C# Controls Datagridview Button Column | Button Column In Datagridview C# - c# - c# tutorial - c# net
What is Datagridview ?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
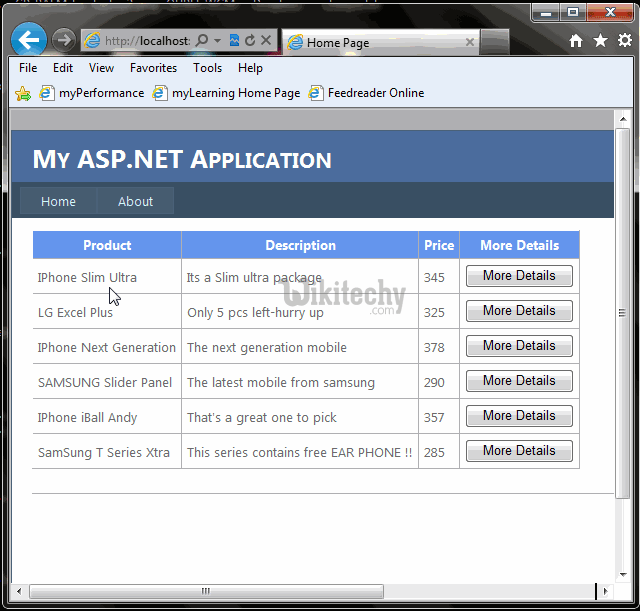
Gridview Button Column
Button Column:
- In c#, a column types for the DataGrid control that contains a user-defined button.
- Hosts a collection of DataGridViewButtonCell objects
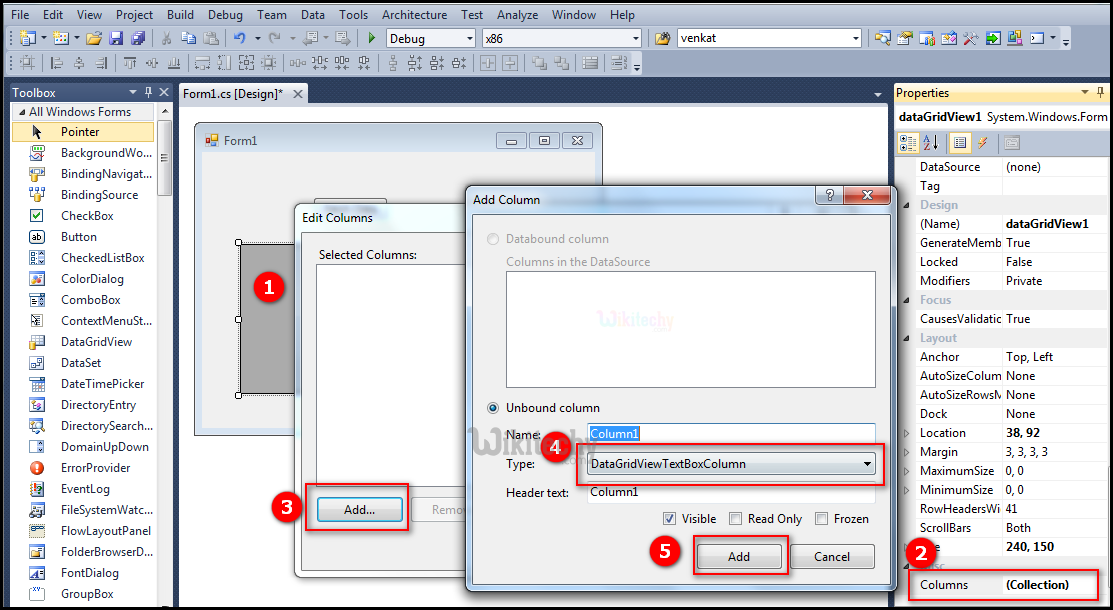
- Go to tool box and click on the DataGridview option the form will be open.
- When we click on the Collections, the String Collection Editor window will pop up where we can type strings. column collection is representing a collection of DataColumn objects for a DataTable.
- Click on "Add" button another Add column windows form will be open.
- After click on text box, and then select the DataGride ViewTextBoxColumn.
- And then click on the "Add" button.
