C# Gridview Hide Column | C# Controls Datagridview Hide Columns Rows - c# - c# tutorial - c# net
What is Datagridview?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
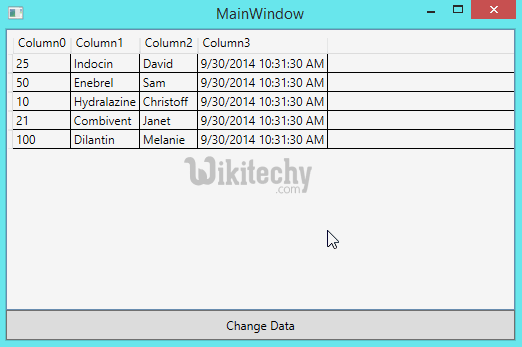
DataGridview Hide Show Columns
Hide columns Rows:
- In C#, displaying data in a tabular format is a task we are likely to perform frequently.
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- In C#, the source code manually creates a DataGridView columns and rows and hide the second column and second row.
Syntax Example
dataGridView1.Rows[Index].Visible = false;
dataGridView1.Columns[Index].Visible = false;
- Here in the below table is representing to Windows Forms:
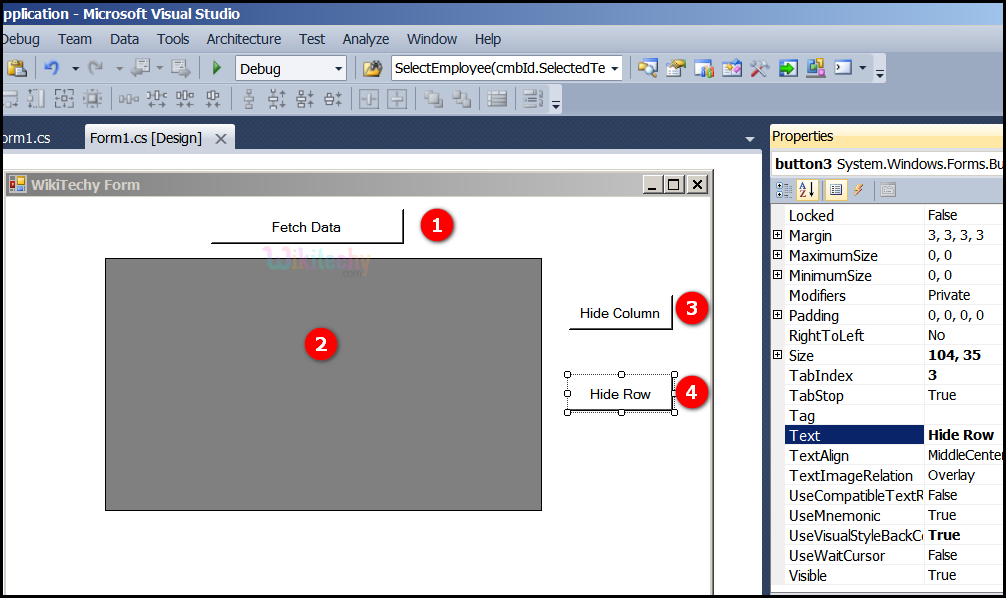
- Here Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the DataGridview option the form will be open.
- Go to tool box and click on the button option and put the name as Hide Column in the button.
