C# Arithmetic Operators | arithmetic operators in c# with example - c# - c# tutorial - c# net
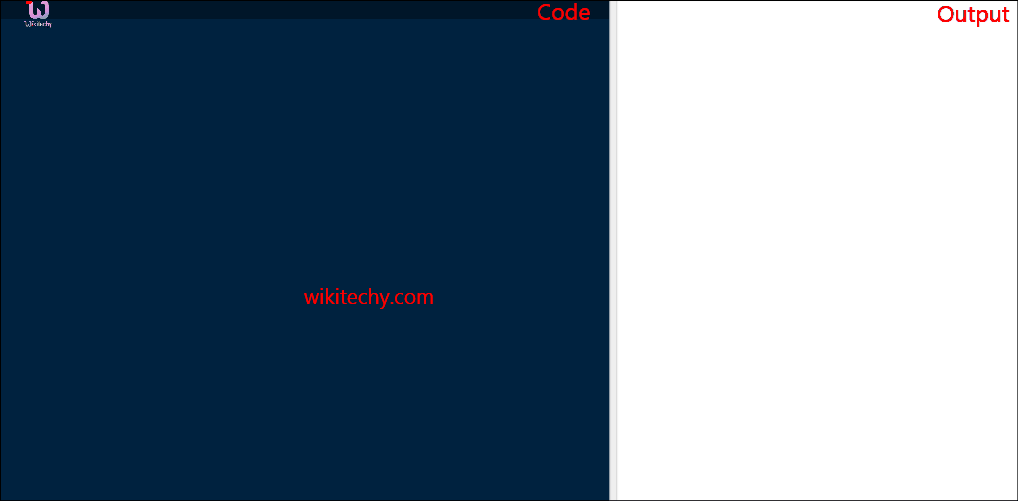
C# Arithemetic Operators
What is Arithmetic Operator in C# ?
- Arithmetic operators take numerical values (either literals or variables) as their operands and return a single numerical value.
- In C#-Programming the Arithmetic operators are used to perform mathematical calculations such as,
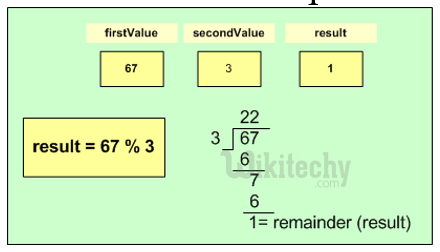
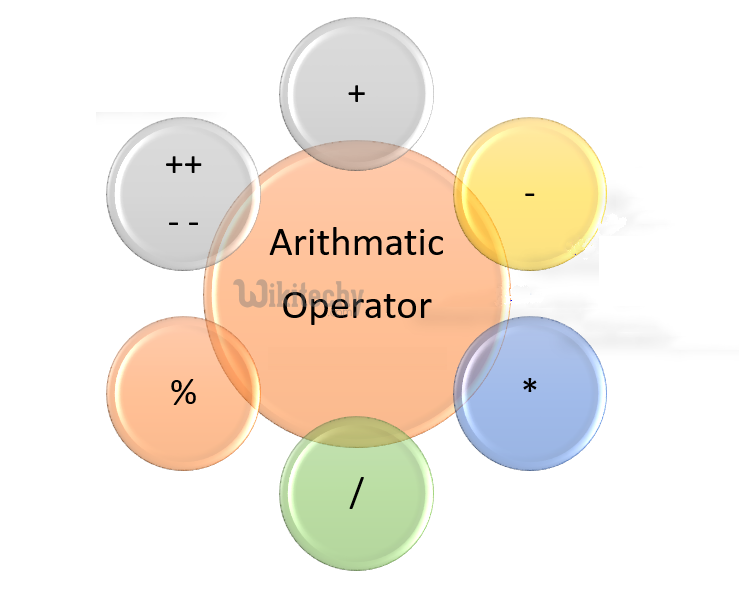
Operator | Description | Example |
---|---|---|
+ | Adds two operands | A + B = 30 |
- | Subtracts second operand from the first | A - B = -10 |
* | Multiplies both operands | A * B = 200 |
/ | Divides numerator by de-numerator | B / A = 2 |
% | Modulus Operator and remainder of after an integer division | B % A = 0 |
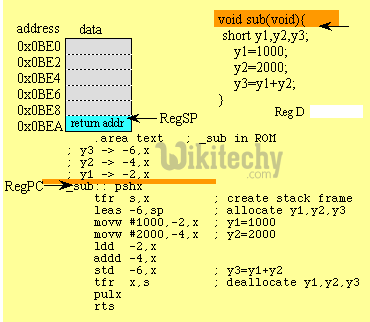
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_arithmetic_operators
{
class Program
{
static void Main(string[] args)
{
int n = 10;
int m = 5;
int x;
x = n + m;
Console.WriteLine("Addition Value of x is : {0}", x);
x = n - m;