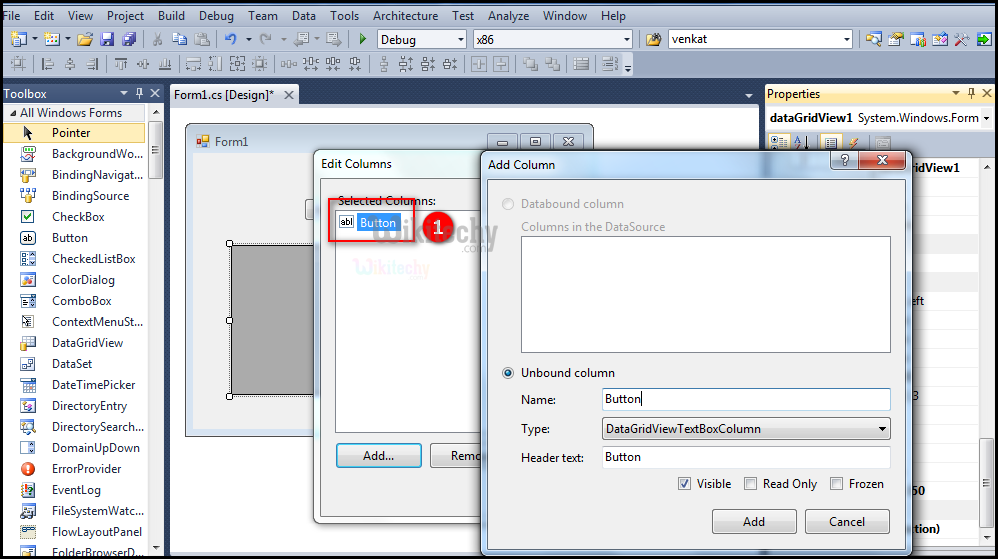
- After click on the "Add" button, "Button" name is adding in Selected Columns windows form.
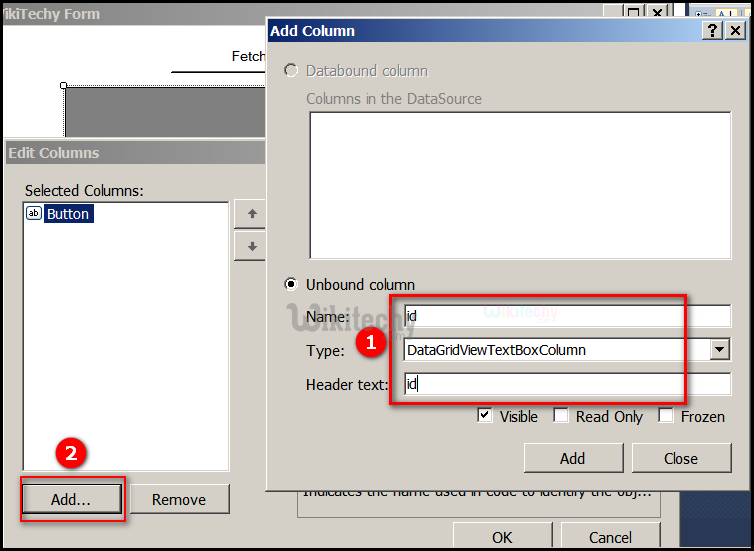
- Next adding id in the Name text box, click on type box and then select the DataGrideViewTextBoxColumn in type text. After add id in the Header text box.
- Click on the "Add" button, "id" name is adding in Selected Columns windows form.

- Next adding name in the Name text box, click on type box and then select the DataGrideViewTextBoxColumn in type text. After add name in the Header text box.
- Click on the "Add" button, "name" name is adding in Selected Columns windows form.
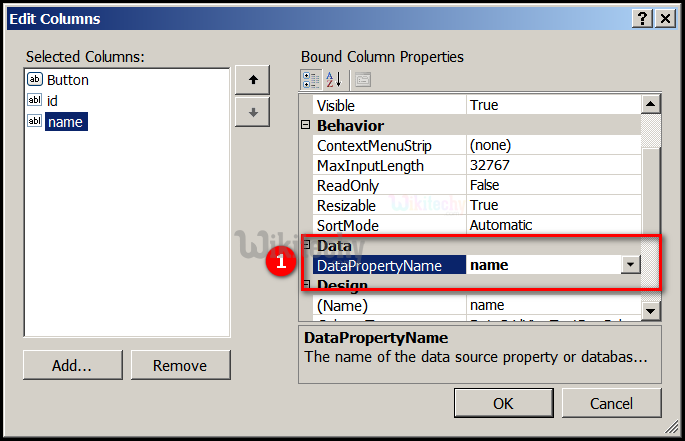
- In above diagram, the DataPropertyName is specifies to name of the property or database column associated with the DataGridViewColumn. Here we are giving name, this name is added in the Selected Columns.
