C# Gridview Sorting - c# - c# tutorial - c# net
What is Datagridview Sorting?
- In general, the DataGridView control provides automatic sorting, so that user can manually sort any column in the control.
- Here we can control whether a column can be sorted by setting the SortMode property of the DataGridViewColumn.
- We can also programmatically sort a column.
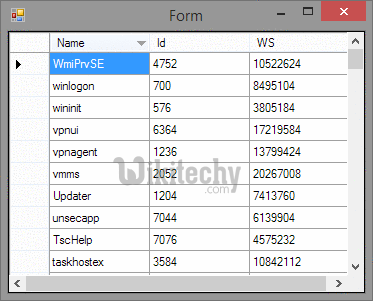
DataGridview Sorting
How to sort Datagridview
- In C#, the DataGridView control provides an automatic sorting, so that we can automatically sort any column in the datagridview control.
- We can sort the data in ascending or descending order based on the contents of the specified column.
- Also we can see the DataGridView sorting, when a user clicks on the column header.
Syntax
dataGridView1.Sort(dataGridView1.Columns[1], ListSortDirection.Ascending);
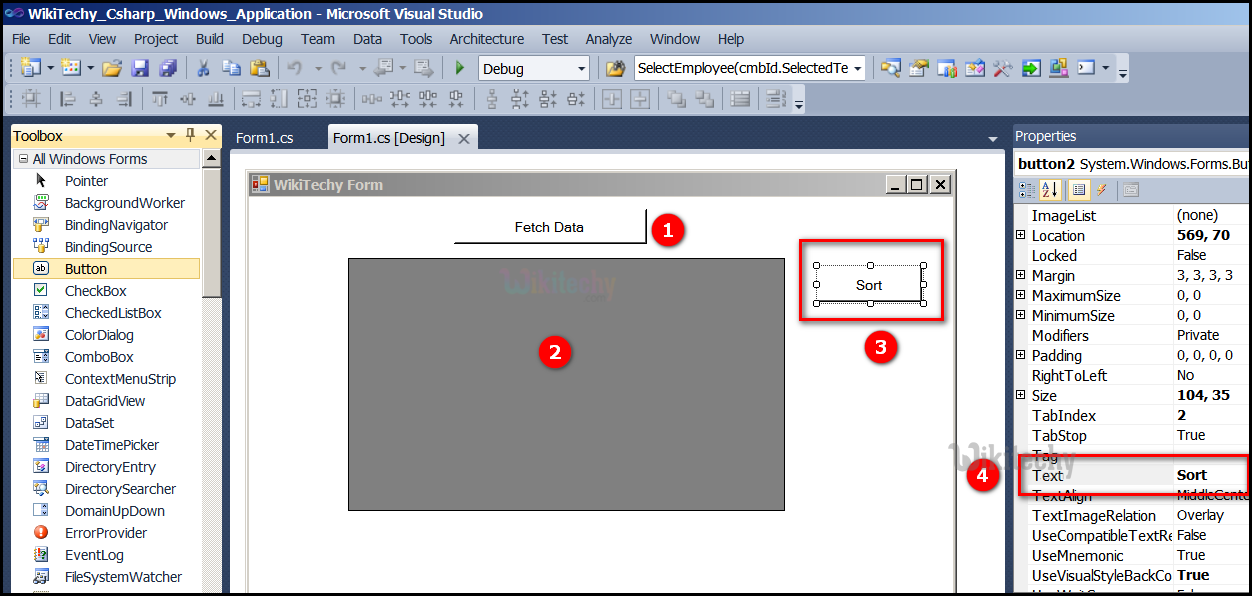
- Here Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Go to tool box and click on the DataGridview option the form will be open.
- Go to tool box and click on the button option and put the name as Sort in the button.
