C# Serializable | C# Serialization - c# - c# tutorial - c# net
What is C# Serialization ?
- Serialization is the process of converting an object into a stream of bytes in order to store the object or transmit it to memory, a database, or a file.
- Its main purpose is to save the state of an object in order to be able to recreate it when needed. The reverse process is called deserialization.
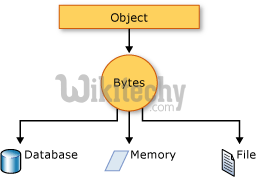
How Serialization Works:
- The object is serialized to a stream, which carries not just the data, but information about the object's type, such as its version, culture, and assembly name.
- From that stream, it can be stored in a database, a file, or memory.
C# Serializable Attribute:
- To serialize the object, you need to apply SerializableAttribute attribute to the type.
- If you don't apply SerializableAttribute attribute to the type, SerializationException exception is thrown at runtime.
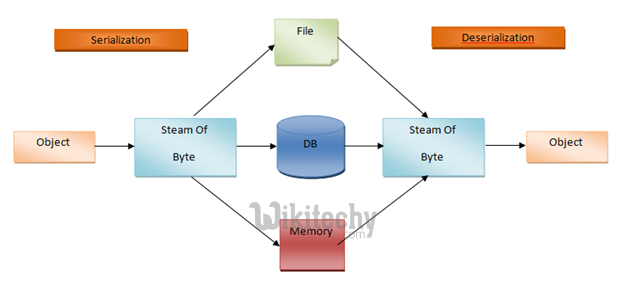
Serialization Deserialization Language
C# Serialization example:
- Let's see the simple example of serialization in C# where we are serializing the object of Student class.
- Here, we are going to use BinaryFormatter.Serialize(stream, reference) method to serialize the object.
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[Serializable]
class Student
{
int rollno;
string name;
public Student(int rollno, string name)
{
this.rollno = rollno;
this.name = name;
}
}
public class SerializeExample
{
public static void Main(string[] args)
{
FileStream stream = new FileStream("e:\\sss.txt", FileMode.OpenOrCreate);
BinaryFormatter formatter=new BinaryFormatter();
Student s = new Student(101, "sonoo");
formatter.Serialize(stream, s);
stream.Close();
}
}
sss.txt:
JConsoleApplication1, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null Student rollnoname e sonoo
- As you can see, the serialized data is stored in the file. To get the data, you need to perform deserialization.