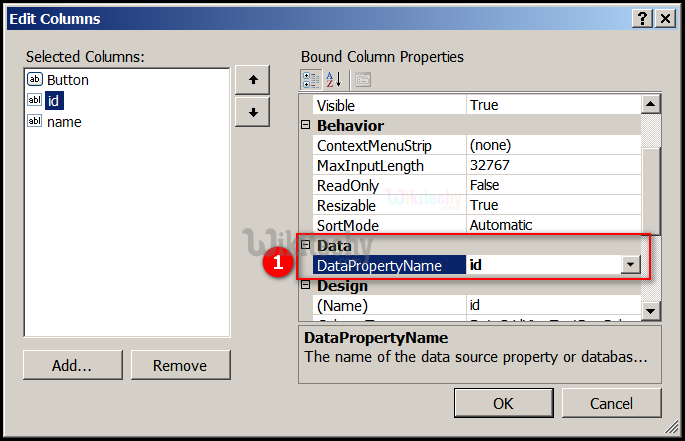
- In above diagram, the DataPropertyName (name of the property or database column associated with the DataGridViewColumn.) Here we are giving id, this id is added in the Selected Columns. Put id name in DataPropertyName and then click on "ok" button.
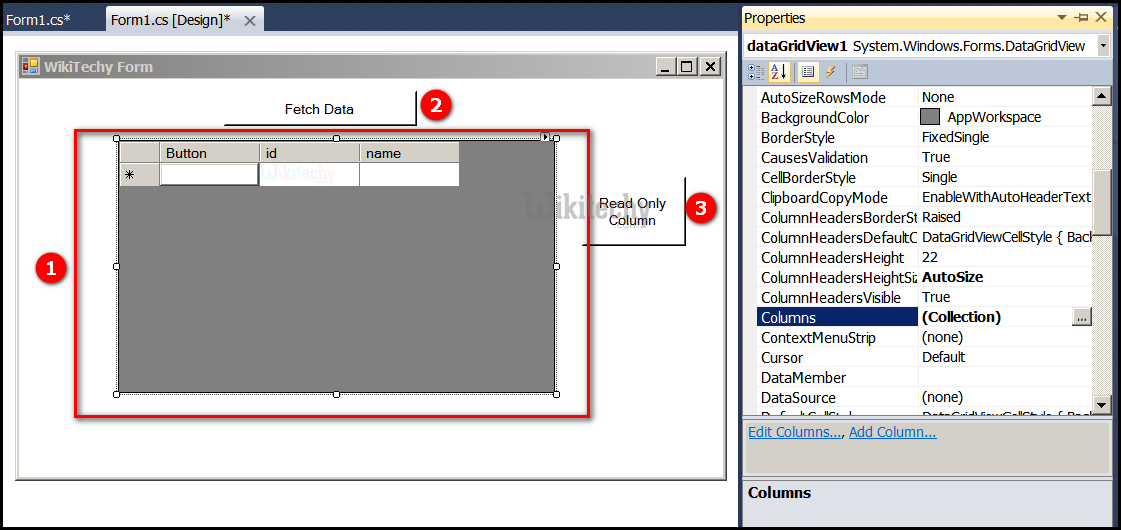
- After click on the ok button "Button", id and name table is opened.
