Delegates in C# - c# - c# tutorial - c# net
What is Delegates in C#?
- Delegates in C# are similar to function or pointers which are given in C or C++
- Hence this is said to be a reference type variable which can be defined as per how to hold the reference to a method
- The reference in C# can be changed only at runtime and it cannot be changed at compile time.
- They are used specifically for implementing events and they are also used for call-back methods.
- System.Delegate class is used to implicitly derived all delegates in C#
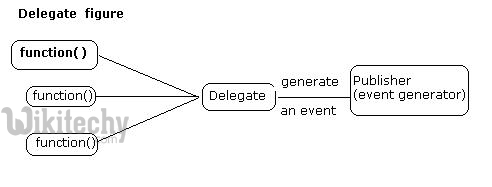
process in c# delegate
Declaring Delegates
- This delegate declaration determines the method which can be referenced by the delegate in C#
- Declaring Delegates can be refer to a method which can have the same signature as a delegate in C#
- Here is an example as per how to declare a delegate
Example:
public delegate int MyDelegate (string s);
Description of the Example:
- The delegate which is given in the example can be used to reference any method which can return a int type variable and also has a single string parameter
Syntax:
delegate <return type> <delegate-name> <parameter list>
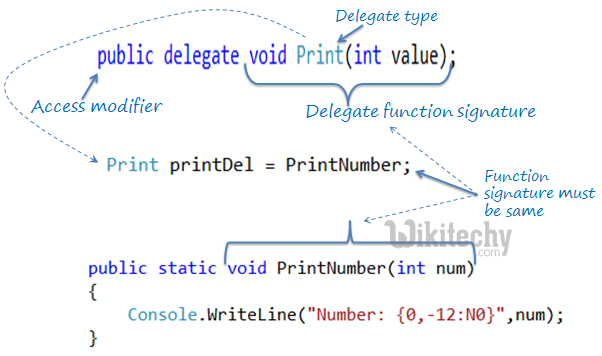
declaration in c# delegate
Instantiating Delegates
- After the delegate is declared, the delegate object should be created with a new keyword and also it should be associated with a particular method in C#
- The argument is passed to the new expression which is similarly written to a method call, but it is done without arguments to the method
- Here is an example to instantiate delegate:
Example:
public delegate void printString(string s);
...
printString ps1 = new printString(WriteToScreen);
printString ps2 = new printString(WriteToFile);
Description of the program:
- The program which is given below is a program which demonstrates declaration and instantiation of a delegate.
- This program is used for reference methods which takes an integer parameter and it returns an integer value
Program:
using System;
delegate int NumberChanger(int n);
namespace DelegateAppl
{
class TestDelegate
{
static int num = 10;
public static int AddNum(int p)
{
num += p;
return num;
}
public static int MultNum(int q)
{
num *= q;
return num;
}
public static int getNum()
{
return num;
}
static void Main(string[] args)
{
//create delegate instances
NumberChanger nc1 = new NumberChanger(AddNum);
NumberChanger nc2 = new NumberChanger(MultNum);
//calling the methods using the delegate objects
nc1(25);
Console.WriteLine("Value of Num: {0}", getNum());
nc2(5);
Console.WriteLine("Value of Num: {0}", getNum());
Console.ReadKey();
}
}
}
Output:
Value of Num: 35
Value of Num: 175
Multicasting of a Delegate
- Here are the properties of delegates which are used to invoke a delegate by using invocation list of methods which are used in delegates.
- Delegate objects are objects which can be composed by using the "+" operator in C#
- Delegate calls are composed and it can call two delegates where the two delegates are composed.
- Delegates of the same type can be composed while delegates of different type cannot be composed
- There is a way to remove a component delegate from a composed delegate and this can be using the "-" operator
- This method which is used to invoke a delegate by using invocation list of methods and also by using the properties of delegates is known as multicasting of a delegate.
- The program which is given below show us the demonstration of a delegate
Read Also
dot net training and placement institutes , dot net course in chennai with placement , dot net course fee in chennaiProgram:
using System;
delegate int NumberChanger(int n);
namespace DelegateAppl
{
class TestDelegate
{
static int num = 10;
public static int AddNum(int p)
{
num += p;
return num;
}
public static int MultNum(int q)
{
num *= q;
return num;
}
public static int getNum()
{
return num;
}
static void Main(string[] args)
{
//create delegate instances
NumberChanger nc;
NumberChanger nc1 = new NumberChanger(AddNum);
NumberChanger nc2 = new NumberChanger(MultNum);
nc = nc1;
nc += nc2;
//calling multicast
nc(5);
Console.WriteLine("Value of Num: {0}", getNum());
Console.ReadKey();
}
}
}
Output:
Value of Num: 75
Use of a Delegate
- It can be used to reference methods which return nothing and takes string as input and this can be used for delegate printString
- Hence we used this delegate printString to call two methods and hence they are
- The first method is used to print the string to the console
- The second method prints to the file
- The program which is given us demonstrate the use of delegate
Program:
using System;
using System.IO;
namespace DelegateAppl
{
class PrintString
{
static FileStream fs;
static StreamWriter sw;
// delegate declaration
public delegate void printString(string s);
// this method prints to the console
public static void WriteToScreen(string str)
{
Console.WriteLine("The String is: {0}", str);
}
//this method prints to a file
public static void WriteToFile(string s)
{
fs = new FileStream("c:\\message.txt",
FileMode.Append, FileAccess.Write);
sw = new StreamWriter(fs);
sw.WriteLine(s);
sw.Flush();
sw.Close();
fs.Close();
}
// this method takes the delegate as parameter and uses it to
// call the methods as required
public static void sendString(printString ps)
{
ps("Hello World");
}
static void Main(string[] args)
{
printString ps1 = new printString(WriteToScreen);
printString ps2 = new printString(WriteToFile);
sendString(ps1);
sendString(ps2);
Console.ReadKey();
}
}
}
Output:
The String is: Hello World