C# if else conditional - c# - c# tutorial - c# net
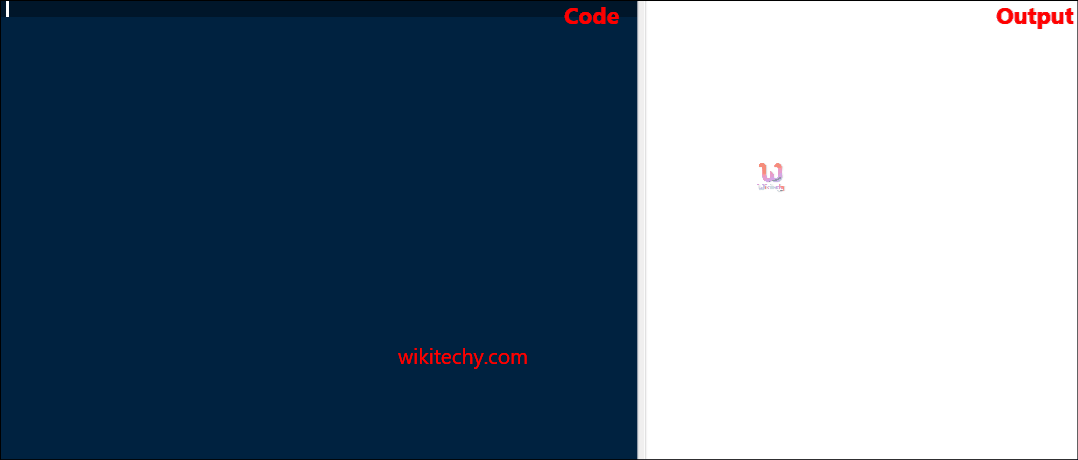
C# If Else Conditional
What is if else statement in C# ?
- In C#, an if statement can be followed by an optional else statement, which executes when the Boolean expression is false.
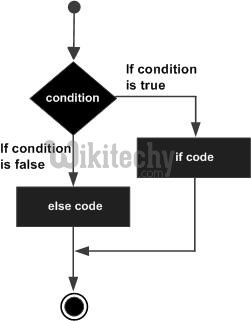
Syntax:
if (condition)
{
condition statement is true;
}
else
{
condition statement is false;
}
- If the Boolean expression evaluates to true, then the if block of code is executed, otherwise else block of code is executed.
C# Sample Code - C# Examples:
using System;
usingSystem.Collections.Generic;
usingSystem.Linq;
usingSystem.Text;
namespace wikitechy_if_else_condition
{
classProgram
{
staticvoid Main(string[] args)
{
bool condition = true;
if (condition)
{
Console.WriteLine("WikiTechy says -if else condition is true.");
}
else
{
Console.WriteLine("WikiTechy says -if else condition is false.");
}
Console.ReadLine();
}
}
}
Code Explanation:
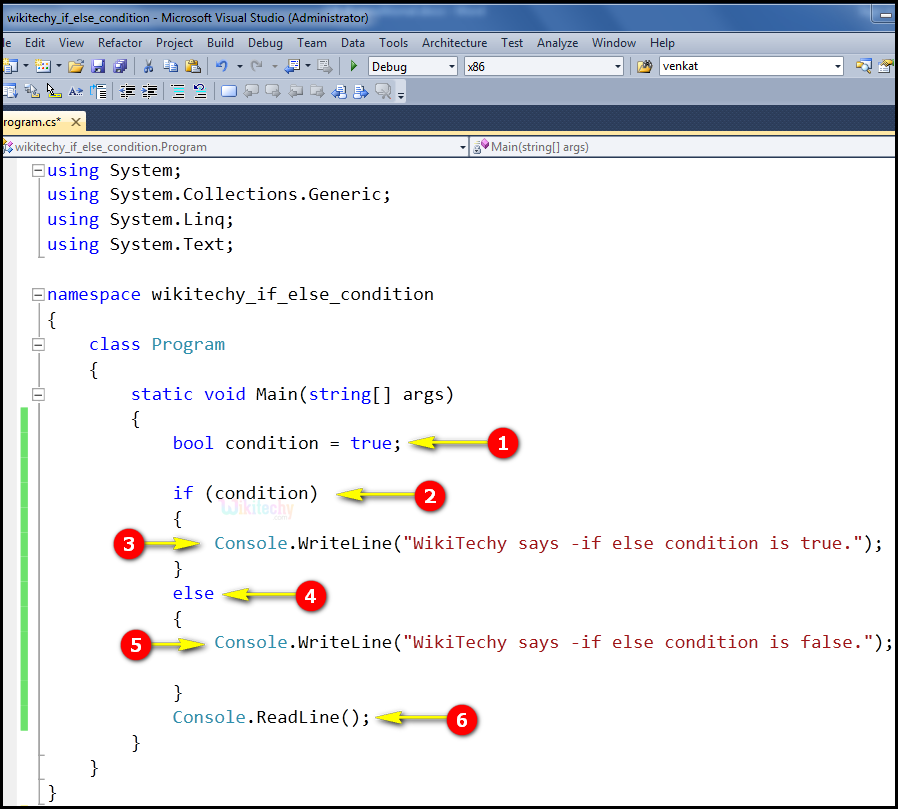
- bool condition = trueBool stores truecondition. Bool variables can be assigned values based on expressions. Many expressions evaluate to a Boolean value. Represented in one byte, the bool type represents truth.
- if (condition)specifies to If the condition is true then the control goes to the body of if block, that is the program will execute the "WikiTechy says -if else condition is true".