- Here thetext field is specifying to enter the text in text field.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
DataSet ds = new DataSet();
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
dataGridView1.Sort(dataGridView1.Columns[1], ListSortDirection.Ascending);
}
}
}
Code Explanation:
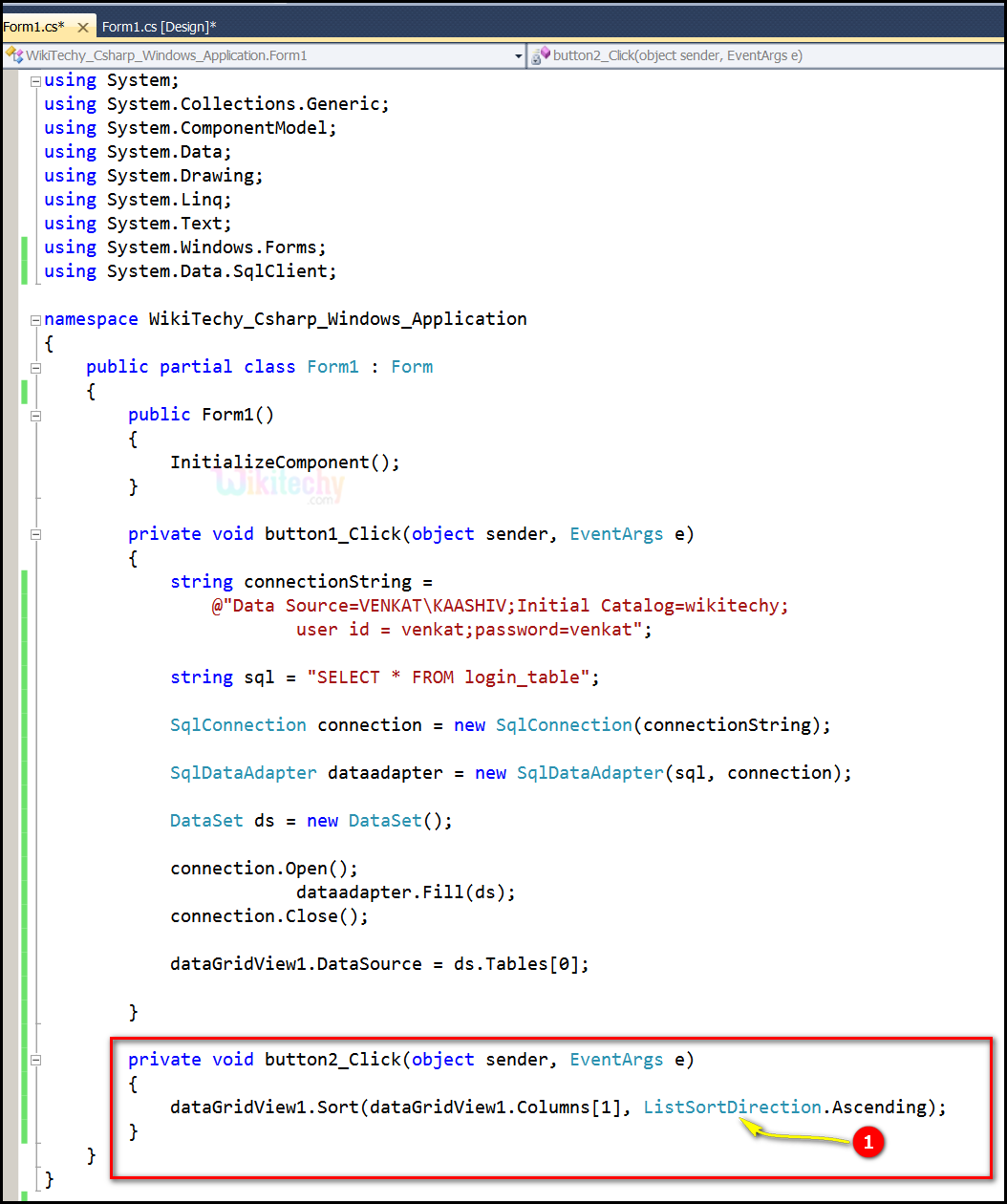
- Here private void button2_Click(object sender, EventArgs e) click event handler for button2 specifies, which is a normal Button control in Windows Forms. The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e). Here dataGridView1.Sort(dataGridView1.Columns[1], ListSortDirection.Ascending); specifies to Sorts the contents of the DataGridView control in ascending order based on the contents of the specified column.
Sample Output
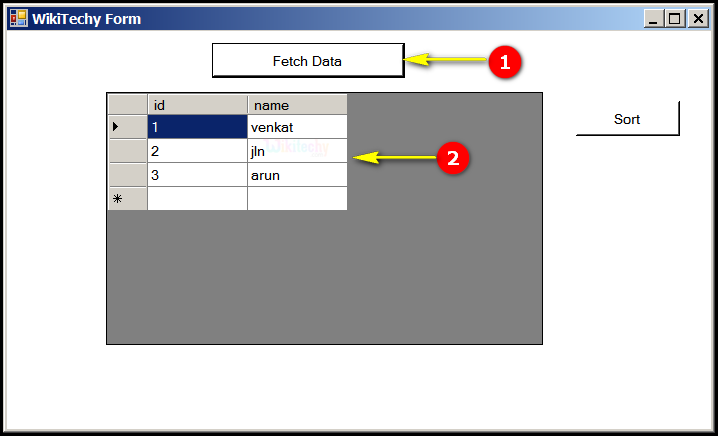
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "1,2,3" and name "venkat, jln, arun".
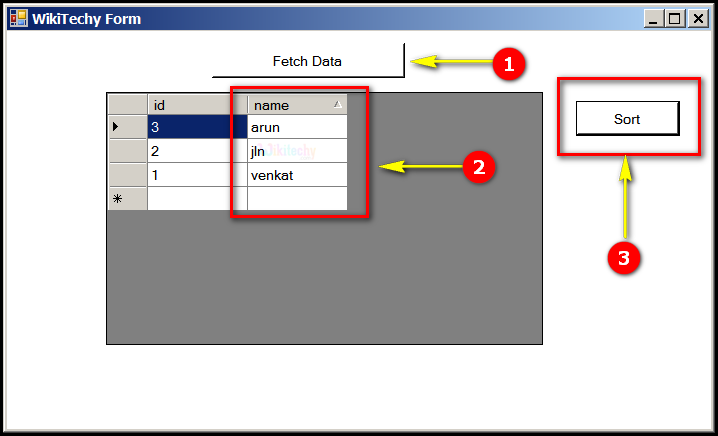
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Id "3,2,1" and name "arun, jln, venkat".
- Here in this output we display Sort, which specifies click on Sort button then the table id’s and names are converted into ascending order (A to Z).