C# FileStream - c# - c# tutorial - c# net
What is C# FileStream ?
- C# FileStream class provides a stream for file operation.
- It can be used to perform synchronous and asynchronous read and write operations.
- By the help of FileStream class, we can easily read and write data into file.
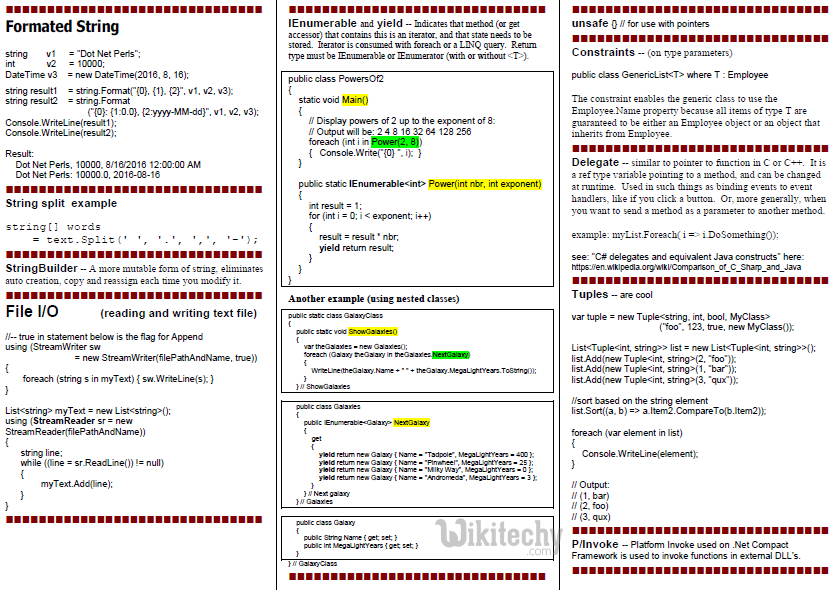
learn csharp tutorial - c# file io - c# example programs
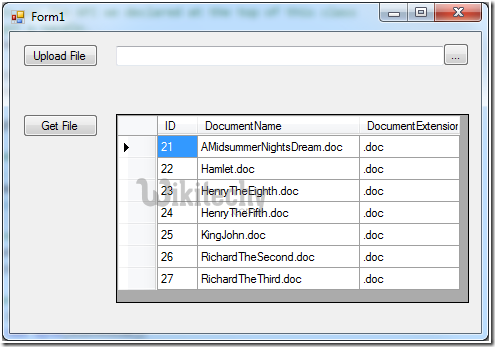
C# FileStream
C# FileStream example: writing single byte into file
- Let's see the simple example of FileStream class to write single byte of data into file. Here, we are using OpenOrCreate file mode which can be used for read and write operations.
using System;
using System.IO;
public class FileStreamExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\b.txt", FileMode.Ope
nOrCreate);//creating file stream
f.WriteByte(65);//writing byte into stream
f.Close();//closing stream
}
}
C# examples - Output :
A
C# FileStream example: writing multiple bytes into file
- Let's see another example to write multiple bytes of data into file using loop.
using System;
using System.IO;
public class FileStreamExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\b.txt", FileMode.OpenOrCreate);
for (int i = 65; i <= 90; i++)
{
f.WriteByte((byte)i);
}
f.Close();
}
}
C# examples - Output :
ABCDEFGHIJKLMNOPQRSTUVWXYZ
C# FileStream example: reading all bytes from file
- Let's see the example of FileStream class to read data from the file. Here, ReadByte() method of FileStream class returns single byte. To all read all the bytes, you need to use loop.
using System;
using System.IO;
public class FileStreamExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\b.txt", FileMode.OpenOrCreate);
int i = 0;
while ((i = f.ReadByte()) != -1)
{
Console.Write((char)i);
}
f.Close();
}
}
C# examples - Output :
ABCDEFGHIJKLMNOPQRSTUVWXYZ