C# Timer - c# - c# tutorial - c# net
What is C# Timer ?
- In C#, the Timer Control plays a main part in the development of programs between Client side and Server side development as well as in Windows Services.
- With the Timer Control we can increase events at a specific interval of time without the interface of another thread.
- We have to use Timer Object when we want to set an interval between events, periodic checking, to start a process at a fixed time schedule, to increase or decrease the speed in an animation graphics with time schedule etc.
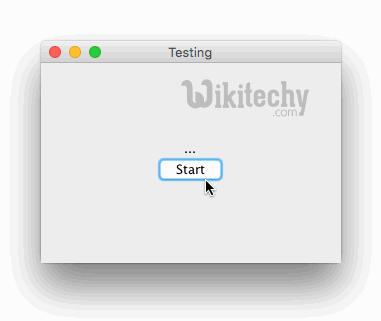
timer control in c# Example
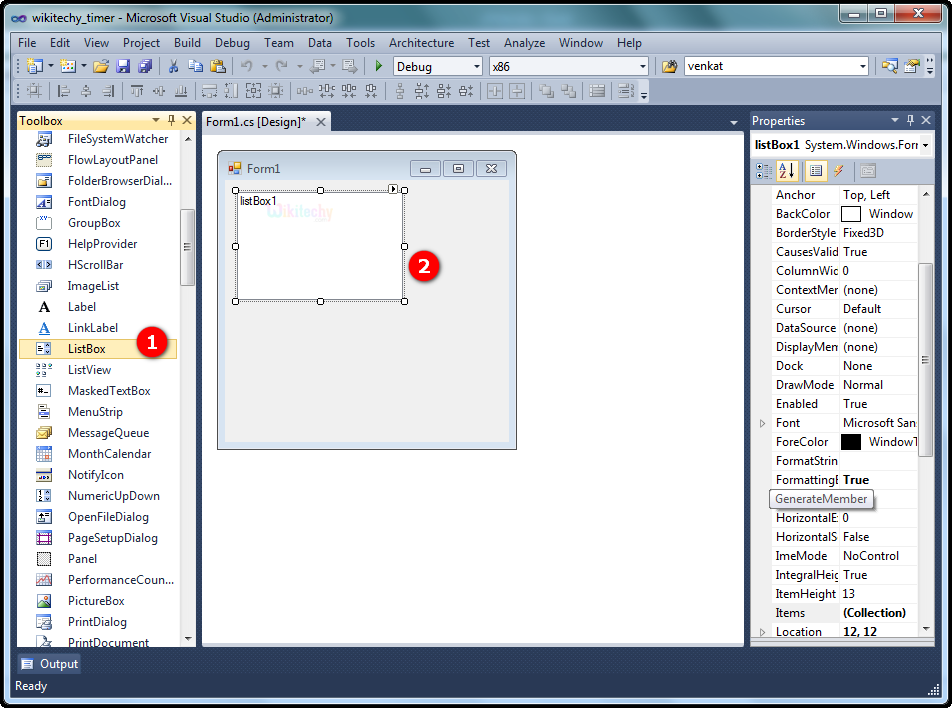
- Go to tool box and select the ListBox option.
- After dragging and dropping a ListBox component from Toolbox to a Form.
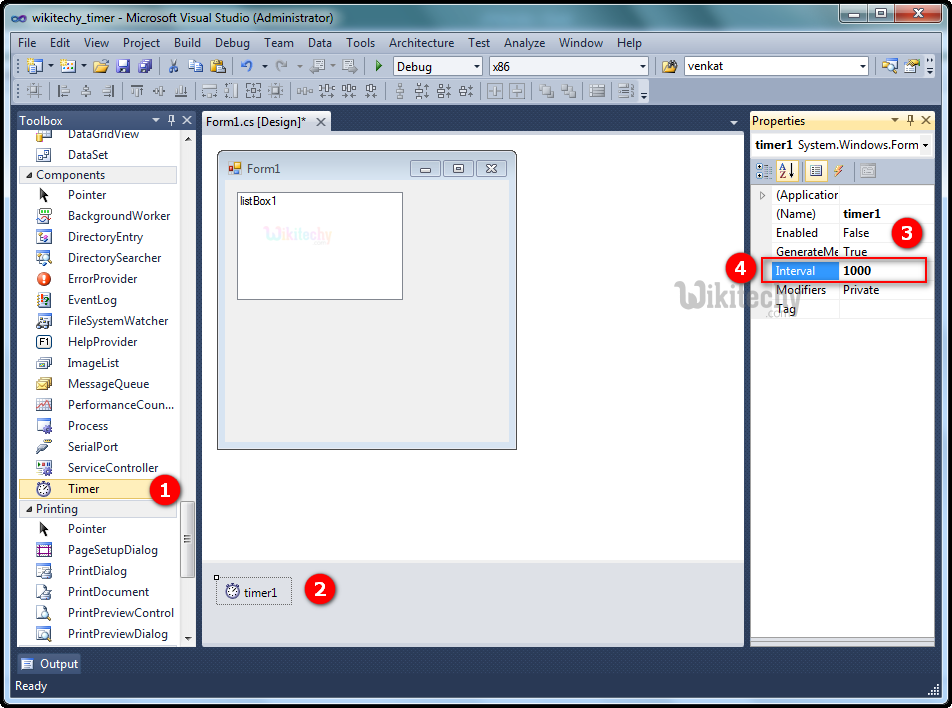
- Go to tool box and select the Timer option.
