C# Data Types - c# - c# tutorial - c# net
What is data type in C# and its types ?
- In data types, a programming language describes that what type of data a variable can hold. C# is a strongly typed language, therefore every variable and object must have a declared type. The C# type system contains three Type categories. They are
- Value Types,
- Reference Types and
- Pointer Types.
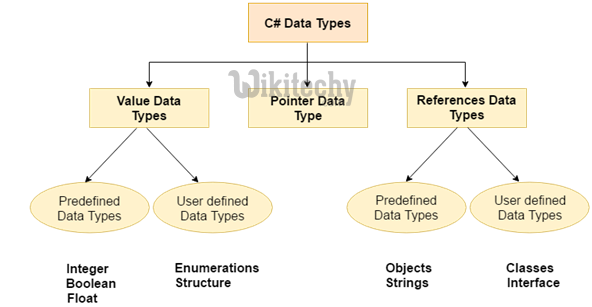
- In C#, it is possible to convert a value of one type into a value of another type.
Syntax
DataType VariableName
Syntax Explanation
- Here DataType is specifying to type of data that the variable can hold.
- In VariableName, the variable we declare for hold the values.
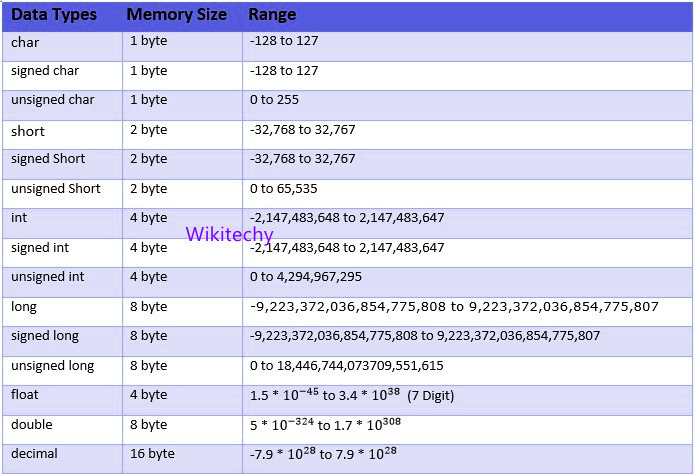
Value Types:
- Value type variables can be assigned to value directly. They arederived from the class System.ValueType.
- The value types directly contain data.
- These are some examples int, char, and float.
- int data typesstores numbers, char data type stores alphabets, and floating point numbers, respectively. When we declare an int type, the system allocates memory to store the value.
c# value type vs reference type :
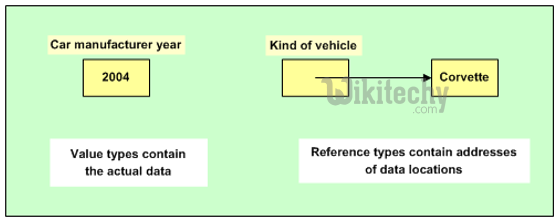
Read Also
dot net training institute near me , summer internship with stipend , .net course online free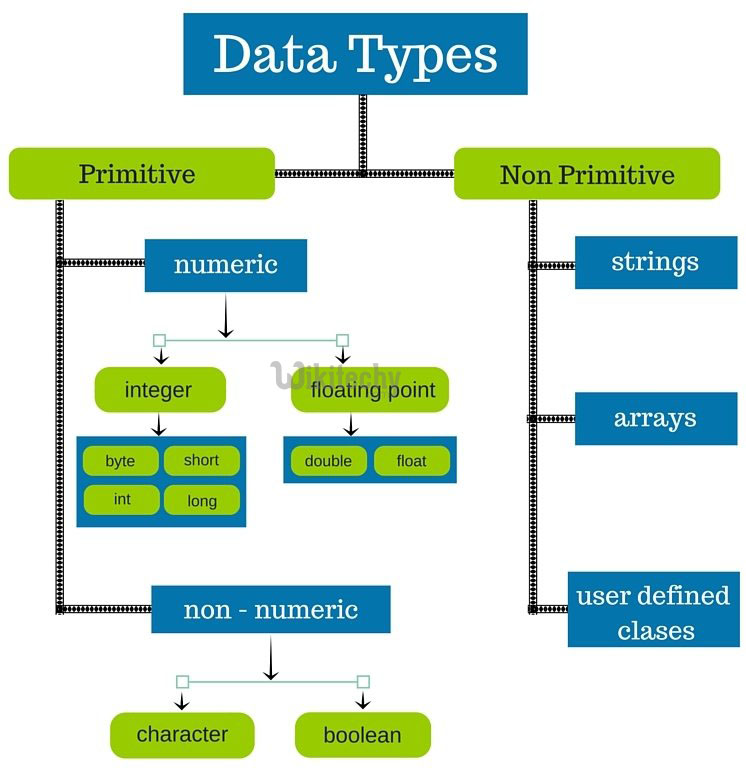
Int datatype:
- Integers are a subset of the real numbers.
