C# Queue - c# - c# tutorial - c# net
What is Queue in C# ?
- C# Queue<T> class is used to Enqueue and Dequeue elements.
- It uses the concept of Queue that arranges elements in FIFO (First In First Out) order.
- It can have duplicate elements.
- It is found in System.Collections.Generic namespace.
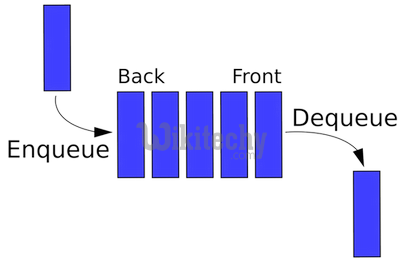
Constructors:
- Queue<T> Constructor - Initializes a new instance of the Queue<T> class that is empty. The next lines shows how we create the empty queue.
Queue<string> queue = new Queue<string>();
- Queue<T> Constructor (IEnumerable<T>) - Initializes a new instance of the Queue<T>class that contains elements copied from the specified collection.
string[] courses = { "MCA","MBA", "BCA","BBA", "BTech","MTech" };
Queue<string> queue = new Queue<string>(courses);
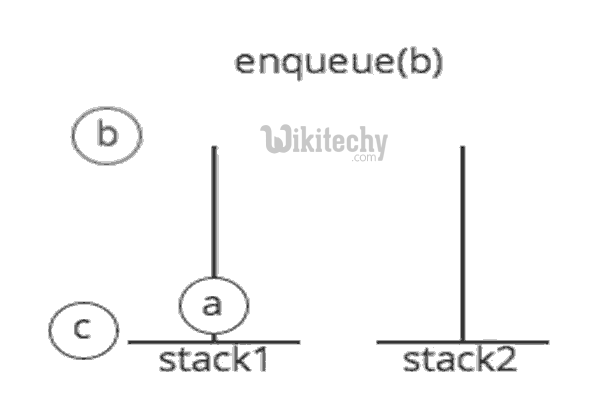
Queue
- Queue<T> Constructor (Int32) - Initializes a new instance of the Queue<T> class that is empty and has the specified initial capacity.
Queue<string> queue = new Queue<string>(4);
C# Queue<T> example:
- Let's see an example of generic Queue<T> class that stores elements using Enqueue() method, removes elements using Dequeue() method and iterates elements using for-each loop.
using System;
using System.Collections.Generic;
public class QueueExample
{
public static void Main(string[] args)
{
Queue<string> names = new Queue<string>();
names.Enqueue("Sonoo");
names.Enqueue("Peter");
names.Enqueue("James");
names.Enqueue("Ratan");
names.Enqueue("Irfan");
foreach (string name in names)
{
Console.WriteLine(name);
}
Console.WriteLine("Peek element: "+names.Peek());
Console.WriteLine("Dequeue: "+ names.Dequeue());
Console.WriteLine("After Dequeue, Peek element: " + names.Peek());
}
}
C# examples - Output :
Sonoo
Peter
James
Ratan
Irfan
Peek element: Sonoo
Dequeue: Sonoo
After Dequeue, Peek element: Peter