C# Polymorphism - c# - c# tutorial - c# net
What is polymorphism in C# ?
- The term "Polymorphism" is the combination of "poly" + "morphs" which means many forms.
- It is a greek word. In object-oriented programming, we use 3 main concepts:
- inheritance,
- encapsulation and
- polymorphism.
- There are two types of polymorphism in C#:
- compile time polymorphism and
- runtime polymorphism.
- Compile time polymorphism is achieved by method overloading and operator overloading in C#.
- It is also known as static binding or early binding. Runtime polymorphism in achieved by method overriding which is also known as dynamic binding or late binding.
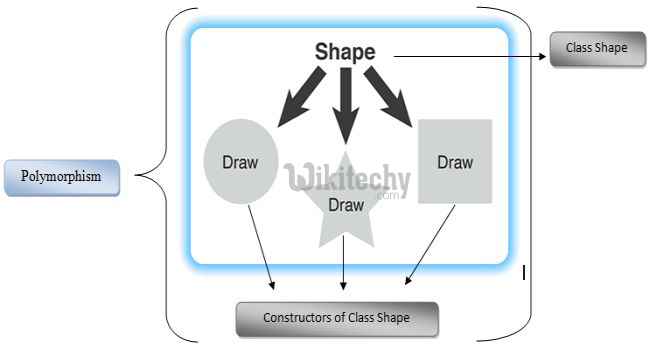
polymorphism in c# Example
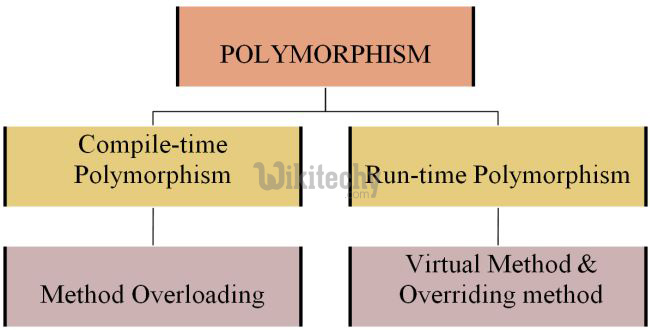
Polymorphism
C# Runtime Polymorphism Example
- Let's see a simple example of runtime polymorphism in C#.
using System;
public class Animal{
public virtual void eat(){
Console.WriteLine("eating...");
}
}
public class Dog: Animal
{
public override void eat()
{
Console.WriteLine("eating bread...");
}
}
public class TestPolymorphism
{
public static void Main()
{
Animal a= new Dog();
a.eat();
}
}
C# examples - Output :
eating bread...
Read Also
dot net developer training , dot net training institute in chennai , net course duration and feesC# Runtime Polymorphism Example 2
- Let's see a another example of runtime polymorphism in C# where we are having two derived classes.
using System;
public class Shape{
public virtual void draw(){
Console.WriteLine("drawing...");
}
}
public class Rectangle: Shape
{
public override void draw()
{
Console.WriteLine("drawing rectangle...");
}
}
public class Circle : Shape
{
public override void draw()
{
Console.WriteLine("drawing circle...");
}
}
public class TestPolymorphism
{
public static void Main()
{
Shape s;
s = new Shape();
s.draw();
s = new Rectangle();
s.draw();
s = new Circle();
s.draw();
}
}
C# examples - Output :
drawing...
drawing rectangle...
drawing circle...
Runtime Polymorphism with Data Members
- Runtime Polymorphism can't be achieved by data members in C#. Let's see an example where we are accessing the field by reference variable which refers to the instance of derived class.
Read Also
summer internship with stipend , dot net course fees in chennai , dot net developer internshipusing System;
public class Animal{
public string color = "white";
}
public class Dog: Animal
{
public string color = "black";
}
public class TestSealed
{
public static void Main()
{
Animal d = new Dog();
Console.WriteLine(d.color);
}
}
C# examples - Output :
white