- int variables are stored signed 32-bit integer values in the range of -2,147,483,648 to +2,147,483,647
Syntax:
int count;
Float Datatype:
- Floating point numbers represent real numbers.
- Real numbers measure continuous quantities, like weight, height, or speed.
- In C# we have three floating point types: float, double, and decimal.
Syntax:
float count;
click below button to copy the code. By - c# tutorial - team
Reference Type
- The reference types do not contain the actual data stored in a variable, but they contain a reference to the variables.
- In other words, they refer to a memory location. Using multiple variables, the reference types can refer to a memory location.
- If the data in the memory location is changed by one of the variables, the other variable automatically reflects this change in value.
- Example of built-in reference types are: object, dynamic, and string.
Char Datatype:
- The char keyword is used to declare an instance of the System.Char structure that the .NET Framework uses to represent a Unicode character.
- The char rage is 16-bit numeric (ordinal) value.
c# type conversion :
-
The conversion is happening automatically no need to specify,
- Changes int data type into a double
- No implicit conversion from double to int
int value1 = 0,
anotherNumber = 75;
double value2 = 100.99,
anotherDouble = 100;
value1 = (int) value2; // value1 = 100
value2 = (double) anotherNumber; // value2 = 75.0
c# format specifiers :
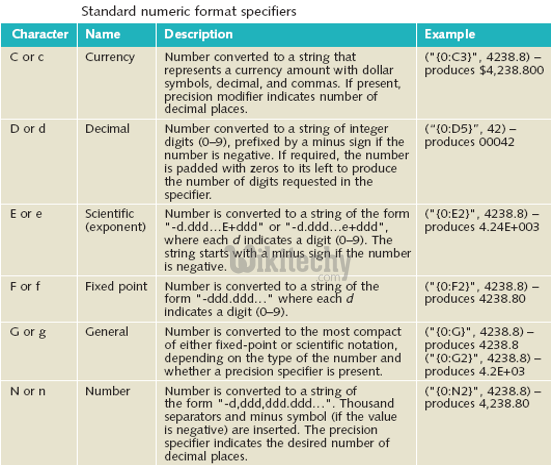

c# Custom Numeric Format Specifiers :
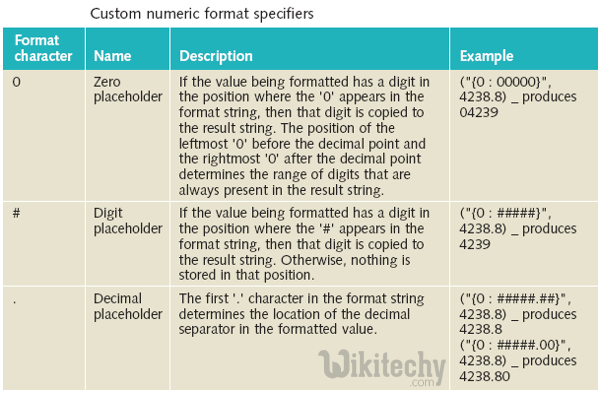
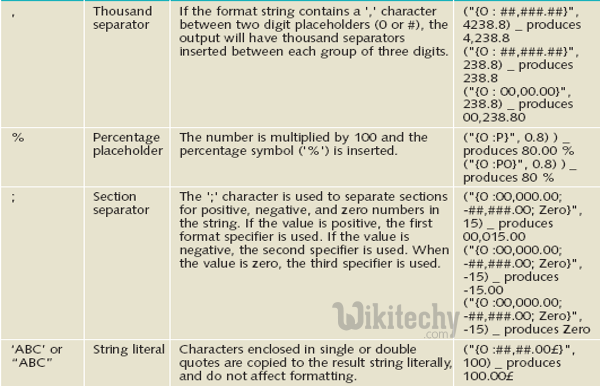
c# Formatting Output :
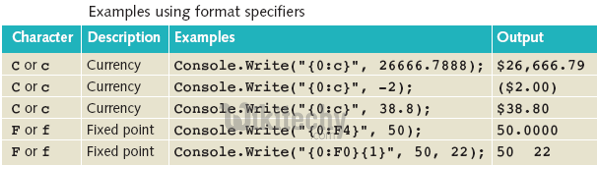