C# Datagridview Bindingsource | C# Controls Datagridview Bind Collections - c# - c# tutorial - c# net
What is Datagridview ?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
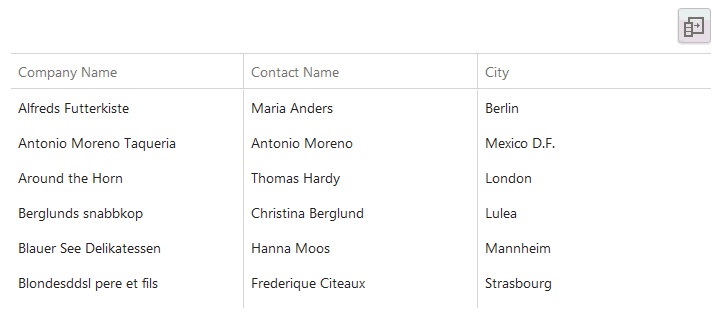
DataGridview Bind Collections
Bind Collections:
- In C#, the DataGridView control supports the standard Windows Forms data binding model, so it will bind to a variety of data sources.
- In most conditions, we will bind to a BindingSource component which will manage the details of interacting with the data source.
- The BindingSource component can represent any Windows Forms data source and gives to us great flexibility when choosing or modifying the location of our data.
Syntax Example
List<student> items = new List<student>();
- Is called as generics, the variable is nothing but the class itself.
- Here in the below table is representing to Windows Forms:
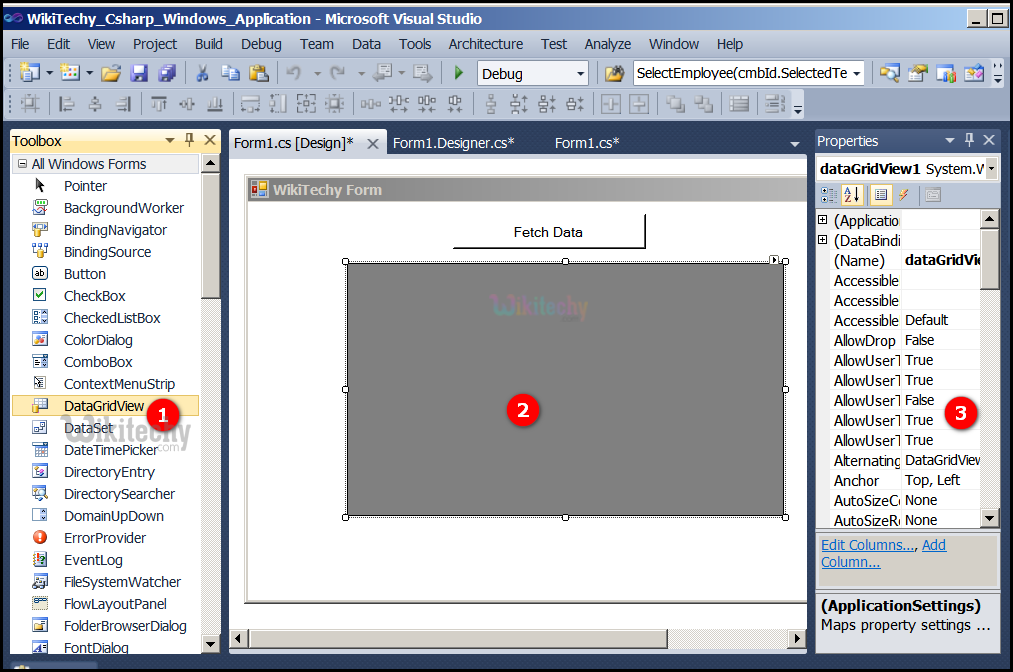
- The DataGridview specifies to displays data from SQL databases.
- Go to tool box and click on the DataGridview option the form will be open.