- In this example Console.WriteLine,the Main method specifies its behavior with the statement "WikiTechy says -if else condition is true".And WriteLine is a method of the Console class defined in the System namespace. This statement reasons the message"WikiTechy says -if else condition is true"to be displayed on the screen.
- If the condition is false then the control goes to next level, that is if we provide else block the program will execute the else statement"WikiTechy says -if else condition is false".
- In this example Console.WriteLine,the Main method specifies its behavior with the statement "WikiTechy says -if else condition is false".And WriteLine is a method of the Console class defined in the System namespace. This statement reasons the message"WikiTechy says -if else condition is false"to be displayed on the screen.
- Here Console.ReadLine(); specifies to reads input from the console. When the user presses enter, it returns a string.
Sample C# examples - Output :
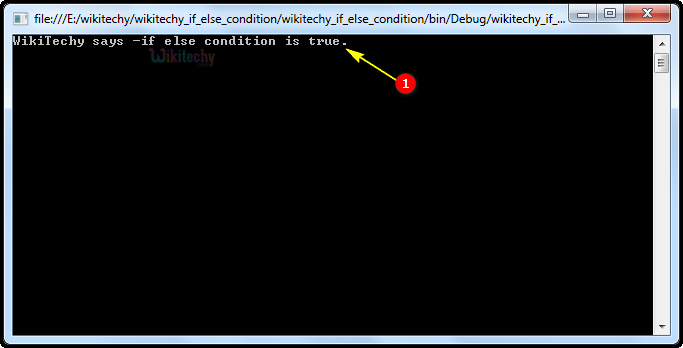
- Here in this output statement executes the if condition by printing the statement "WikiTechy says -if else condition is true".If the condition is false it goes to the else condition and prints the else statements as shown below:
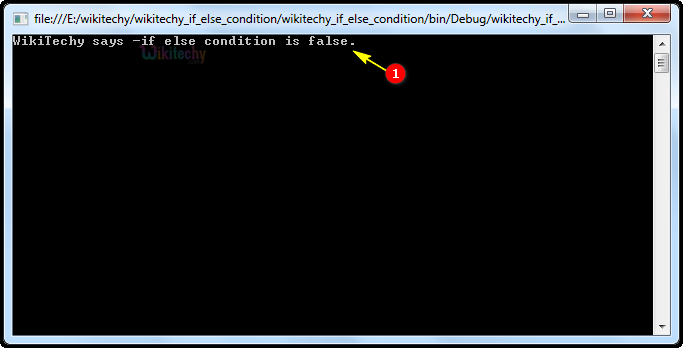
- Here the output executes else statement by printing the statement "WikiTechy says -if else condition is false".