C# List - c# - c# tutorial - c# net
What is C# list ?
- C# List<T> class is used to store and fetch elements.
- It can have duplicate elements. It is found in System.Collections.Generic namespace.
- The List<T> collection is the same as an ArrayList except that List<T> is a generic collection whereas ArrayList is a non-generic collection.
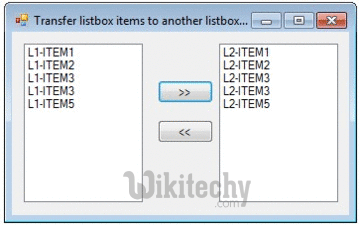
List
Syntax:
[SerializableAttribute]
public class List<T> : IList<T>, ICollection<T>, IEnumerable<T>,
IEnumerable, IList, ICollection, IReadOnlyList<T>, IReadOnlyCollection<T>
- List<T> includes more helper methods than IList<T> interface. The table shown below lists important properties and methods of List<T>, which are initialized using a List<T>:
Property | Usage |
---|---|
Items | Gets or sets the element at the specified index |
Count | Returns the total number of elements exists in the List<T> |
Method | Usage |
---|---|
Add | Adds an element at the end of a List<T>. |
AddRange | Adds elements of the specified collection at the end of a List<T>. |
BinarySearch | Search the element and returns an index of the element. |
Clear | Removes all the elements from a List<T>. |
Contains | Checks whether the speciied element exists or not in a List<T>. |
Find | Finds the first element based on the specified predicate function. |
Foreach | Iterates through a List<T>. |
Insert | Inserts an element at the specified index in a List<T>. |
InsertRange | Inserts elements of another collection at the specified index. |
Remove | Removes the first occurence of the specified element. |
RemoveAt | Removes the element at the specified index. |
RemoveRange | Removes all the elements that match with the supplied predicate function. |
Sort | Sorts all the elements. |
TrimExcess | Sets the capacity to the actual number of elements. |
TrueForAll | Determines whether every element in theĆ List<T> matches the conditions defined by the specified predicate. |
C# List example:
- Let's see an example of generic List<T> class that stores elements using Add() method and iterates the list using for-each loop.
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
// Create a list of strings
var names = new List<string>();
names.Add("Sonoo Jaiswal");
names.Add("Ankit");
names.Add("Peter");
names.Add("Irfan");
// Iterate list element using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Sonoo Jaiswal
Ankit
Peter
Irfan
C# List<T> example using collection initializer:
using System;
using System.Collections.Generic;
public class ListExample
{
public static void Main(string[] args)
{
// Create a list of strings using collection initializer
var names = new List<string>() {"Sonoo", "Vimal", "Ratan", "Love" };
// Iterate through the list.
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Sonoo
Vimal
Ratan
Love
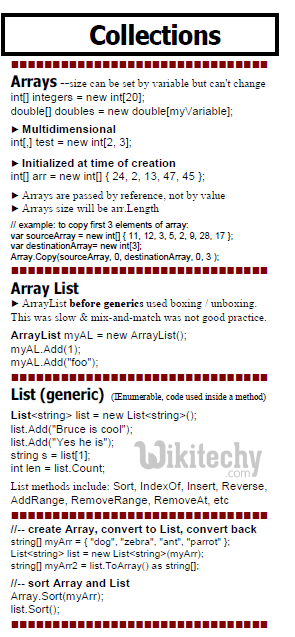
c# collections
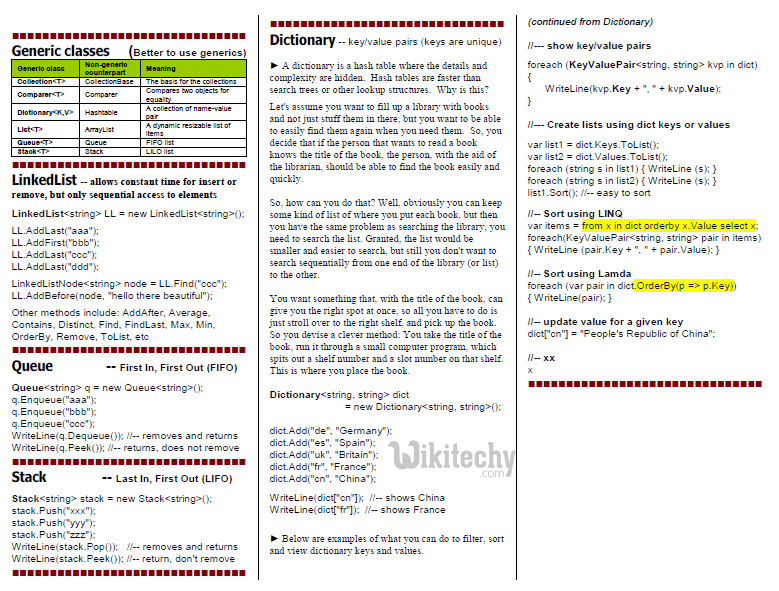
csharp collections details