C# Stack - c# - c# tutorial - c# net
What is Stack in C# ?
- C# Stack<T> class is used to push and pop elements.
- It uses the concept of Stack that arranges elements in LIFO (Last in First Out) order.
- It can have duplicate elements.
- It is found in System.Collections.Generic namespace.
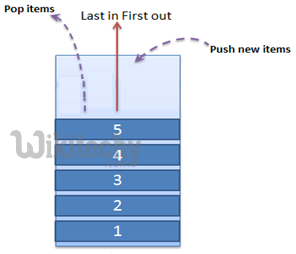
C# Stack<T> example:
- Let's see an example of generic Stack
class that stores elements using Push() method, removes elements using Pop() method and iterates elements using for-each loop
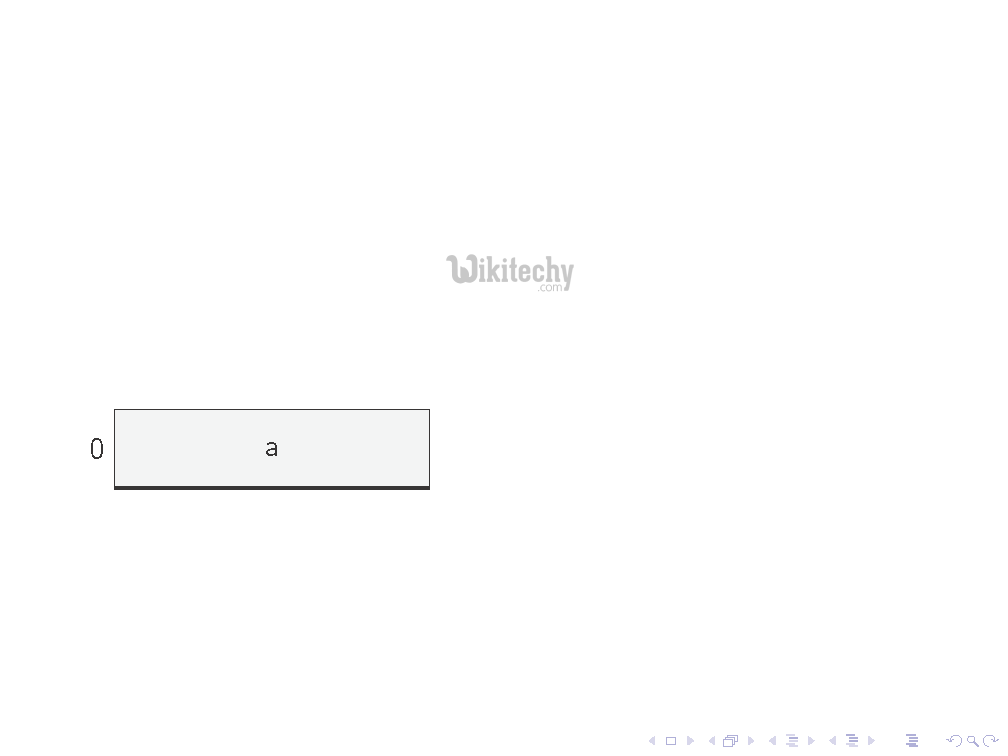
Stack
C# Sample Code - C# Examples:
using System;
using System.Collections.Generic;
public class StackExample
{
public static void Main(string[] args)
{
Stack<string> names = new Stack<string>();
names.Push("Sonoo");
names.Push("Peter");
names.Push("James");
names.Push("Ratan");
names.Push("Irfan");
foreach (string name in names)
{
Console.WriteLine(name);
}
Console.WriteLine("Peek element: "+names.Peek());
Console.WriteLine("Pop: "+ names.Pop());
Console.WriteLine("After Pop, Peek element: " + names.Peek());
}
}
C# examples - Output :
Sonoo
Peter
James
Ratan
Irfan
Peek element: Irfan
Pop: Irfan
After Pop, Peek element: Ratan
C# program that creates new Stack of integers
using System;
using System.Collections.Generic;
class Program
{
static Stack<int> GetStack()
{
Stack<int> stack = new Stack<int>();
stack.Push(100);
stack.Push(1000);
stack.Push(10000);
return stack;
}
static void Main()
{
var stack = GetStack();
Console.WriteLine("--- Stack contents ---");
foreach (int i in stack)
{
Console.WriteLine(i);
}
}
}
Output
--- Stack contents ---
10000
1000
100