- After dragging and dropping a Timer component from Toolbox to a Form.
- After that, right click on Timer1 select Properties menu to set a Timer property. The Enabled property is false.
- In properties, Interval is set to 1000 milliseconds (1 second).
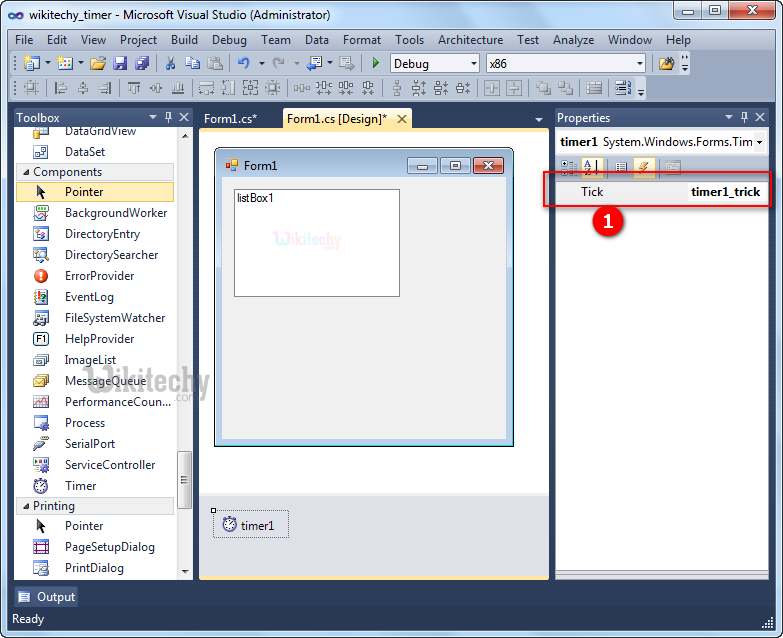
- Go to properties and add an event handler. If we go to the Events window by clicking events icon, we will see only one Tick Event and double click on it will add the Tick event handler.
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace wikitechy_timer
{
public partial class Form1 : Form
{
int second = 0;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
timer1.Interval = 1000;
timer1.Start();
}
private void timer1_trick(object sender, EventArgs e)
{
listBox1.Items.Add(DateTime.Now.ToLongTimeString() + "," + DateTime.Now.ToLongDateString());
second = second + 1;
if (second >= 10)
{
timer1.Stop();
MessageBox.Show("Terminate from Timer....");
}
}
}
}
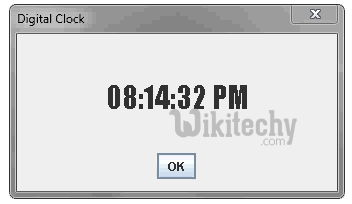
Code Explanation:
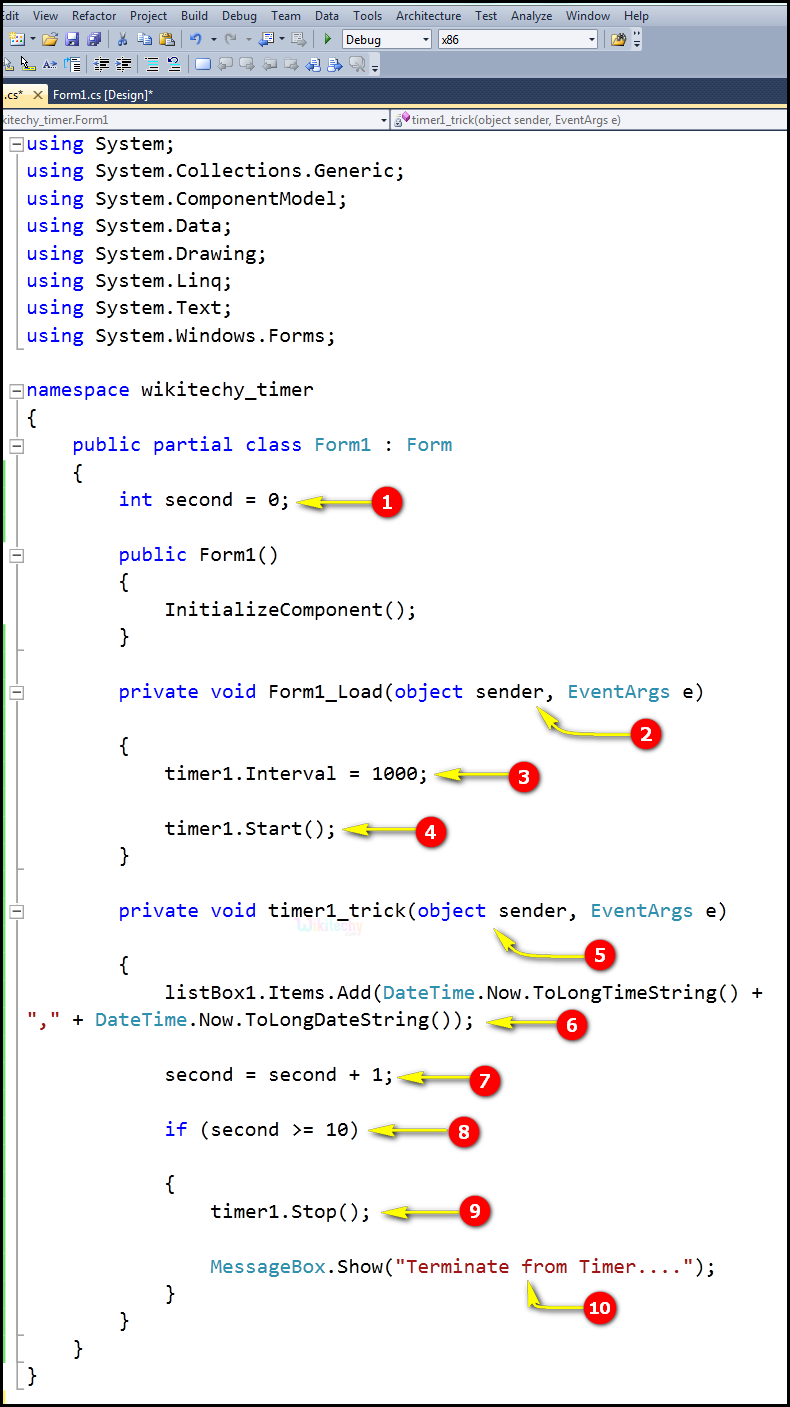
- Here we declare the integer variables second and assigning the value of the variable 0.
- Here private void Form1_Load(object sender, EventArgs e) click event handler for form specifies, which is a form control in Windows Forms.The two parameters, "object sender" and "EventArgs e" parameters of function of event of a form such as private void Form1_Load(object sender, EventArgs e).