- Here Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the button option and put the name as Read Only Column in the button.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 0)
{
MessageBox.Show((e.RowIndex + 1)+" Row "+(e.ColumnIndex + 1)+
" Column button clicked ");
}
}
}
}
Code Explanation:
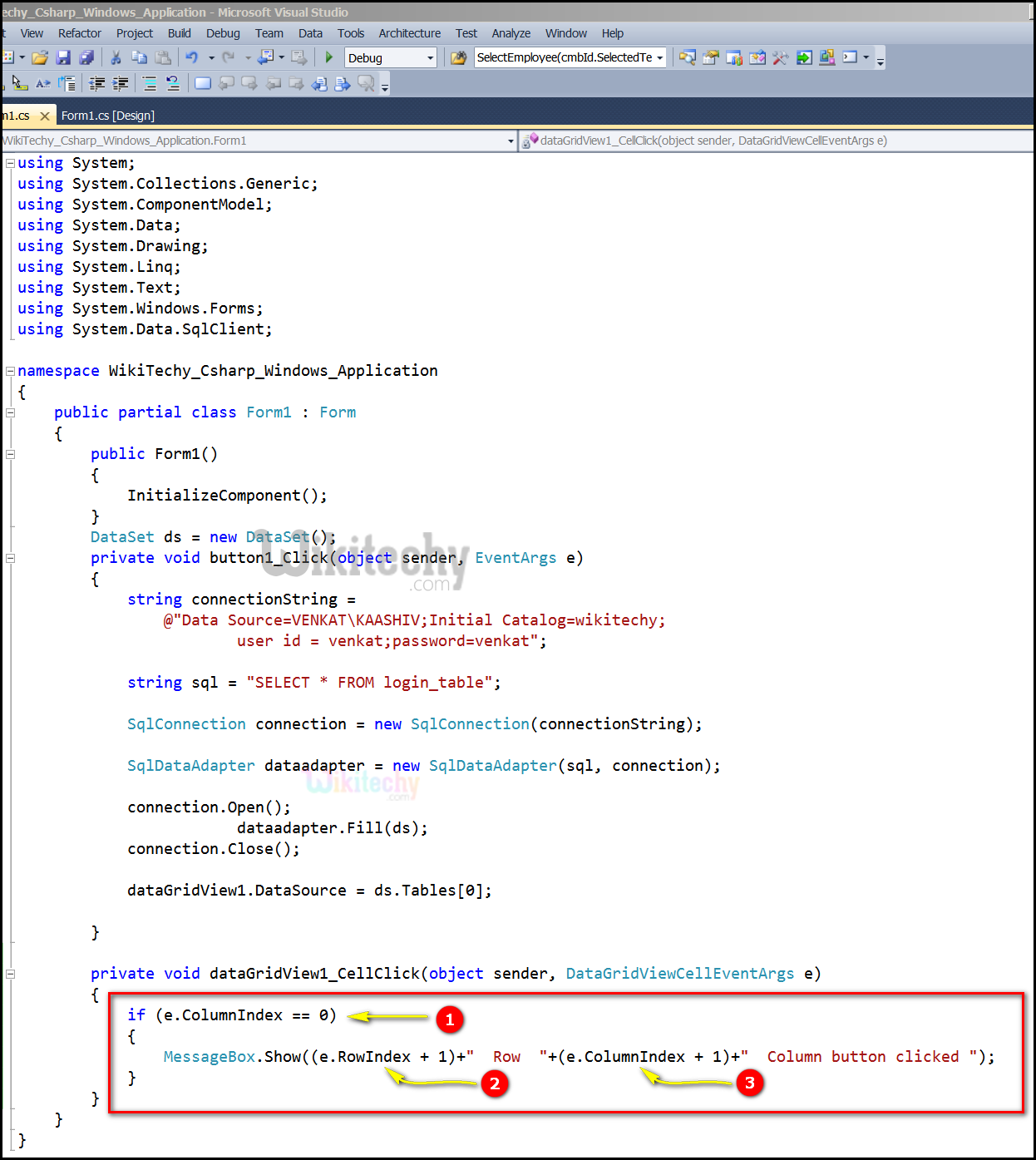
- if (e.ColumnIndex == 0) specifies to gets the column index of the current DataGridViewCell.
- MessageBox.Show((e.RowIndex + 1) + " Row " + (e.ColumnIndex + 1) + " Column button clicked "); displays a message box with the specified string "Column button clicked" which presents a message to the user.(e.RowIndex + 1) specifies to gets a value indicating the row index of the cell that event occur for DataGridView1.
- (e.ColumnIndex + 1) specifies to gets a value indicating the Column index of the cell that event occur for DataGridView1.

- In this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Button, id "1,2,3" and name "venkat, jln, arun".
