C# Program Structure - c# - c# tutorial - c# net
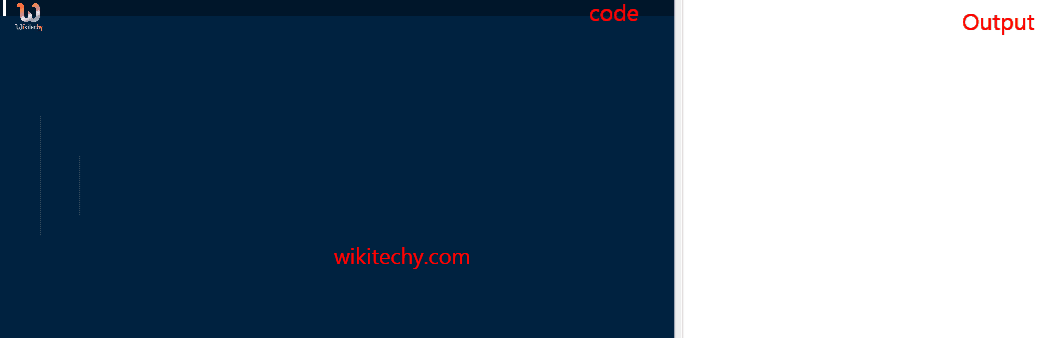
C# Program Structure
How to Create a programming structure in C#?
- It is very simple to use structure in C#.
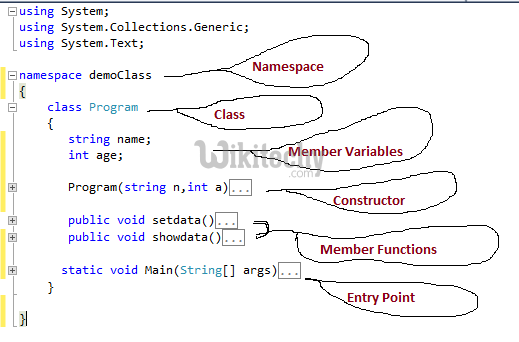
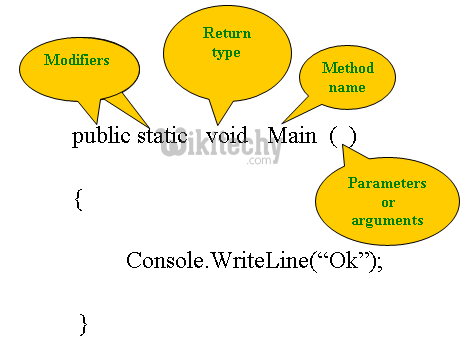
c# windows applications - program structure :
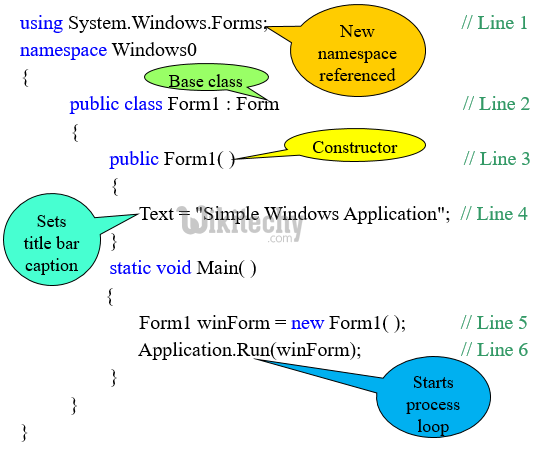
c# program hierarchy :
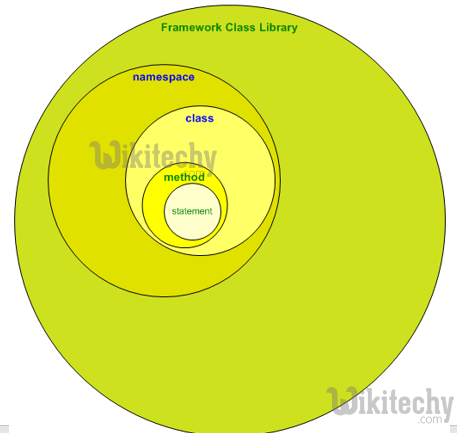
Comments in c# :
- Make the code more readable
- Three types of commenting syntax
- 1. Inline comments
- 2. Multiline comments
- 3. XML documentation comments
Inline Comments :
- Indicated by two forward slashes (//)
- Considered a one-line comment
- Everything to the right of the slashes ignored by the compiler
- Carriage return (Enter) ends the comment
- Example - > // This is wikitechy first program written by venkat.