- Go to tool box and click on the button option and put the name as Hide Row in the button.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString =@"Data Source=VENKAT\KAASHIV;
Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
dataGridView1.Columns[1].Visible = false;
}
private void button3_Click(object sender, EventArgs e)
{
dataGridView1.Rows[1].Visible = false;
}
}
}
Read Also
dotnet questions for interview , how to learn dotnet for beginners , how to learn dotnet for beginnersCode Explanation:
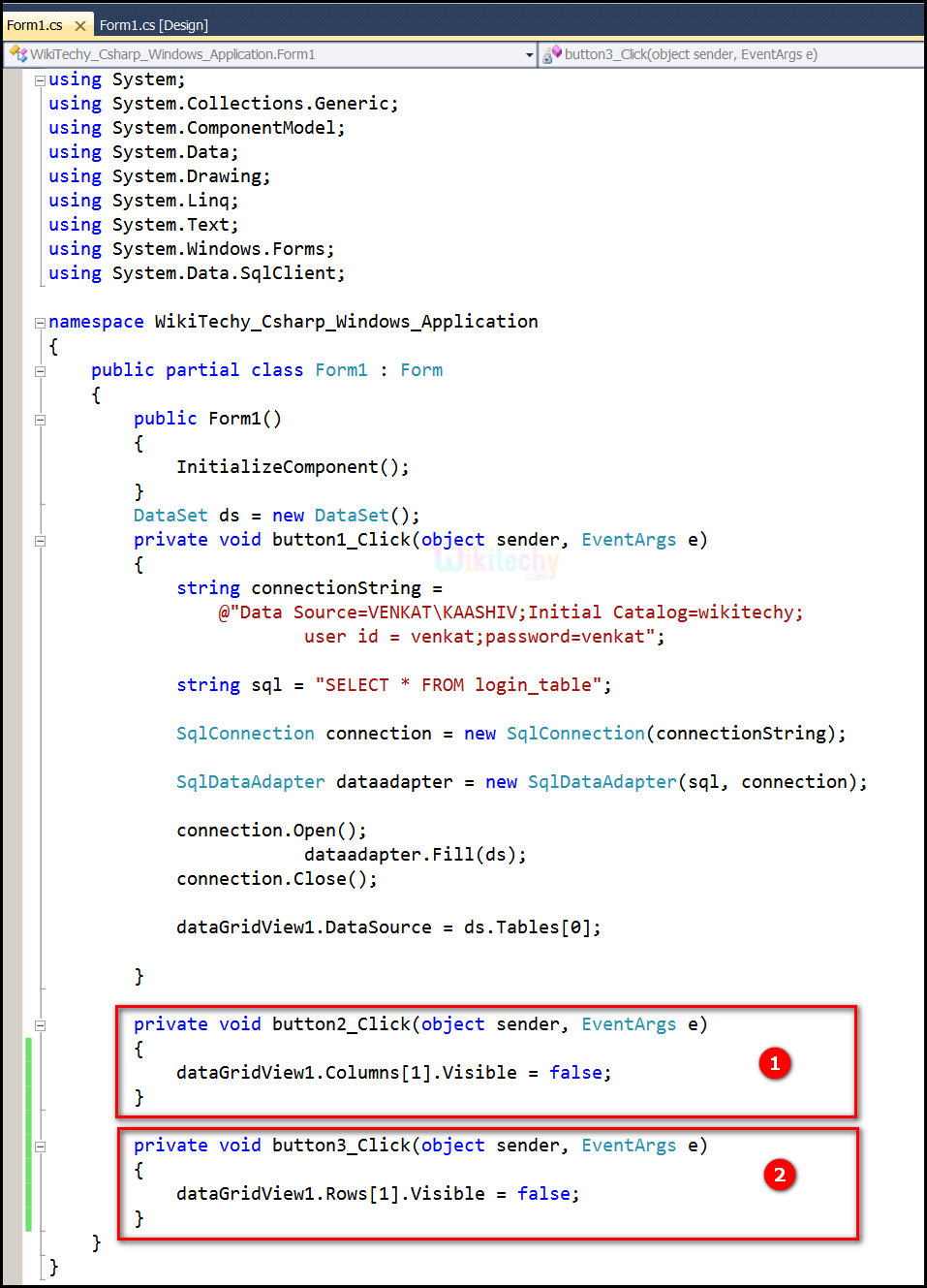
- Here private void button2_Click(object sender, EventArgs e) click event handler for button2 specifies, which is a normal Button control in Windows Forms.The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e).Here dataGridView1.Columns[1].Visible = false; specifies DataGridViewColumn.Visible property to false. To hide a ID and name columns that is automatically generated during data binding.
