C# Methods - c# - c# tutorial - c# net
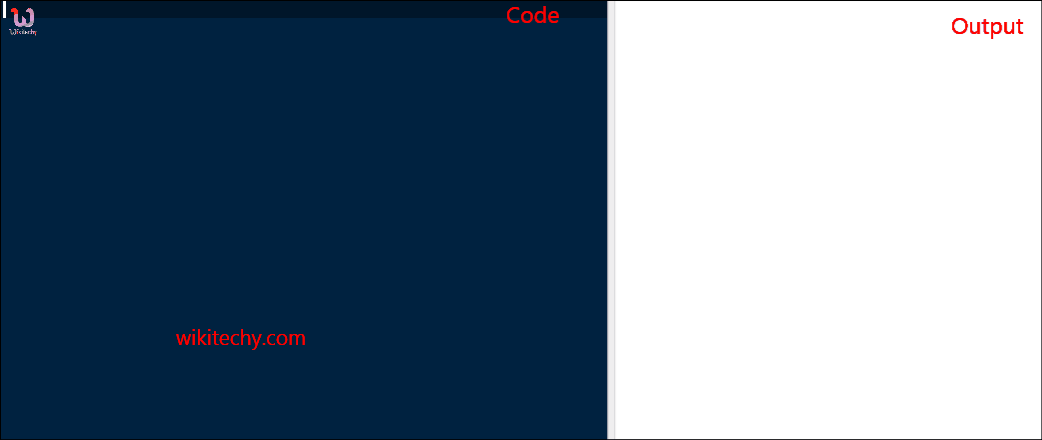
C# Methods
What are the Methods in C# ?
- A method is a code block containing a series of statements. Methods must be declared within a class or a structure. It is a good programming practice that methods do only one specific task. In C#, every program has at least one class with a method named Main.
- Define the method
- Call the method
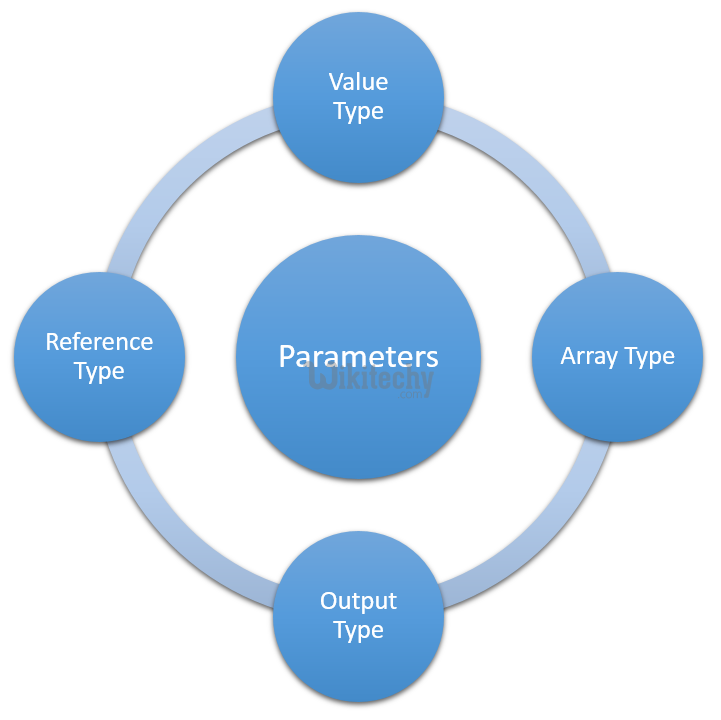
Defining Methods
- When we define a method, we essentially declare the elements of its structure. The syntax for defining a method in C# is as follows:
- Define the method
- Call the method
Syntax
< Access Specifier> < Return Type> < Method Name> (Parameter list)
{
Body
}
Syntax Explanation:
- Access Specifier: access specifier determines the visibility of a variable or a method from another class.
- Return type: A method can return a value. Here the return type is the data type of the value the method returns. If the method is not returning any values, then the return type is void.
- Method name: Method name is a unique identifier and it is a case sensitive. Method name cannot be same as any other identifier declared in the class.
- Parameter List: Enclosed between parentheses, the parameters are used to pass and receive data from a method. The parameter list refers to the type, order, and number of the parameters of a method.
- Method body: method body contains the set of instructions its needed to complete the required activity.
Call the method:
- In c#, after creating a function, we need to call Main() method to execute.
- In order to call method, we need to create an object of containing class, then followed by dot(.) operator we can call the method.
- If method is static, then there is no need to create an object and we can directly call it followed by class name.