- Here the Properties window, specifies to properties for the form. Forms have various properties. For example, we can set the foreground and background color, title text that appears at the top of the form, size of the form, and other properties.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
class student
{
public string Name { get; set; }
public int id { get; set; }
// WikiTechy C# code
public student(string name, int id)
{
this.Name = name;
this.id = id;
}
}
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
List<student> items = new List<student>();
items.Add(new student("venkat", 1));
items.Add(new student("jln", 2));
items.Add(new student("arun", 3));
items.Add(new student("arun", 3));
// WikiTechy C# code
// string array to data grid view
dataGridView1.DataSource = items;
}
}
}
Code Explanation:
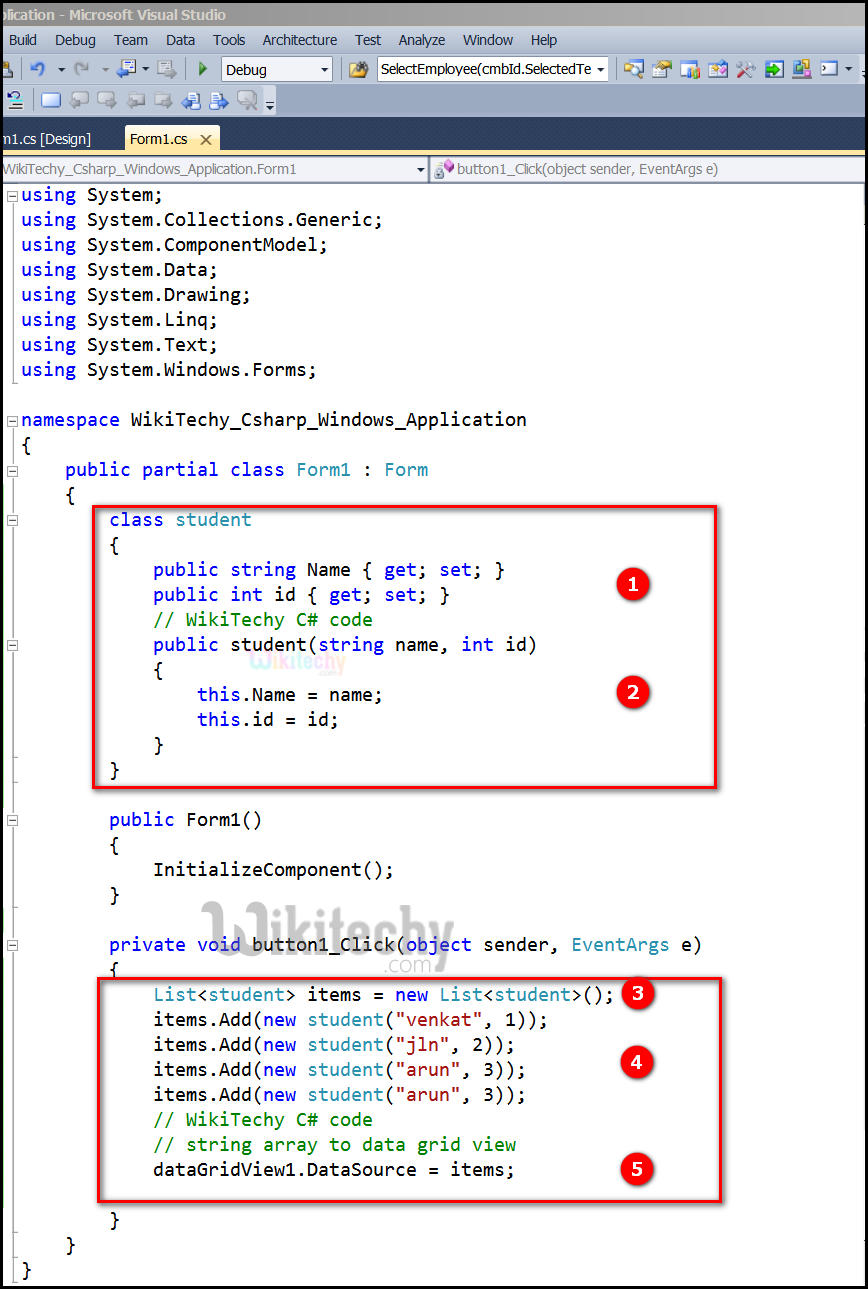
- Here public string Name { get; set; } specifies to the accessor declare a Name property of type string can contain a get accessor, a set accessor, or both. Here in this example public int id { get; set; } specifies to the accessor declare a id property of type string can contain a get accessor, a set accessor, or both.
- Here this.Name = name; and this.id = id; specifies to qualify of the fields, such as name and id.
- List<student> items = new List<student>(); specifies to list of Student objects.
- Here in this example items.Add(new student("venkat", 1)); items.Add(new student("jln", 2)); items.Add(new student("arun", 3)); items.Add(new student("arun", 3)); specifies to add the student name and id.
- Here dataGridView1.DataSource = items; used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.