C# String - c# - c# tutorial - c# net
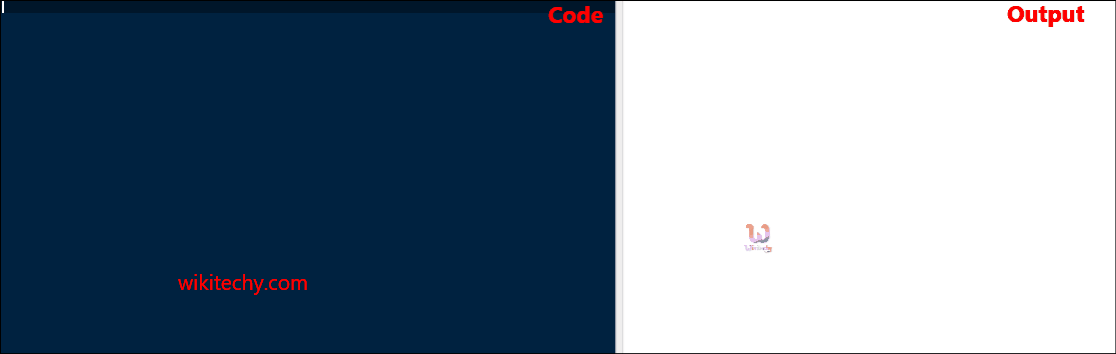
C# String
What is C# String ?
- In c#, a string is an object of type String whose value is text. Internally, the text is stored as a sequential read-only collection of Char objects.
- There is no null-terminating character at the end of a C# string; so, a C# string can contain any number of embedded null characters ('\0').
- The String is an immutable sequence of characters.
- He StringBuilder is a mutable sequence of characters.
- The string keyword is an alias for the System.String class.
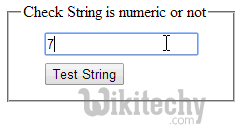
C# String
Create a string
- There are several ways to construct strings in C#:
- Create a string using a constructor.
- Create a string from a literal.
- Create a string using concatenation.
- Create a string using a property or a method.
- Create a string using formatting.
String Class Properties in C#:
- The String class has the following two types of string properties:
- Char
- Length
Char:
- Strings contain characters. These char values can be accessed with an indexer expression in the C# language.
- Gets the Char object at a specified position in the current String object.
- "Char Objects and Unicode Characters" section in the String class overview. In C#, the Chars property is an indexer.
Length:
- Gets the number of characters in the current String object.
String functions | Definitions |
---|---|
Clone() | Create a clone of string. |
Compareto() | Compareto() specifies to compare two strings and returns integer value as output. It returns 0 for true and 1 for false. |
Contains() | The C# contains() method checks whether specified character or string is existing or not in the string value. |
Endswith() | In strings the endswith() method checks whether specified to character is the last character of string or not. |
Equals() | The equals() method in C# compares two string and returns boolean value as output. |
Gethashcode() | Gethashcode() method returns hashvalue of specified string. |
Gettype() | Gettype() method returns the system.type of current instance. |
Gettypecode() | It returns the stystem.typecode for class system.string. |