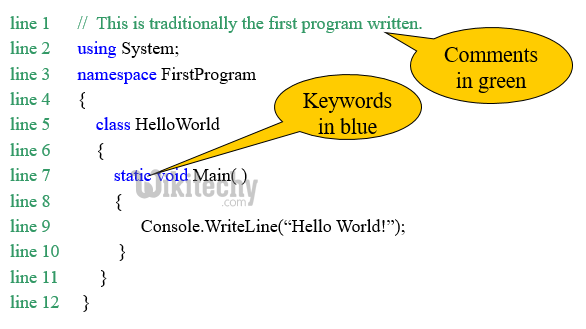
Multiline Comment :
- Forward slash followed by an asterisk (/*) marks the beginning
- Opposite pattern (*/) marks the end
- Also called block comments
- Example - > /* This is the beginning of a block multiline comment. It can go on for several lines or just be on a single line. No additional symbols are needed after the beginning two characters. Notice there is no space placed between the two characters. To end the comment, use the following symbols. */
XML Documentation Comments :
- Extensible Markup Language (XML)
- Markup language that provides a format for describing data using tags Similar to HTML tags
- Three forward slashes (///) mark beginning of comment
- Advanced documentation technique used for XML-style comments
- Compiler generates XML documentation from them
c# namespace :
- Namespaces provide scope for the names defined within the group
- Groups semantically related types under a single umbrella
- System:System: most important and frequently used namespace
- most important and frequently used namespace
- Can define your own namespace
- Each namespace enclosed in curly braces: { }
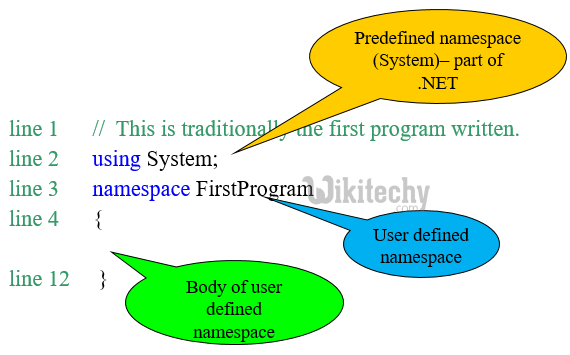
c# Main( ) function :
- "Entry point" for all applications
- Where the program begins execution
- Execution ends after last statement in Main( )
- Can be placed anywhere inside the class definition
- Applications must have one Main( ) method
- Begins with uppercase character
- static void Main( ) Begins with the keyword static – a static member is a member of a class that isn't associated with an instance of a class. Instead, the member belongs to the class itself.