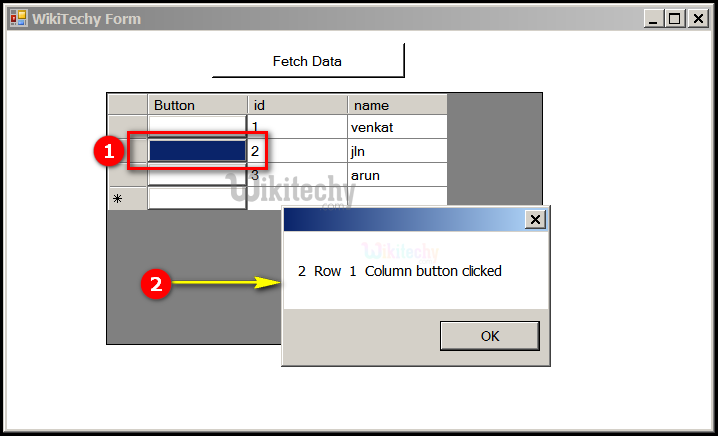
- In this output table displays the Button, id "1,2,3" and name "venkat, jln, arun" and button is clicked on "jln".
- In this output table displays the 2 Row 1 column button clicked message box window is opened.
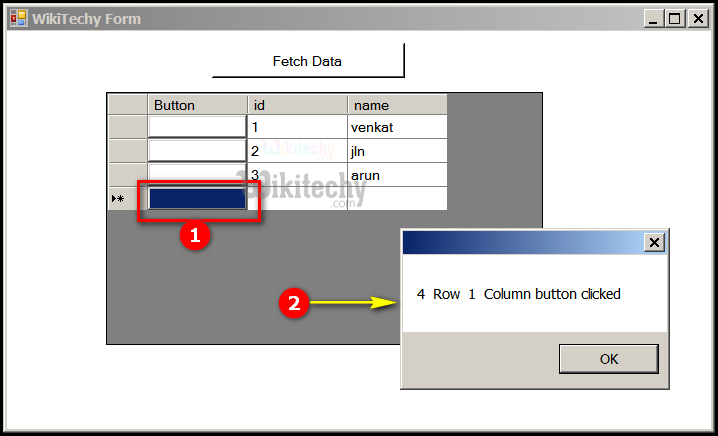
- In this output table displays the Button, id "1,2,3" and name "venkat, jln, arun" and button is clicked on empty column.
- In this output table displays the 4 Row 1 column button clicked message box window is opened.
Adding Button programmatically in Data grid view:
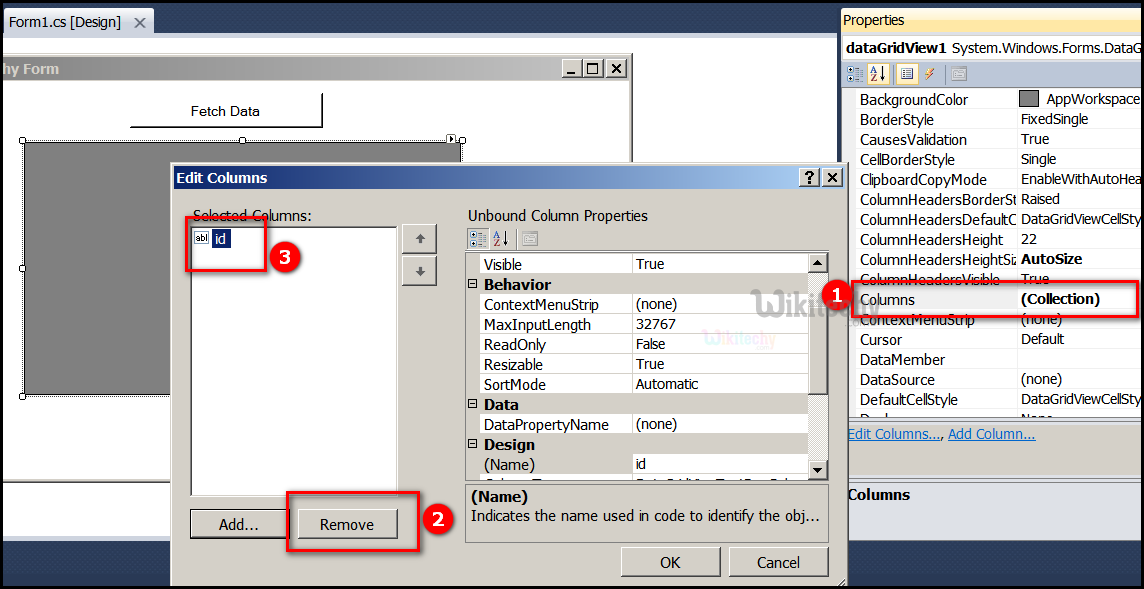
- When we click on the Collections, the String Collection Editor window will pop up where we can type strings.