Indexof() | Returns the index position of first occurrence of specified character. |
Tolower() | It converts string into lower case based on rules of the current principles. |
Toupper() | Toupper() method converts string into upper case based on rules of the current method. |
Insert() | Insert the string or character in the string at the specified position. |
Isnormalized() | This method checks whether this string is in unicode standardization form C. |
Lastindexof() | Returns the index position of last occurrence of specified character. |
Length | It is a string property that returns length of string. |
Remove() | This method deletes all the characters from beginning to specified index position. |
Replace() | This method replaces the character. |
Split() | This method splits the string based on specified value. |
Startswith() | It checks whether the first character of string is same as specified character. |
Substring() | This method returns substring. |
Tochararray() | Converts string into char array. |
Trim() | It removes extra whitespaces from beginning and ending of string. |
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_string
{
class Program
{
static void Main(string[] args)
{
string Name1 = "wikitechy";
string Name2 = "string";
string age = "22";
Console.WriteLine(" First Name: {0}", Name1);
Console.WriteLine(" Last Name: {0}", Name2);
Console.WriteLine(" Age: {0}", age);
Console.ReadKey();
}
Code Explanation:
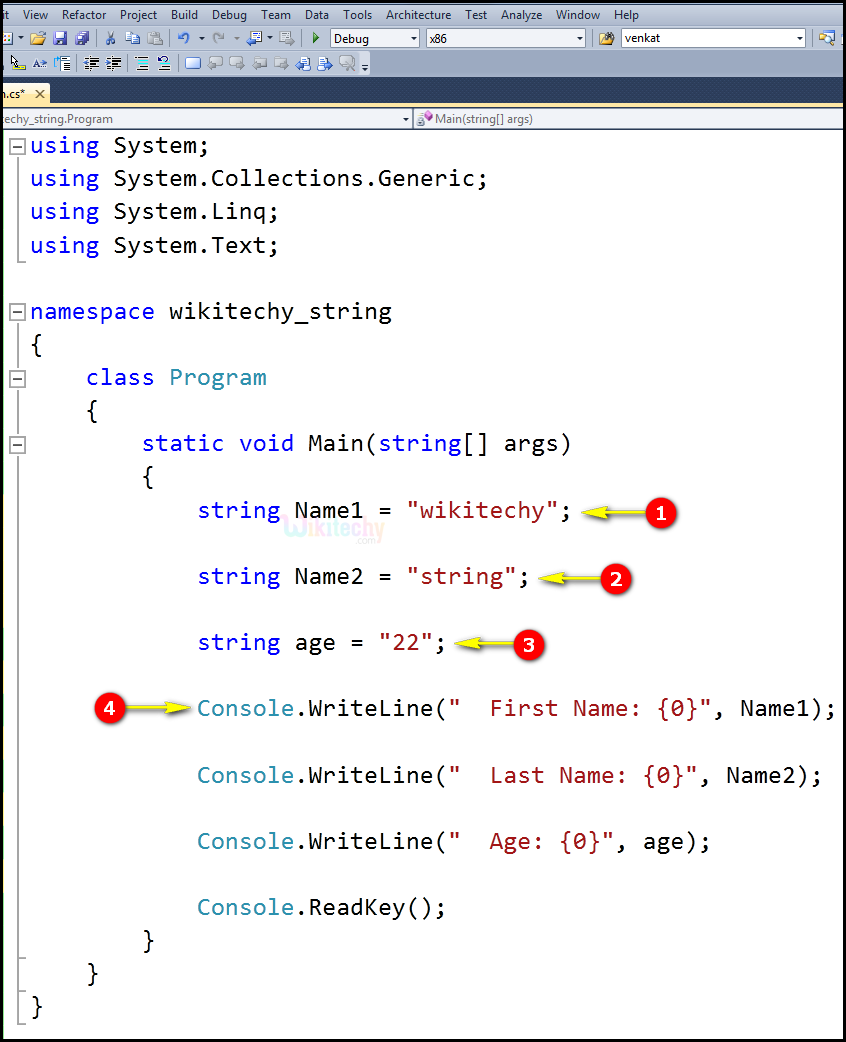
- It defines a string variable named Name1 and then assigns text value "wikitechy" to it.
- Here it defines a string variable named Name2 and then assigns text value "string" to it.
- Here it defines a string variable named age and then assigns text value "22" to it.
- In Console.WriteLine, the Main method specifies its behavior with the string statement "First Name, Last Name, Age:" assigned in the text values on the screen.
Sample C# examples - Output :
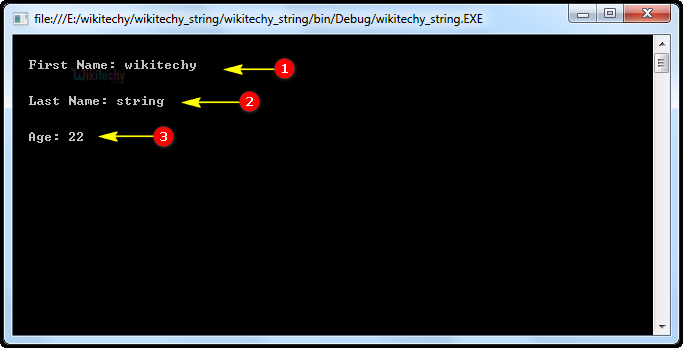
- Were in this output, we have shown the "first Name: wikitechy", which specifies to name1 variable is assigned to the wikitechy name.