Console.WriteLine("Subtraction Value of x is : {0}", x);
x = n * m;
Console.WriteLine("Multiplication Value of x is : {0}", x);
x = n / m;
Console.WriteLine("Division Value of x is : {0}", x);
x = n % m;
Console.WriteLine("Modulus Value of x is : {0}", x);
Console.ReadLine();
}
}
}
Code Explanation:
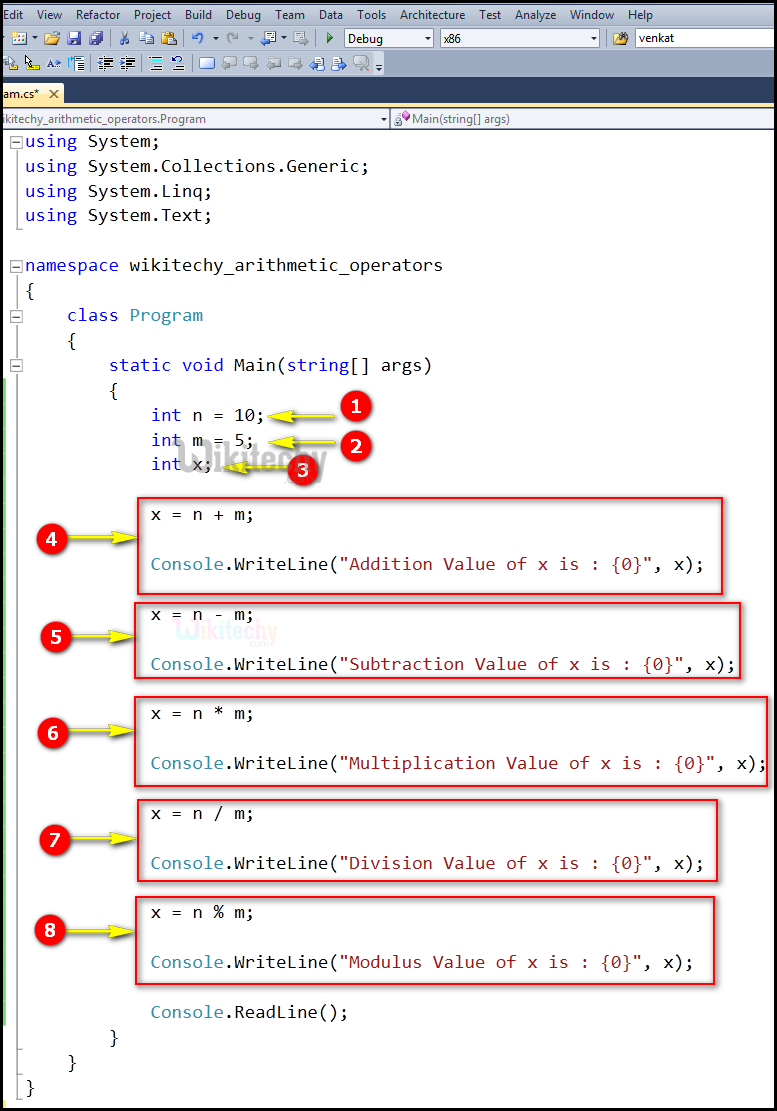
- Here we declare the integer variables n and assigning the value of the variable n=10. and m=5.
- Here we declare another integer variables m and assigning the value of the variable m=5.
- Here int x; is an integer variable.
- In this statement, we perform an arithmetic operation for the variable "n" and "m" and the output value will be stored in the variable "x". And Console.WriteLine, the Main method specifies its behavior with the statement "Addition Value of x is:" to be displayed on the screen.
- In this statement, we perform a subtraction operation for the variable "n" and "m" and the output value will be stored in the variable "x". And Console.WriteLine, the Main method specifies its behavior with the statement "Subtraction Value of x is:" to be displayed on the screen.
