C# Assignment Operators | c# assignment - c# - c# tutorial - c# net
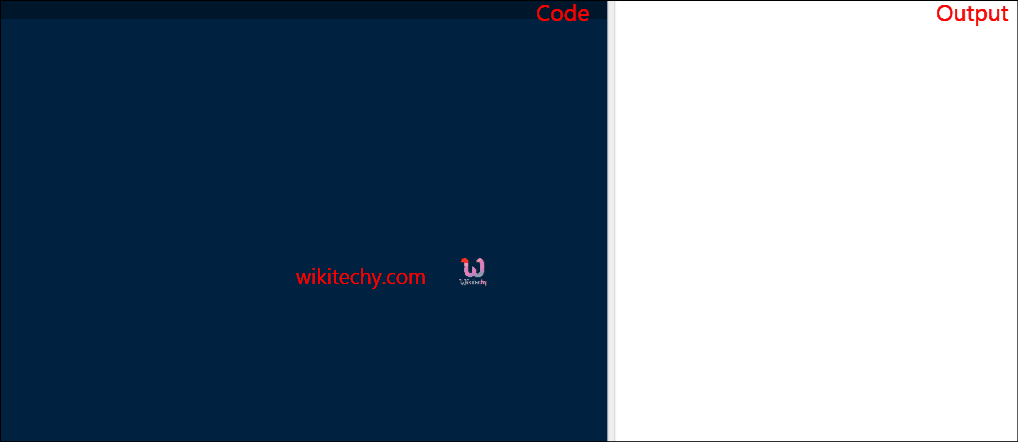
C# Assignment Operators
What is the use of assignment operator in C# ?
- In C# programs, values for the variables are assigned using assignment operators (=).
- The assignment operators assign a new value to a variable, a property, an event, or an indexer element.
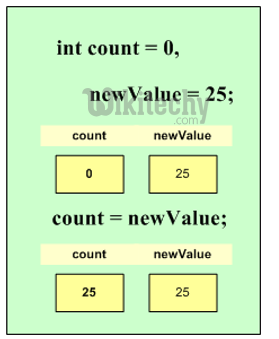
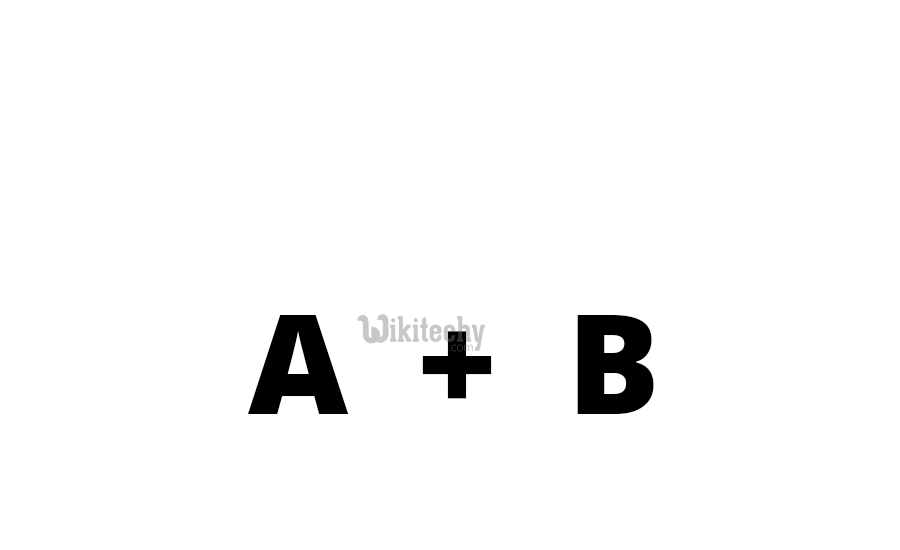
Assignment Operator
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_assignment_operator
{
class Program
{
static void Main(string[] args)
{
int a = 10;
int b;
b = a;
Console.WriteLine("\n\nThe value of b is = {0}", b);
b += a ;
Console.WriteLine("\n\nAdd value is = {0}", b);
b -= a;
Console.WriteLine("\n\nSubtraction value is = {0}", b);
b *= a;
Console.WriteLine("\n\nMultiplication value is ={0}", b);
b %= a;
Console.WriteLine("\n\nModulus value is = {0}", b);
Console.ReadLine();
}
}
}
Code Explanation:
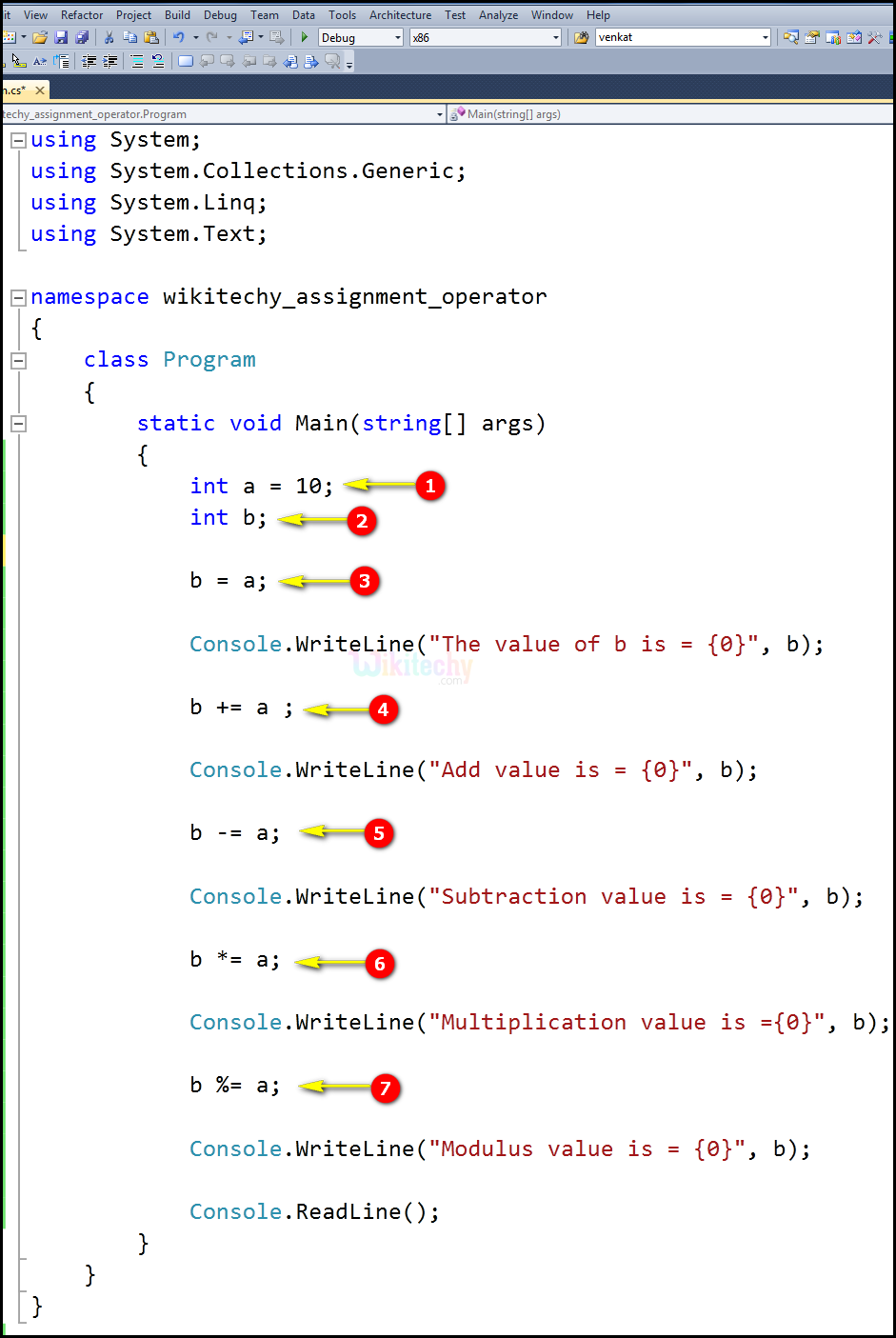
- int a = 10 is an integer variable value as 10.
- int b is an integer variable.
- In this statement we are assigning the "a" variable value 10 to "b" Variable.
- In this statement, we are performing an addition operation that b+=a (10+10=20) and the value will be assigned for the variable "b" =20.