- Click on "id" button.
- And then click on remove button id is removed in the Edit Columns window.
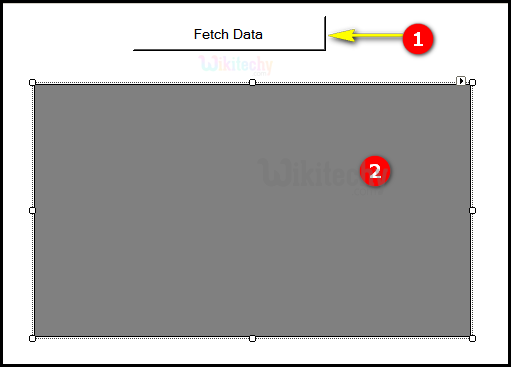
- Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the DataGridview option the form will be open.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
DataGridViewButtonColumn btn = new DataGridViewButtonColumn();
dataGridView1.Columns.Add(btn);
btn.HeaderText = "Click Data";
btn.Text = "Click Here";
btn.Name = "btn";
btn.UseColumnTextForButtonValue = true;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 2)
{
MessageBox.Show((e.RowIndex + 1)+" Row "+(e.ColumnIndex + 1)+
" Column button clicked ");
}
}
}
}
Code Explanation:
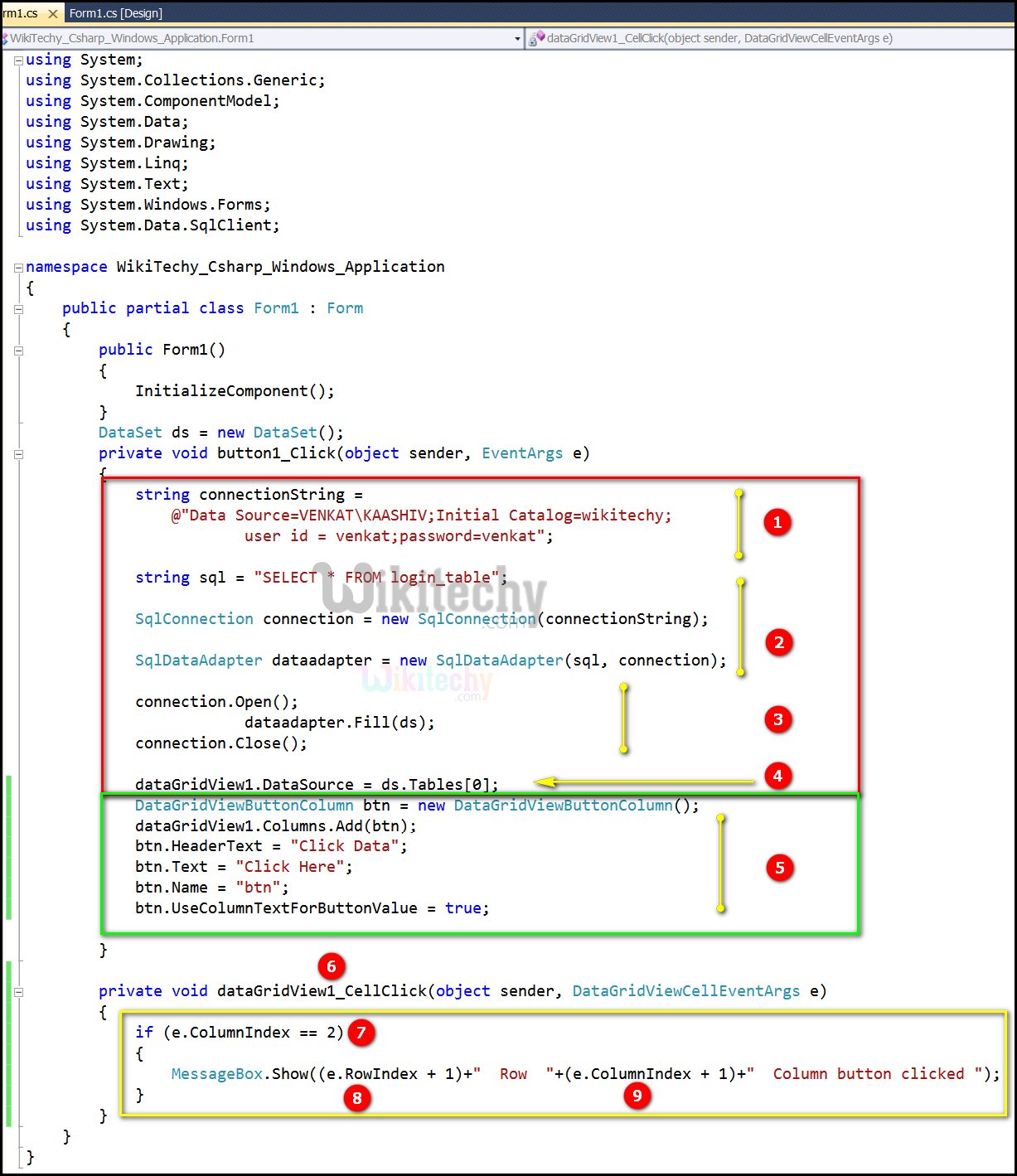
- string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;password=venkat"; connection string specifies that includes the source database name here the name is VENKAT\KAASHIV, and other parameters needed to establish the initial catalog connection is wikitechy. The default value is user id and password is venkat.
- In this example string sql = "SELECT * FROM login_table"; specifies to select the login_table in SQL server. SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource. SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection); specifies to initializes a new instance of the SqlDataAdapter class with a SelectCommand (sql) and a SqlConnection object(connection).
- connection.Open(); specifies to Open the SqlConnection.dataadapter.Fill(ds); specifies to fetches the data from User and fills in the DataSet ds.connection.Close(); it is used to close the Database Connection.
- dataGridView1.DataSource = ds.Tables[0]; is used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