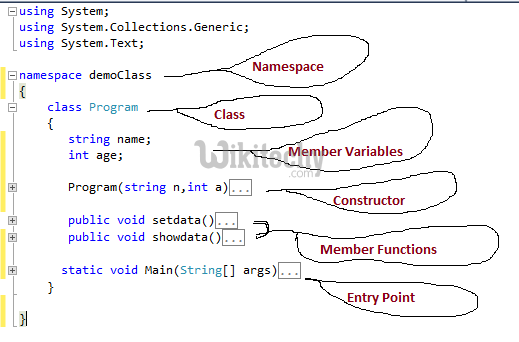
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_Method
{
class print
{
public static void wikitechy()
{
Console.WriteLine("www.wikitechy.com");
Console.ReadLine();
}
}
class Program
{
static void Main(string[] args)
{
print.wikitechy();
}
}
}
Read Also
.net developer internship , .net training institute near me , dotnet core 5 , dotnet training institute in chennaiCode Explanation:
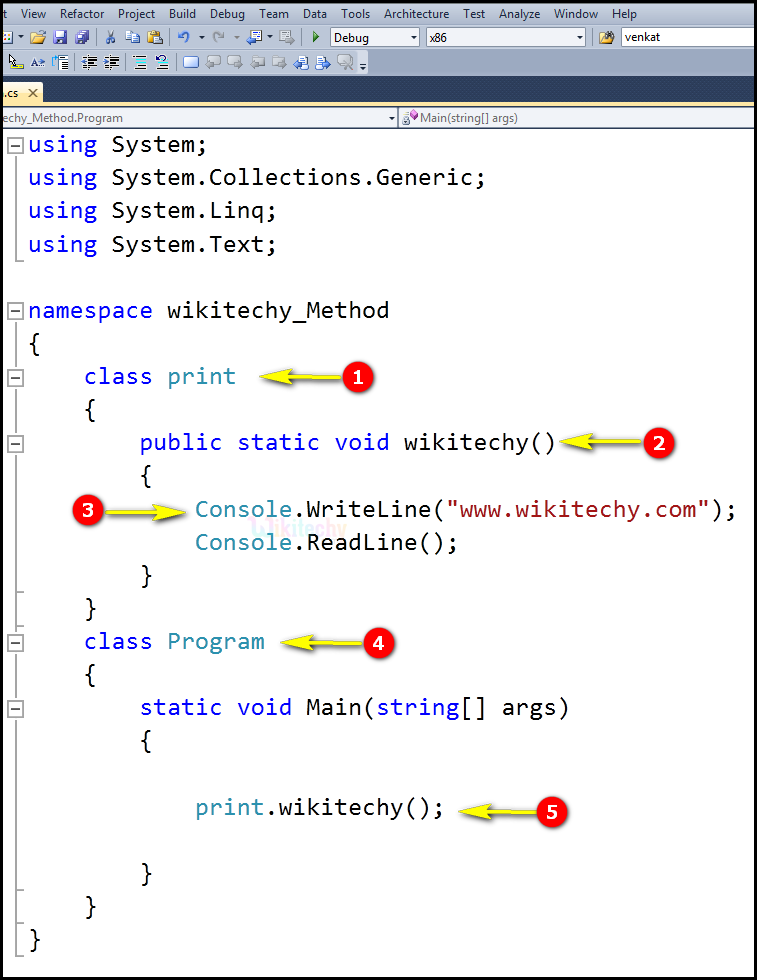
- class print is specifying to class name of print.
- These specifies to main method call directly static method with class name "wikitechy". And public member can be called "wikitechy" from external locations.
- Here we print the class of "wikitechy" using the www.wikitechy.com Console WriteLine statement.
- class program is specifying to another class name of program.
- Here in this example print class call the main method directly from static method with the class "wikitechy".
Sample C# examples - Output :
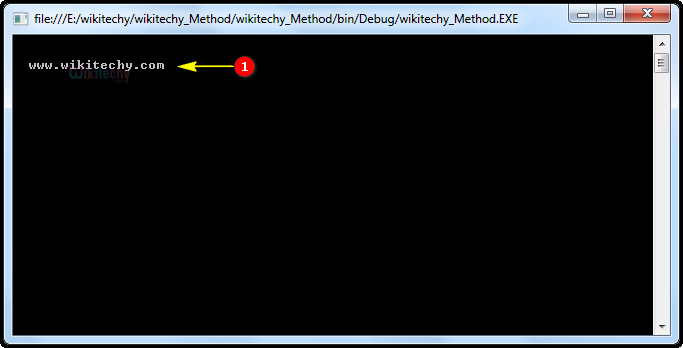
- Here in this output we display the www.wikitechy.com which specifies to console statement.