- In this statement, we perform a multiplication operation for the variable "n" and "m" and the output value will be stored in the variable "x". And Console.WriteLine, the Main method specifies its behavior with the statement "Multiplication Value of x is:" to be displayed on the screen.
- In this statement, we perform a division operation for the variable "n" and "m" and the output value will be stored in the variable "x". And Console.WriteLine, the Main method specifies its behavior with the statement "Division Value of x is:" to be displayed on the screen.
- In this statement, we perform a mod operation for the variable "n" and "m" and the output value will be stored in the variable "x". Console.WriteLine, the Main method specifies its behavior with the statement " Modulus Value of x is:" to be displayed on the screen
Sample C# examples - Output :
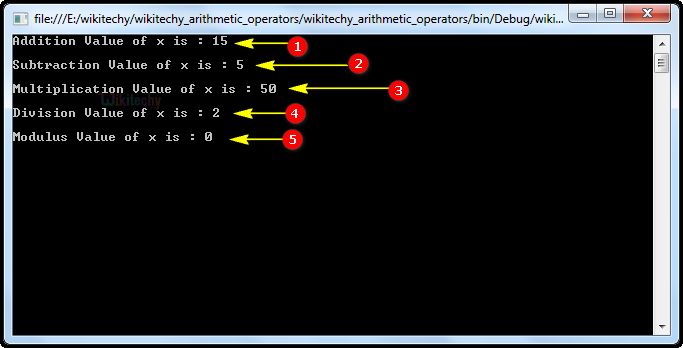
- Here the arithmetic operation for the variable "n" (value=10) and "m" (value=5) has been shown with its output result as "Addition Value of x is: 15".
- Here the subtraction operation for the variable "n" (value=10) and "m" (value=5) has been shown with its output result as "Subtraction Value of x is: 5".
- multiplication operation for the variable "n" (value=10) and "m" (value=5) has been shown with its output result as "Subtraction Value of x is: 50".
- division operation for the variable "an" (value=10) and "m" (value=5) has been shown with its output result as "Division Value of x is: 2".
- mod operation for the variable "n" (value=10) and "m" (value=5) has been shown with its output result as "Division Value of x is: 0".
Basic Arithmetic Operations with strings :
string result;
string fullName;
string firstName = "Rochelle";
string lastName = "Howard";
fullName = firstName + " " + lastName;
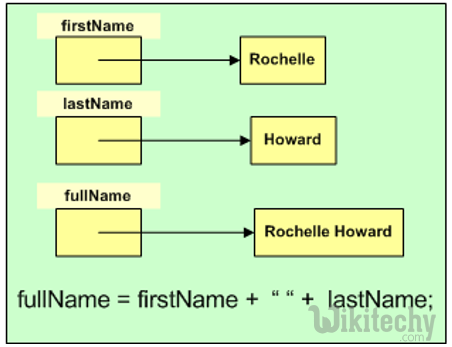
Read Also
dotnet institute in chennai , dot net developer internship , dot net training and placement institutesc# compound arithmetic operator :
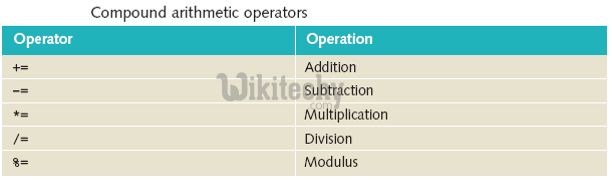
c# operator precedence :
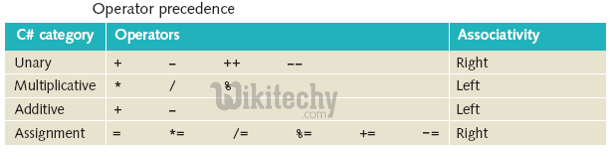
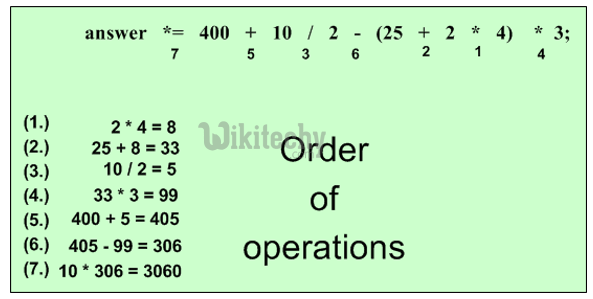
Operators in C# :
- Unary – take one operand
- Binary – take two operands
- Ternary (?:) – takes three operands