- In this statement, we are performing a subtraction operation that b-=a (20-10=10) and the value will be assigned for the variable "b" =10.
- In this statement, we are performing a multiplication operation that b*=a (10*10=100) and the value will be assigned for the variable "b" =100.
- In this statement, we are performing a mod operation that b%=a (10%10=0) and the value will be assigned for the variable "b" =0.
Sample C# examples - Output :
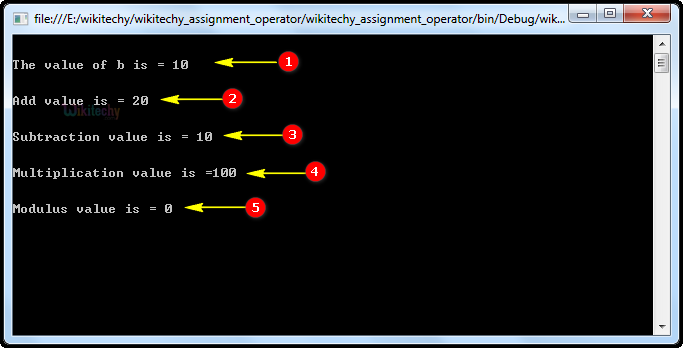
- Here in this output we have shown the assignment operation of variable "The value of b is = 10" where its output value will be displayed.
- Here in this output we have shown the addition operation of variable "b" & "a" (10+10 =20) where its output value of the variable "Addition value is = 10" will be displayed.
- Here in this output we have shown the subtraction operation of variable "b" & "a" (20-10=10) where its output value of the variable "Subtraction is = 10" will be displayed.
- Here in this output we have shown the multiplication operation of variable "b" & "a" (10*10=100) where its output value of the variable "Multiplication is = 100" will be displayed.
- Here in this output we have shown the mod operation of variable "b" & "a" (10/10=0) where its output value of the variable "Modulus is = 0" will be displayed.
c# Operator precedence :
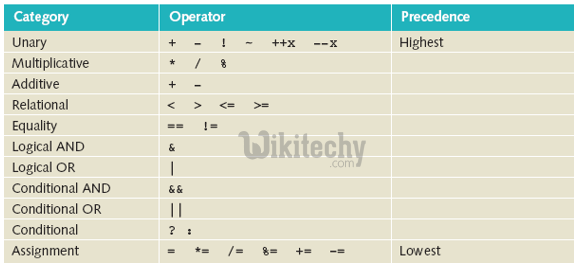