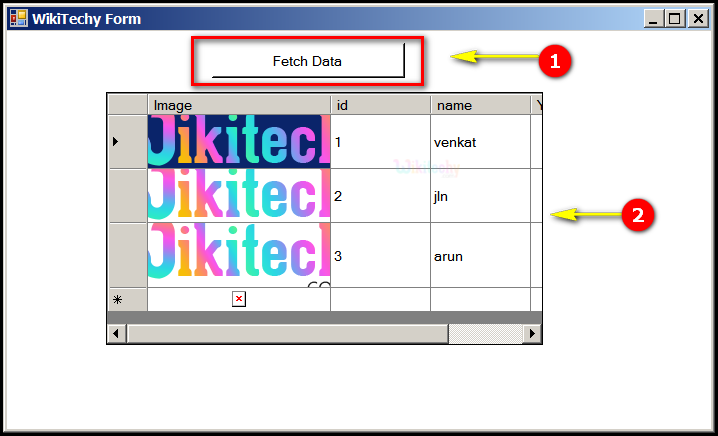
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the image "wikitechy.com" logo, id "1,2,3" and name "venkat, jln, arun".
Add image column programmatically in datagridview in c#
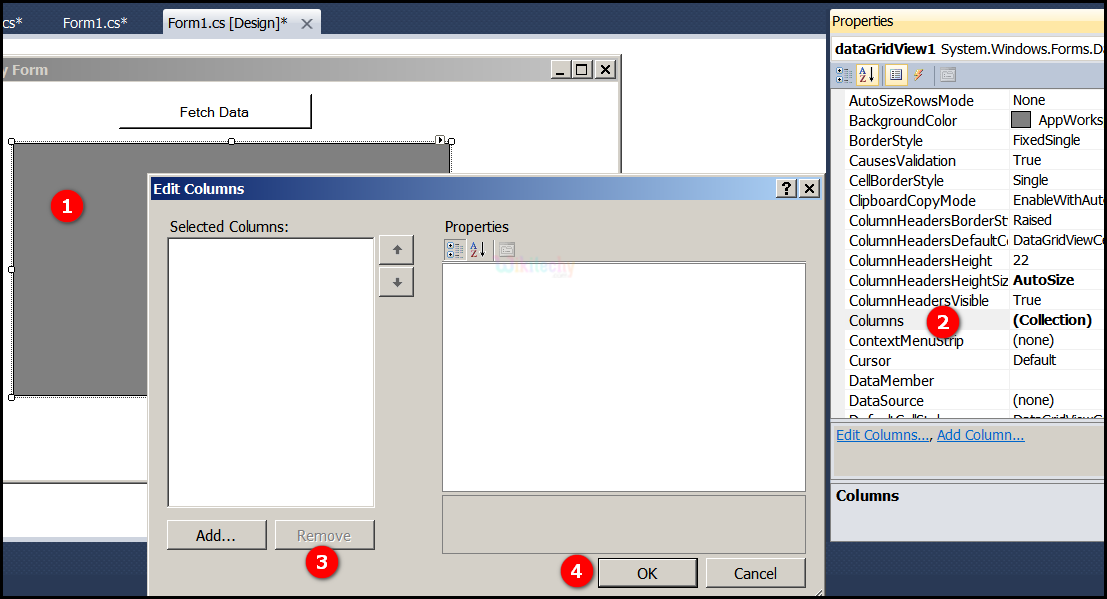
- Go to tool box and click on the DataGridview option the form will be open.
- Go to properties click on column collection pop up Edit Columns window will open after choose the image button.
- Click on "remove" button in Edit Columns window.
- After click on the ok button.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
using System.IO;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
string connectionString =@"Data Source=VENKAT\KAASHIV;
Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
DataGridViewImageColumn img = new DataGridViewImageColumn();
Image image = System.Drawing.Image.FromFile(@"E:\wikitechy\logo.png");
img.Image = image;
dataGridView1.Columns.Add(img);
img.HeaderText = "Image";
img.Name = "img";
}
}
}
{
Code Explannation
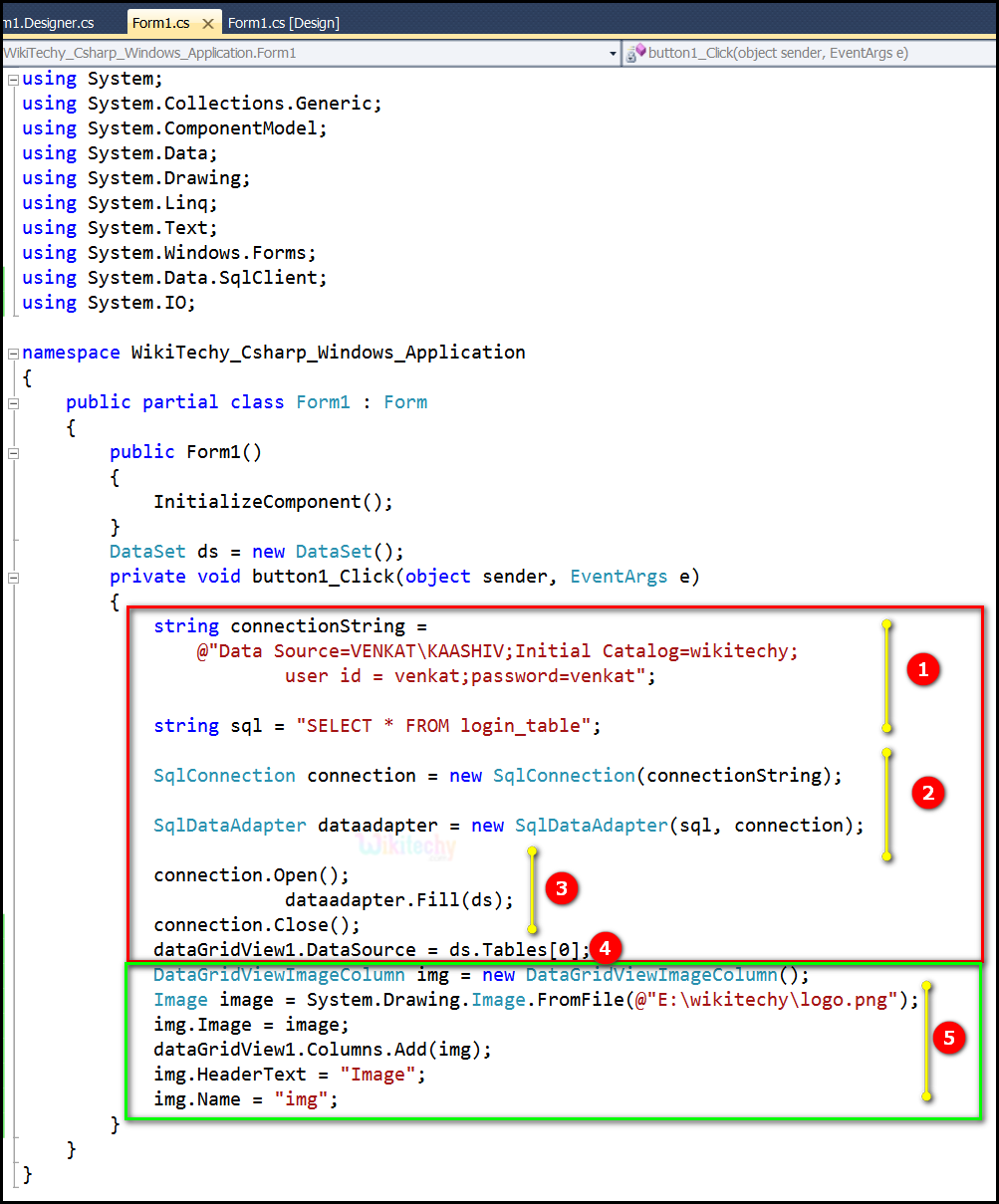
- string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;password=venkat"; connection string specifies that includes the source database name here the name is VENKAT\KAASHIV, and other parameters needed to establish the initial catalog connection is wikitechy. The default value is user id and password is venkat.In this example string sql = "SELECT * FROM login_table"; specifies to select the login_table in SQL server.SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource.
- SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection); specifies to initializes a new instance of the SqlDataAdapter class with a SelectCommand (sql) and a SqlConnection object(connection).
- connection.Open(); specifies to Open the SqlConnection.dataadapter.Fill(ds); specifies to fetches the data from User and fills in the DataSet ds.connection.Close(); it is used to close the Database Connection.
- dataGridView1.DataSource = ds.Tables[0]; is used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
- DataGridViewImageColumn img = new DataGridViewImage Column(); specifies to a new instance of the DataGridView ImageColumn.
- Image image = System.Drawing.Image.FromFile(@"E:\wikitechy\logo.png"); specifies to creates an image from the specified file. That file location is "E:\wikitechy\logo.png".Here dataGridView1.Columns.Add(img); specifies to add image in the column of a DataGridView , the column type contains cells of type DataGrideViewImageColumn.img.HeaderText = "Image"; used to store the image in DataTable.Here img.Name = "img"; specifies text series of Unicode name "img" .
Sample C# examples - Output :
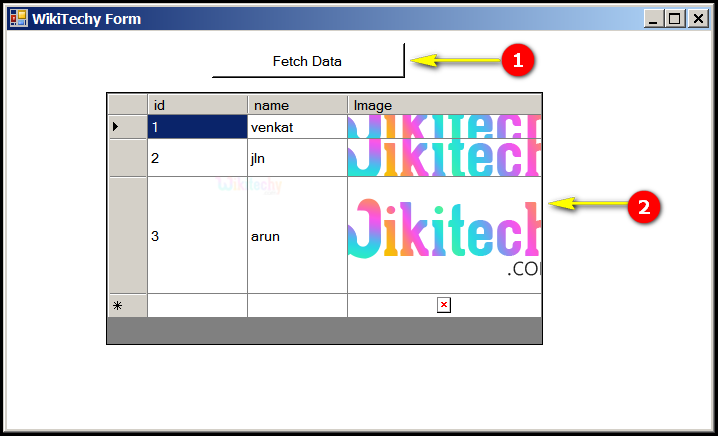
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the id "1,2,3", name "venkat, jln, arun" and Image "wikitechy.com".