C# StreamReader - c# - c# tutorial - c# net
What is StreamReader Class in C# ?
- The StreamReader class also inherits from the abstract base class TextReader that represents a reader for reading series of characters.
- C# StreamReader class is used to read string from the stream.
- It inherits TextReader class. It provides Read() and ReadLine() methods to read data from the stream.
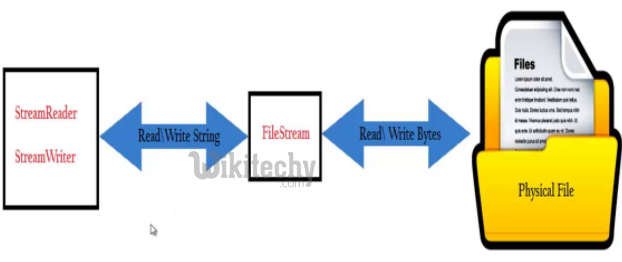
Stream writer and stream reader
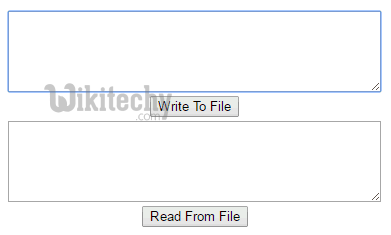
C# StreamReader
Important Points about StreamReader Class
- Implements a TextReader that reads characters from a byte stream in a particular encoding.
- StreamReader class uses UTF-8 Encoding by defaults.
- StreamReader class is designed for character input in a particular encoding.
- Use this class for reading standard text file.
- By default, it is not thread safe.
- The following table shows some members of the StreamReader class.
Member | Description |
---|---|
Close() | Closes the current StreamReader object and the underlying stream. This method is equivalent to Dispose(), that is used to release resources. |
Read() | Reads the next character from the input stream. |
ReadLine() | Reads a line of characters from the current stream and returns the data as a string. |
ReadToEnd() | Reads the stream from the current position to the end of the stream. |
C# StreamReader example to read one line:
- Let's see the simple example of StreamReader class that reads a single line of data from the file.
using System;
using System.IO;
public class StreamReaderExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\output.txt", FileMode.OpenOrCreate);
StreamReader s = new StreamReader(f);
string line=s.ReadLine();
Console.WriteLine(line);
s.Close();
f.Close();
}
}
C# examples - Output :
Hello C#
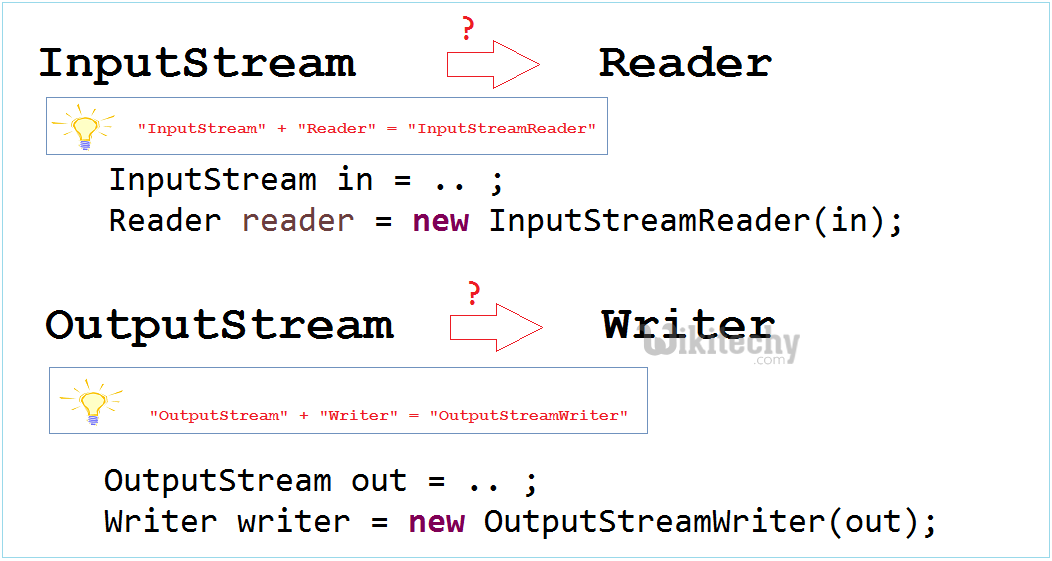
stream writer and stream reader
C# StreamReader example to read all lines:
using System;
using System.IO;
public class StreamReaderExample
{
public static void Main(string[] args)
{
FileStream f = new FileStream("e:\\a.txt", FileMode.OpenOrCreate);
StreamReader s = new StreamReader(f);
string line = "";
while ((line = s.ReadLine()) != null)
{
Console.WriteLine(line);
}
s.Close();
f.Close();
}
C# examples - Output :
Hello C#
this is file handling