- Second keyword → return type
- void signifies no value returned
- Name of the method
- Main is the name of Main( ) method
- Parentheses "( )" used for arguments
- No arguments for Main( ) – empty parentheses
c# Body of a Method :
- Enclosed in curly braces
- Example Main( ) method body
- line 7 static void Main( )
- line 8 {
- line 9 Console.WriteLine("Hello World!");
- line 10 }
- Includes program statements
- Calls to other method
- Here Main( ) calling WriteLine( ) method
c# Escape Sequence :
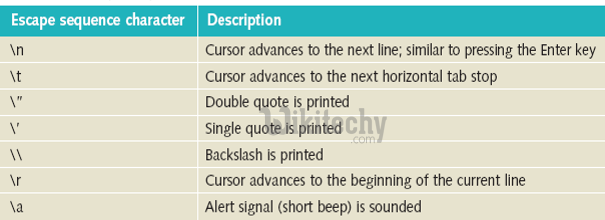
c# - Types of errors :
- Syntax errors
- Typing error
- Misspelled name
- Forget to end a statement with a semicolon
- Run-time errors
- Failing to fully understand the problem
- More difficult to detect
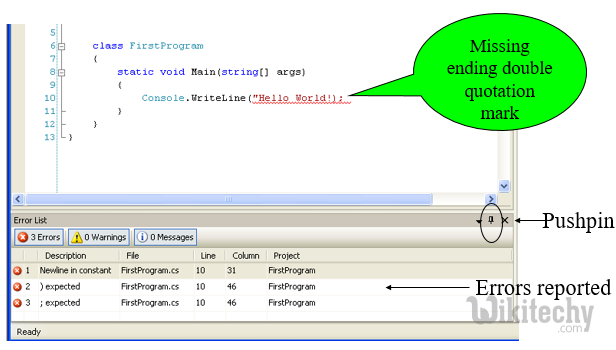
c# naming convention :
-
Pascal case
- First letter of each word capitalized
- Class, metahod, namespace, and properties identifiers
- Camel case
- Hungarian notation
- First letter of identifier lowercase; first letter of subsequent concatenated words capitalized
- Variables and objects
- Every character is uppercase
- Constant literals and for identifiers that consist of two or fewer letters
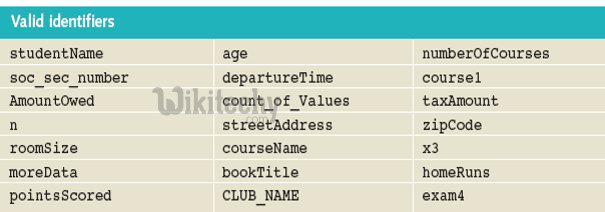
c# invalid naming convention - c# invalid identifiers :
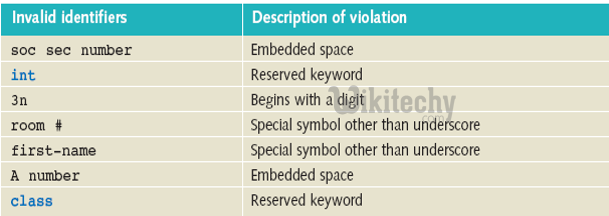
c# Assemblies :
Code contained in files called "assemblies"
- code and metadata
- It can be .exe or .dll
- Executable needs a class with a "Main" method: public static void Main(string[] args)
-
Various types of assemblies
- local : local assembly, not accessible by others
- shared : well-known location, can be GAC - Gobal Assembly Cache
- strong names : use crypto for signatures then can add some versioning and trust
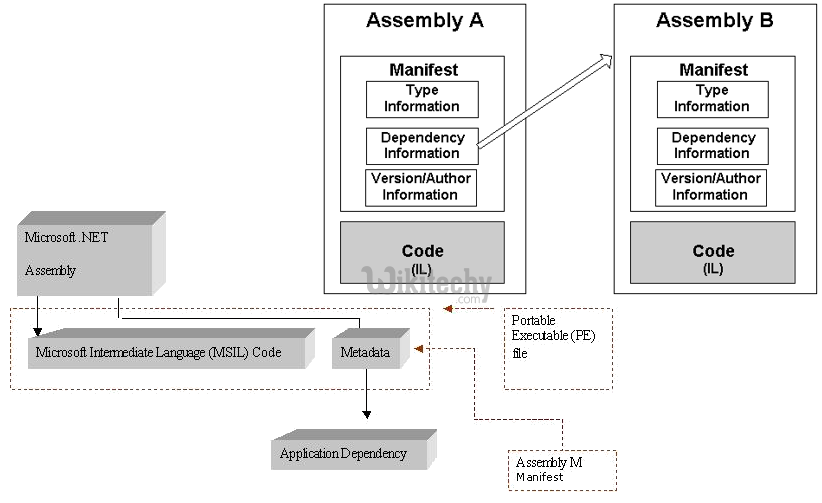
The following programming example we will show how to create and use structure in C# programming.