- Here timer1.Interval = 1000; we set Timer interval as 1000 that represents the 1second That is, one second is equal to 1000 milliseconds. (1 sec = 1000 milliseconds).
- timer1.Start(); it checks weather each seconds for stopping Timer Control after 10 seconds.
- private void timer1_trick(object sender, EventArgs e) specifies to write the timer's tick event to write the current time to the text file.
- In event Handler, it will be executed in every 1 second. If we have a ListBox control on a Form and we want to add some items to it. (i.e., DateTime).
- The second initial value is 0, and it gets incremented by 1 until it reaches the 10 seconds.
- Here, if (second >= 10) expression specifies the second =0 is greater than, or equal to 10. i.e. (0>=10).
- The Timer control have included the Stop methods for stop the Timer control functions.
- MessageBox.Show("Terminate from Timer...."); which represents "Terminate from Timer...." message to the user.
Sample C# examples - Output :
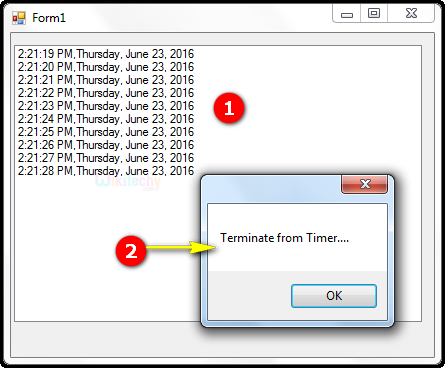
- In this example we run this program only 10 seconds. This represents the current time, date, month and year, here we set Timer interval as 1000 (1 second) and check each seconds for stopping the Timer Control after 10 seconds.
- In this output table displays the "Terminate from Timer…" in the message box window.