C# Progress Bar - c# - c# tutorial - c# net
What is C# Progress Bar ?
- In C#, a progress bar is a control that an application can use to specify the progress of a lengthy operation such as calculating a complex result, downloading a large file from the Web etc.
- The progress control can be used for the "Loading" process in many other cases. It is used in WinForms.ProgressBar controls are used, once an operation takes more than a short period of time. The Maximum and Minimum properties are defining the range of values to represent the progress of a task.
- Minimum: It sets the lower value for the range of valid values for progress.
- Maximum: Maximum value sets the upper value for the range of valid values for progress.
- Value: This property gets or sets the current level of progress.

C# Progress Bar
Syntax:
ProgressBar pBar = new ProgressBar();
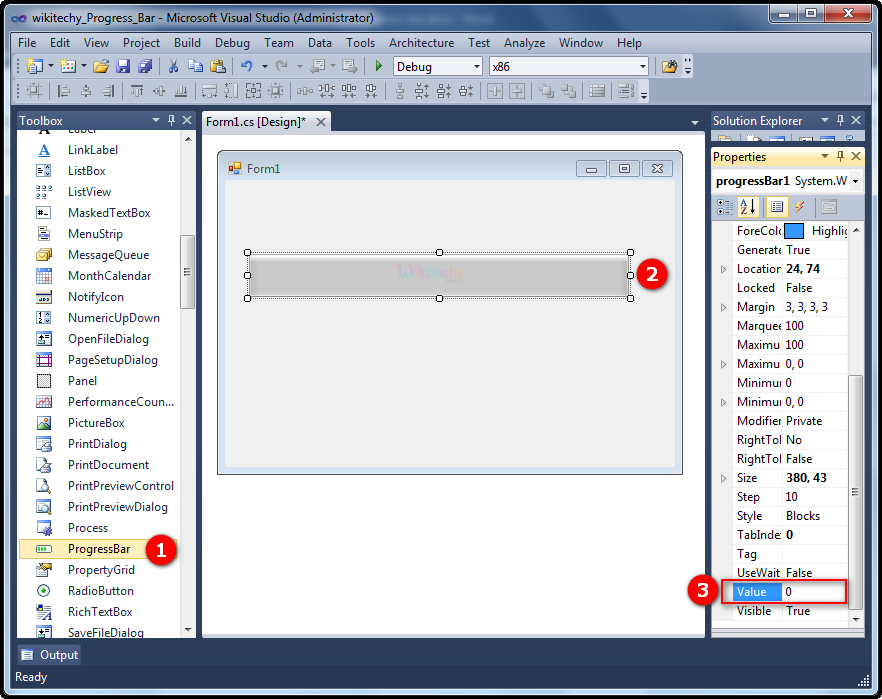
- Go to tool box and select the ProgressBar option.
- After dragging and dropping a ProgressBar component from Toolbox to a Form.
- After that, right click on ProgressBar select Properties menu to set a value "0".
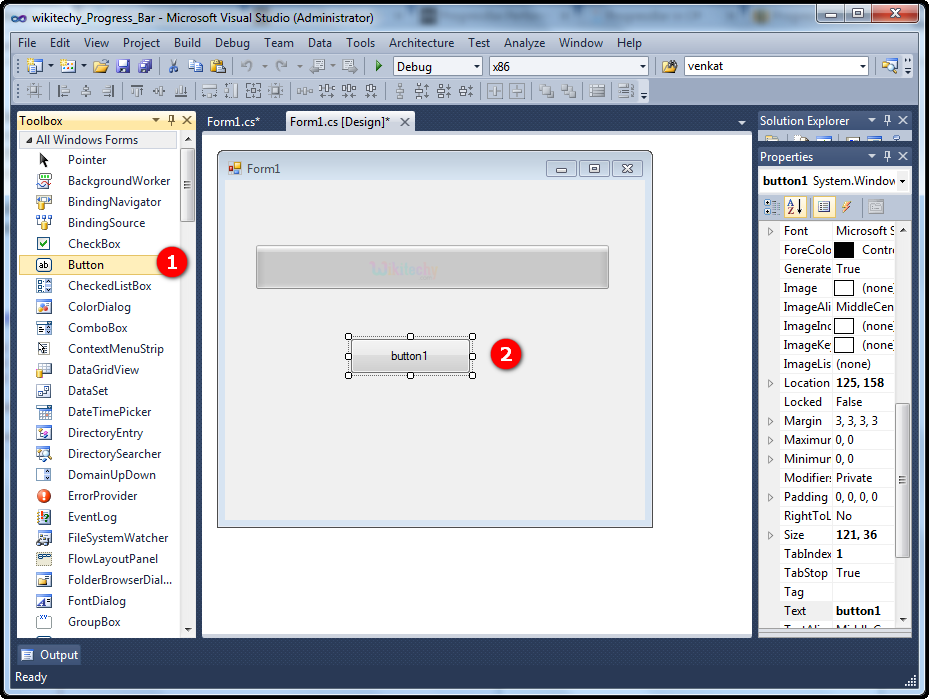
- Go to tool box and select the Button option.