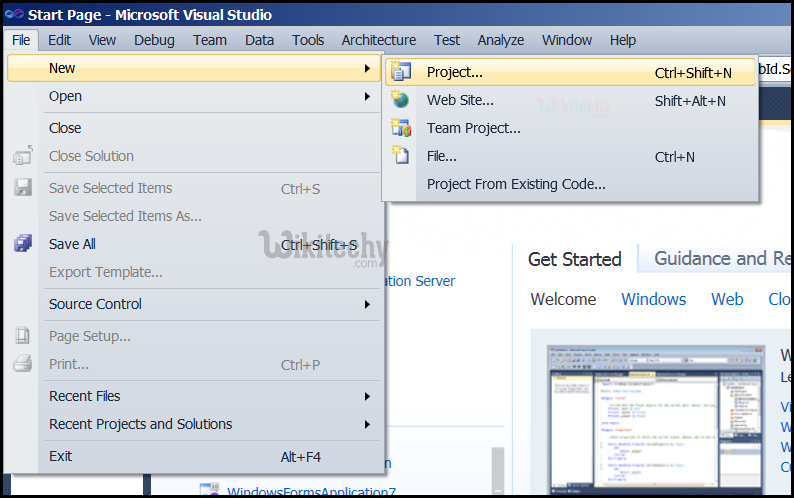
- Go to Start and type Visual Studio.
- On the menu bar and choose File, New, click on Project.
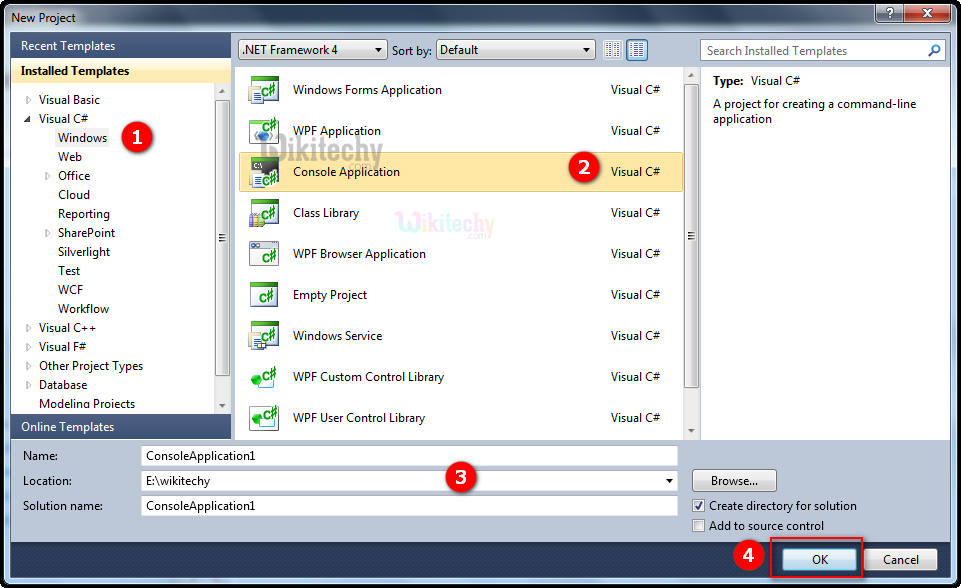
- And then select Windows Forms Application from the center pane.
- Expand Installed, expand Templates, expand Visual C#, and then choose Console Application.