- Here in this output, we have shown the "Last Name: string", which specifies to name2 variable is assigned to the string name.
- In this output, we have shown the "Age: 22" which specifies to age variable is assigned to the value of 22.
c# strings :
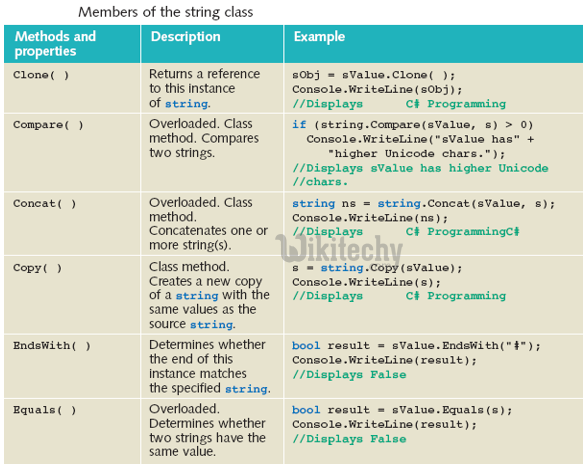
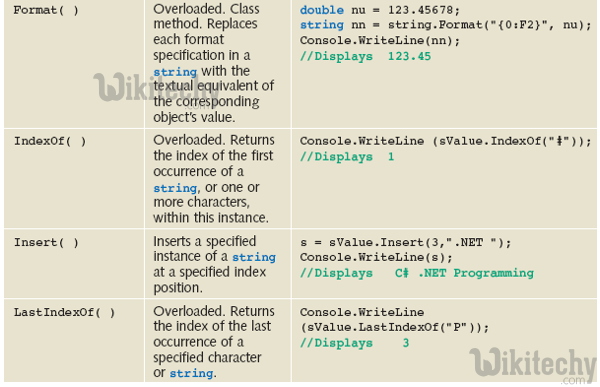
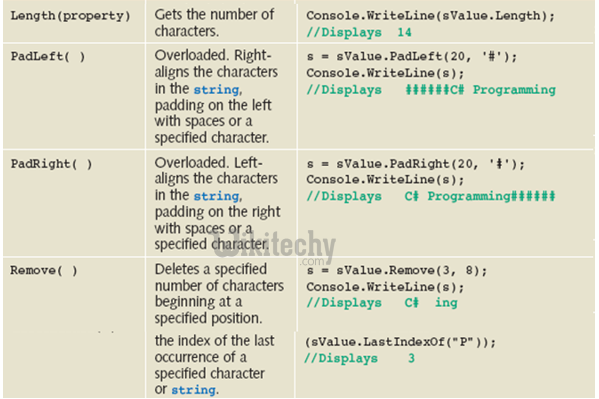
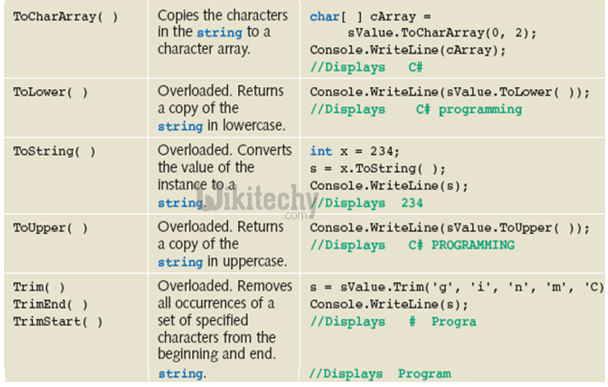
c# collections :
-
BitArray class
- Stores a collection of bit values represented as Booleans
-
HashTable class
- Stores a collection of key/value pairs that are organized based on the hash code of the key
-
Queue class
- Represents a FIFO (first in, first out) collection
-
Stack class
- Represents a simple LIFO (last in, first out) collection