C# Break - c# - c# tutorial - c# net
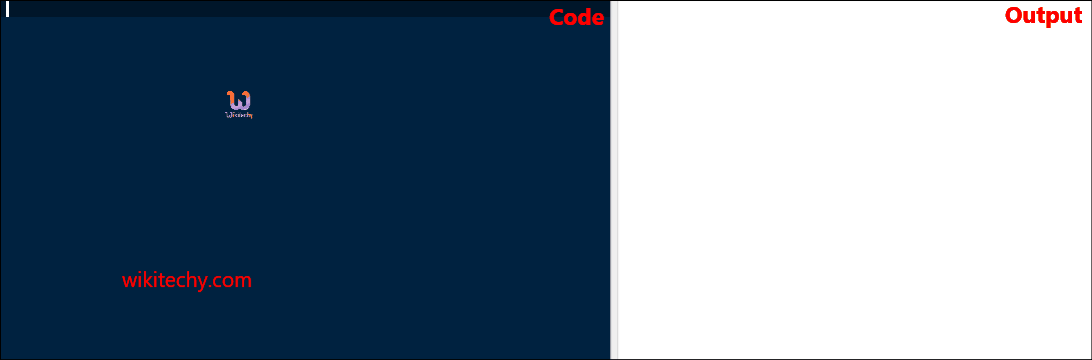
C# Break
What is break statement in C# ?
- The C# break is used to break loop or switch statement.
- It breaks the current flow of the program at the given condition.
- In case of inner loop, it breaks only inner loop.
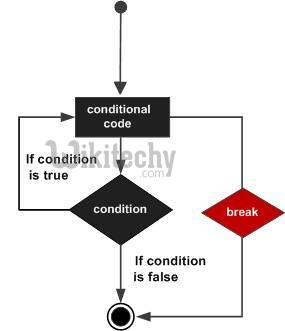
Syntax:
jump-statement;
break;
Flowchart:
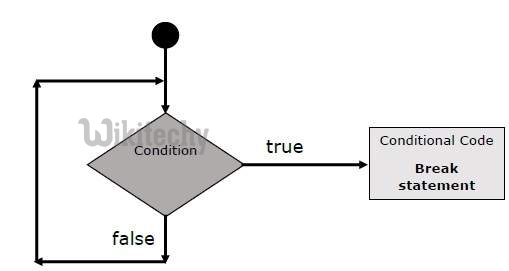
C# Break Statement Example
- Let's see a simple example of C# break statement which is used inside the loop.
using System;
public class BreakExample
{
public static void Main(string[] args)
{
for (inti = 1; i<= 10; i++)
{
if (i == 5)
{
break;
}
Console.WriteLine(i);
}
}
}
C# examples - Output :
1
2
3
4
C# Break Statement with Inner Loop
- The C# break statement breaks inner loop only if you use break statement inside the inner loop. Let's see the example code:
using System;
public class BreakExample
{
public static void Main(string[] args)
{
for(inti=1;i<=3;i++){
for(int j=1;j<=3;j++){
if(i==2&&j==2){
break;
}
Console.WriteLine(i+" "+j);
}
}
}
}
C# examples - Output :
1 1
1 2
1 3
2 1
3 1
3 2
3 3