- In the Name box, specify a name for our project, and then choose
- And Click on OK button. The new project appears in Solution Explorer.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to wikitechy world - C#..!");
Console.WriteLine("Press any key to exit.");
Console.ReadKey();
}
}
}
Code Explanation:
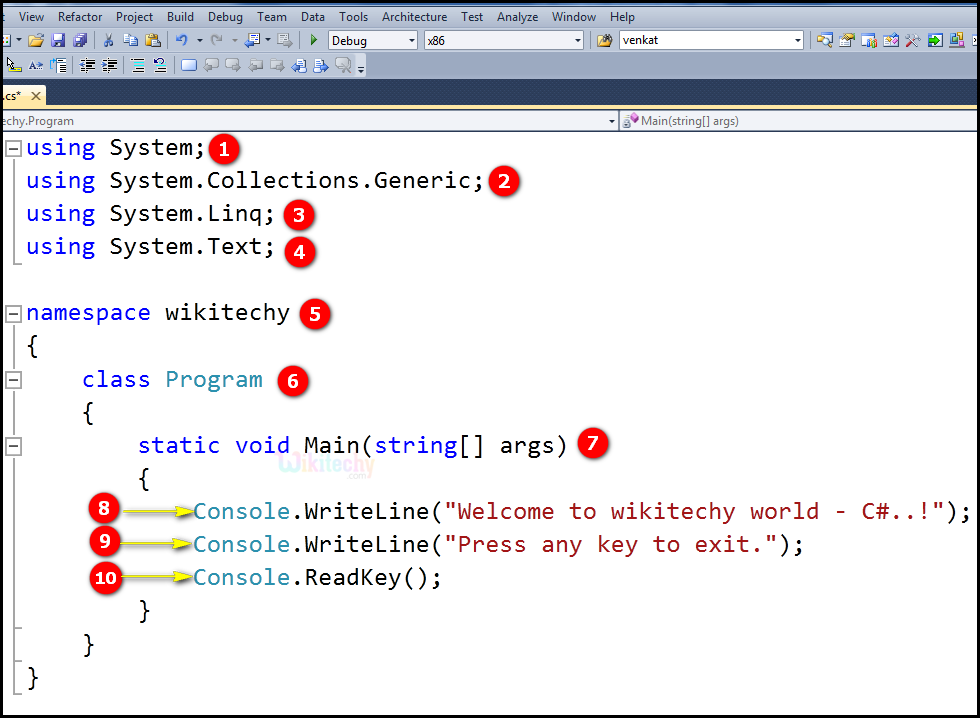
- using System; it is a keyword, these key word is used to include the System namespace in the program.
- using System.Collections.Generic namespace contains interfaces and classes that define generic collections, which allow users to create strongly typed collections that provide better type safety and performance than non-generic strongly typed collections.
- using System.Linq the System.Linq namespace provides classes and interfaces that support queries that use Language-Integrated Query (LINQ).
- using System.Text the namespace contains classes that represent ASCII and Unicode character encodings.
- A namespace is a collection of classes. The wikitechy Application namespace contains the class wikitechy.
- class Program is specifying to class wikitechy contains the data and method definitions that our program uses. Classes generally contain multiple methods. Methods define the behavior of the class. However, the wikitechy class has only one method Main.
- Here static void Main(string[] args) main method, which is the entry point for all C# programs. The Main method states what the class does when executed.
- In Console.WriteLine, the Main method specifies its behavior with the statement "Welcome to wikitechy world - C#..!". WriteLine is a method of the Console class defined in the System namespace. This statement reasons the message " Welcome to wikitechy world - C#..!" to be displayed on the screen.
- In this example Console.WriteLine, the Main method specifies its behavior with the statement "Press any key to exit.". WriteLine is a method of the Console class defined in the System namespace. This statement reasons the message "Press any key to exit." to be displayed on the screen.
- Here Console.ReadKey(); is used for the VS.NET Users. This makes the program wait for a key press and it prevents the screen from running and closing quickly when the program is launched from Visual Studio .NET.
Sample C# examples - Output :
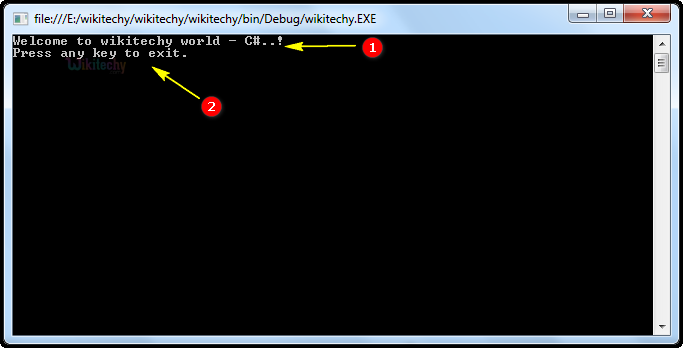
- Here in this output we display the "Welcome to wikitechy world - C#..!" which specifies to console WriteLine statement.
- Here in this output we display the "Press any key to exit." which specifies to console WriteLine statement.