- Here private void button3_Click(object sender, EventArgs e) click event handler for button3 specifies, which is a normal Button control in Windows Forms.The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button3_Click(object sender, EventArgs e).Here dataGridView1.Rows[1].Visible = false; specifies DataGridViewRows.Visible property to false. To hide a ID and name rows that is automatically generated during data binding.
Sample C# examples - Output :
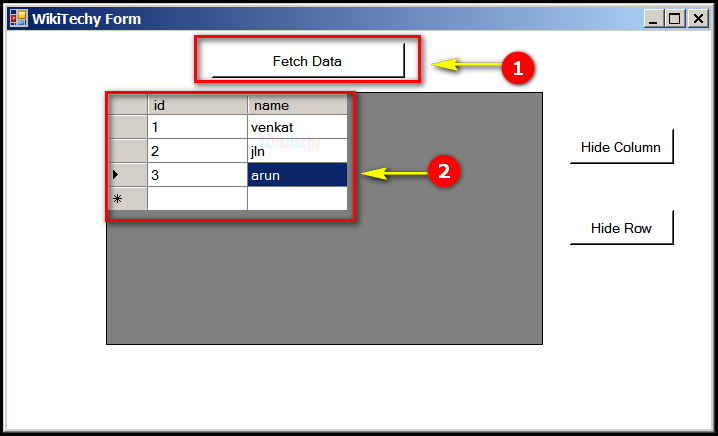
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1,2,3" and name "venkat, jln, arun".
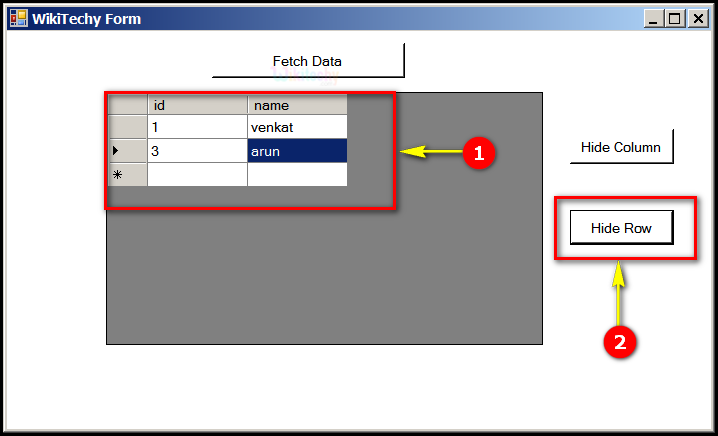
- Here in this output table displays the Id "1,3" and name "venkat, arun".
- Here in this output we display Hide Row, the data row "jln" is hided in the DataTable.
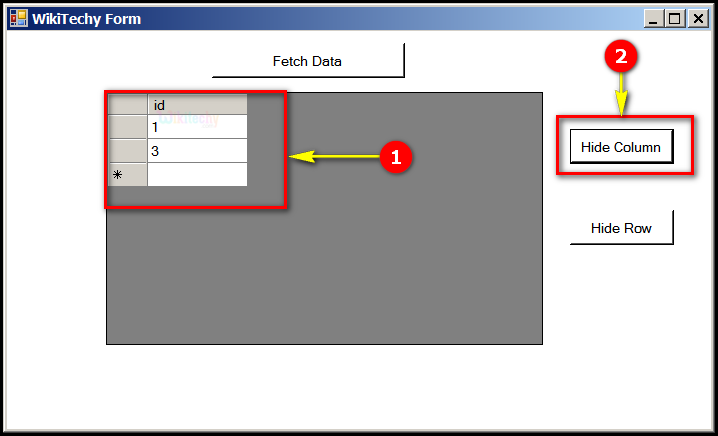
- Here in this output table displays the Id "1,3" .
- Here in this output we display Hide Column, the data column is Hided in DataTable.