C# Null | C# Nullable - c# - c# tutorial - c# net
What is Nullable type in C# ?
- A value type variable cannot be null.
- A nullable type can represent the correct range of values for its underlying value type, plus an additional null value.
- Nullable types can represent all the values of an underlying type, and an additional null value.
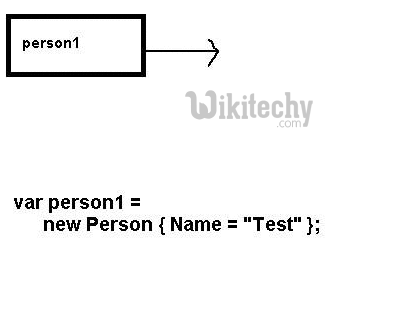
Nullable in csharp
Syntax:
<data_type> ? <variable_name> = null;
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Wikitechy_naullable
{
class Program
{
static void Main(string[] args)
{
int? a = null;
int? b = 10;
double? c = new double?();
double? d = 2.2254;
Console.WriteLine("wikitechy says the Nullables values are: {0}, {1},
{2}, {3}", a, b, c, d);
Console.ReadLine();
}
}
}
Code Explanation:
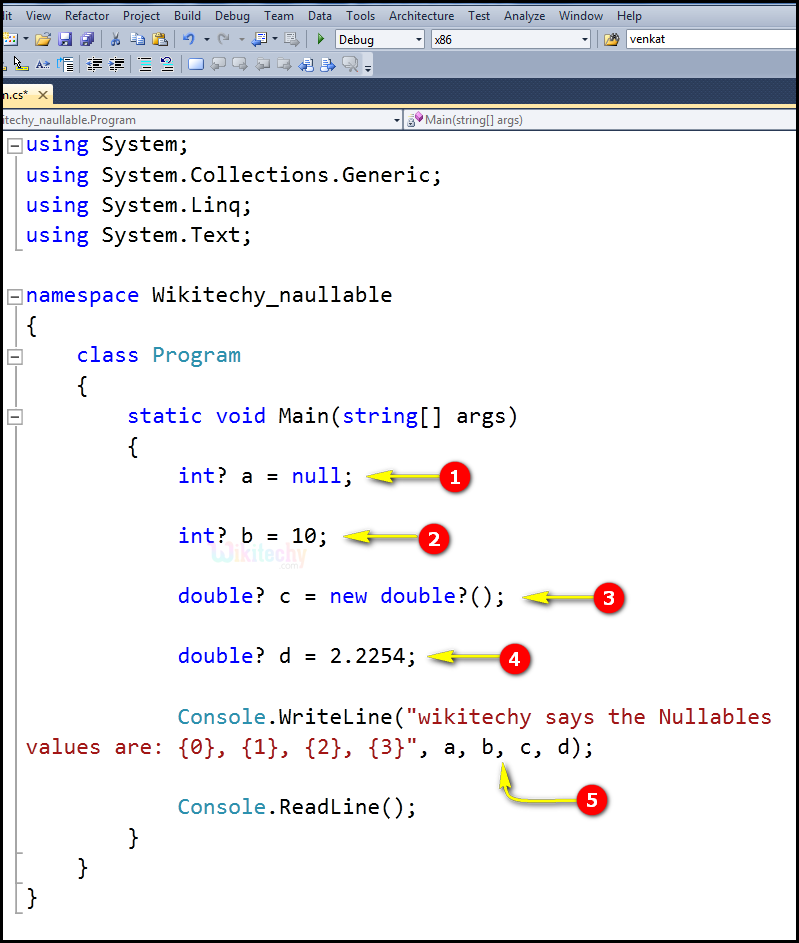
- Integer type can also be assigned the value a null : int? a = null;
- Integer type can also be b assigned the value 10 null: int? b = 10;
- It is used to store large and small values. It is used to new double type that assigned to the c variable
- Double type can also be d assigned the value double? d = 2.2254; null: double? d = 2.2254;
- In Console.WriteLine, the Main method specifies its behavior with the statement "wikitechy says the Nullables values are:". to be displayed on the screen.
Sample C# examples - Output :
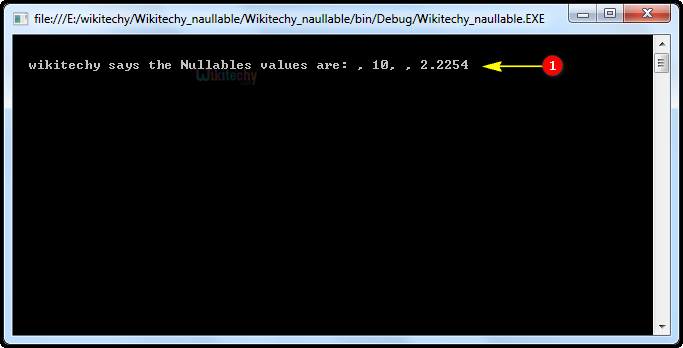
- Here in this output we display the "wikitechy says the Nullables values are: , 10, , 2.2254" which specifies to integer variable a "null", integer b variable value as 10, new double value and double variable d value as 2.2254.