- DataGridViewButtonColumn btn = new DataGridView ButtonColumn();specifies to a new instance of the DataGrid ViewButtonColumn.dataGridView1.Columns.Add(btn); specifies to add check in the column of a DataGridView , the column type contains cells of type DataGridViewCheckColumn. chk.HeaderText = "Check Data"; used to store the check data in DataTable.btn.Text = "Click Here"; specifies to text series of Unicode text " Click Here". Here chk.Name = "btn"; specifies to text series of Unicode name "btn" . btn.UseColumnTextForButtonValue = true; specified to button value in the DataGridViewButtonValue.
- private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e) click event handler for Cellclick specifies to select a row by click a cell on the datagridview.The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e).
- if (e.ColumnIndex == 2) specifies to the column index of the cell that is second formatted.
- MessageBox.Show((e.RowIndex + 1)+" Row "+(e.ColumnIndex + 1)+" Column button clicked "); displays a message box with the specified string "Column button clicked" which presents a message to the user.(e.RowIndex + 1) specifies to gets a value indicating the row index of the cell that event occur for DataGridView1.
- (e.ColumnIndex + 1) specifies to gets a value indicating the Column index of the cell that event occur for DataGridView1.
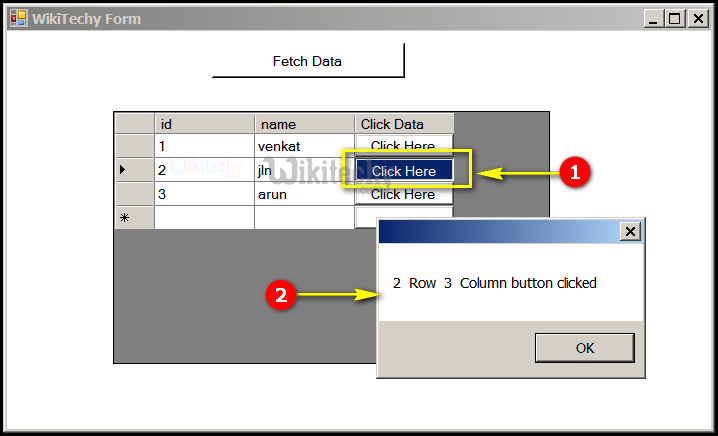
- In this output table displays the id "1,2,3" and name "venkat, jln, arun" Click Data "Click Here", and click data is clicked on "Click Here"
- In this output table displays the 2 Row 3 column button clicked message box window is opened.