- After dragging and dropping a Button component from Toolbox to a Form.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace wikitechy_Progress_Bar
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int a;
progressBar1.Minimum = 0;
progressBar1.Maximum = 300;
for (a = 0; a <= 300; a++)
{
progressBar1.Value = a;
}
}
}
}
Code Explanation
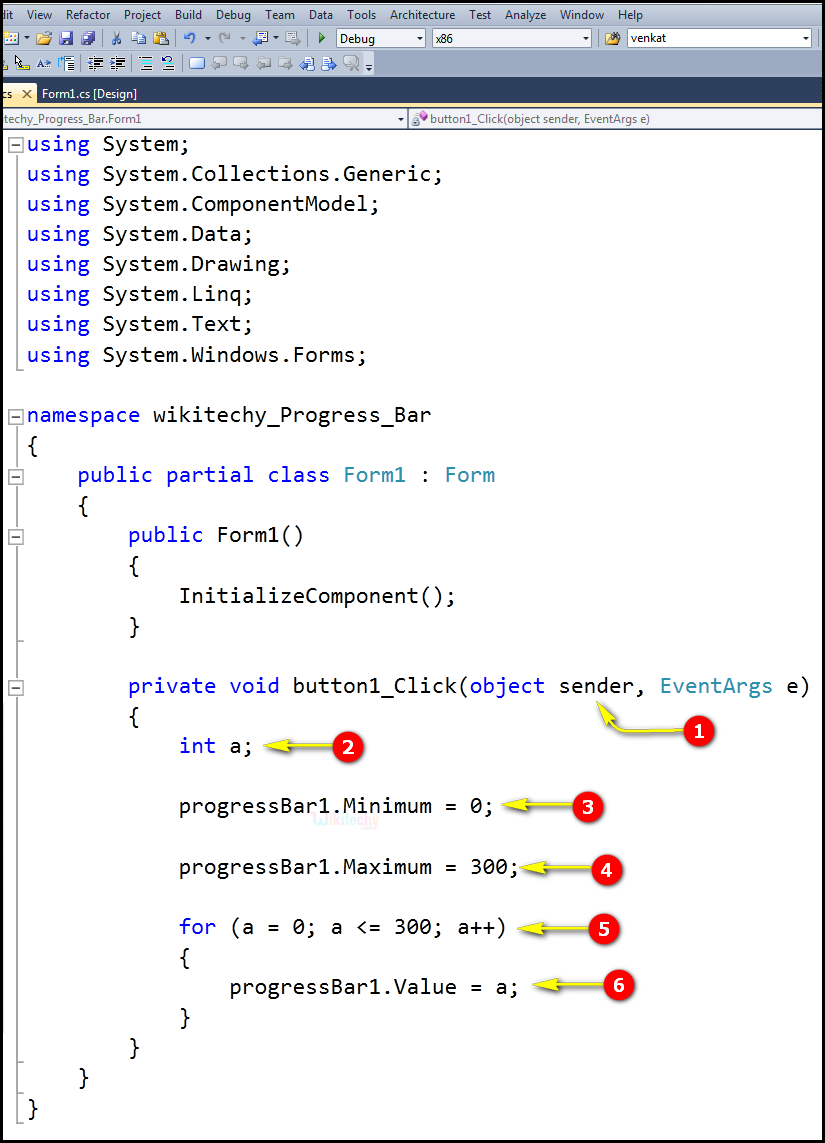
- Here private void button1_Click(object sender, EventArgs e) click event handler for button1 specifies, which is a nomal Button control in Windows Forms. The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button1_Click(object sender, EventArgs e).
- Here we declare the integer variables a.
- progressBar1.Minimum = 0; Minimum are set to 0. The ProgressBar fills in from the left to the right.
- progressBar1.Maximum = 300; the Minimum properties define the range of a ProgressBar1. The Value property represents the current value of a ProgressBar1 value as 300.
- Here for (a = 0; a <= 300; a++) int a = 5 is an integer variable that initial value as 0. which means the expression, (a<= 300) less than or equal to (0<=300) condition is true. and a++ gets incremented by 1 and becomes less than or equal to 300.
- progressBar1.Value = a; the current value (300) of the ProgressBar for the minimum value is specifies to color green, it display the progress of the control. Here we can also use the Value property to display the progress of an operation.
Sample Output
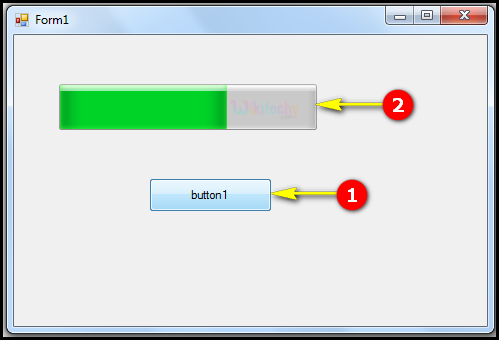
- Here in this output the button1 will display the data values by clicking it.
- After click on button1, the progress of a ProgressBar is moves from left to right. Here we are using the minimum and maximum values set to 0 and 300. The ProgressBar fully fills from left to right as shown below.
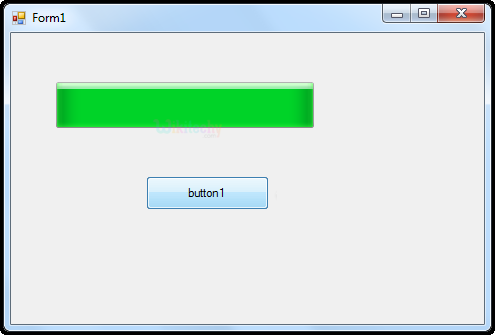