C# Windows Application Project
Combo Box
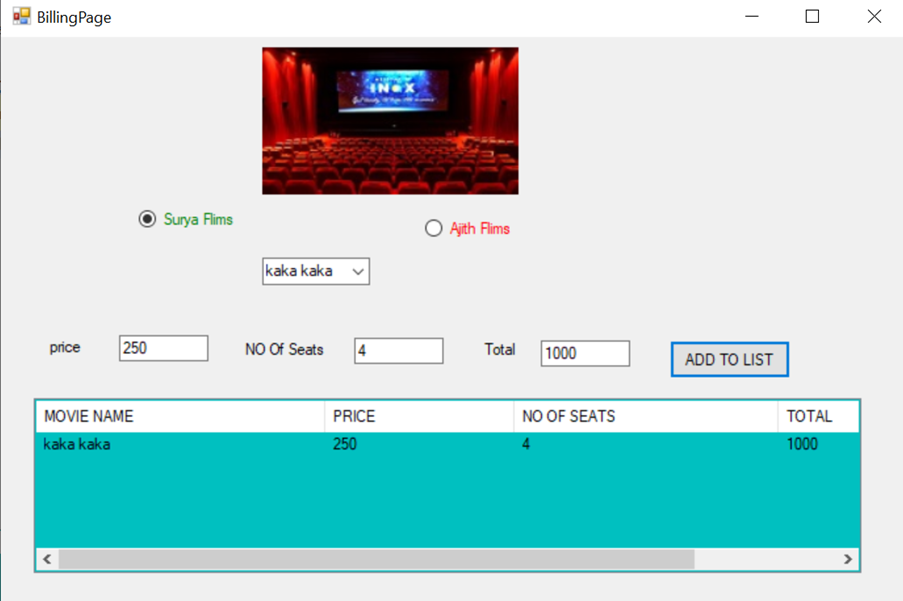
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsForm_octoberbatch23
{
public partial class BillingPage : Form
{
public BillingPage()
{
InitializeComponent();
}
private void rdb_1_CheckedChanged(object sender, EventArgs e)
{
rdb_1.ForeColor = System.Drawing.Color.Green;
rdb_2.ForeColor = System.Drawing.Color.Red;
comboBox1.Items.Clear();
comboBox1.Items.Add("kaka kaka");
comboBox1.Items.Add("varanum aayiram");
comboBox1.Items.Add("NGK");
}
private void rdb_2_CheckedChanged(object sender, EventArgs e)
{
rdb_1.ForeColor = System.Drawing.Color.Red;
rdb_2.ForeColor = System.Drawing.Color.Green;
comboBox1.Items.Clear();
comboBox1.Items.Add("viswasam");
comboBox1.Items.Add("Arrambam");
comboBox1.Items.Add("Dheena");
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
if (comboBox1.SelectedItem.ToString() == "kaka kaka")
{
txt_price.Text = "250";
}
else if (comboBox1.SelectedItem.ToString() == "varanum aayiram")
{
txt_price.Text = "500";
}
else if (comboBox1.SelectedItem.ToString() == "NGK")
{
txt_price.Text = "170";
}
else if (comboBox1.SelectedItem.ToString() == "viswasam")
{
txt_price.Text = "120";
}
else if (comboBox1.SelectedItem.ToString() == "Arrambam")
{
txt_price.Text = "400";
}
else if (comboBox1.SelectedItem.ToString() == "Dheena")
{
txt_price.Text = "300";
}
else
{
txt_price.Text = "0";
}
txt_total.Text = "";
txt_noOfSeats.Text = "";
}
private void txt_noOfSeats_TextChanged(object sender, EventArgs e)
{
if (txt_noOfSeats.Text.Length > 0)
{
txt_total.Text = (Convert.ToInt16(txt_price.Text) *
Convert.ToInt16(txt_noOfSeats.Text)).ToString();
}
}
private void btn_add_Click(object sender, EventArgs e)
{
string[] arr = new string[4];
arr[0] = comboBox1.SelectedItem.ToString();
arr[1] = txt_price.Text;
arr[2] = txt_noOfSeats.Text;
arr[3] = txt_total.Text;
ListViewItem lv = new ListViewItem(arr);
listView1.Items.Add(lv);
}
}
}
CheckList Box
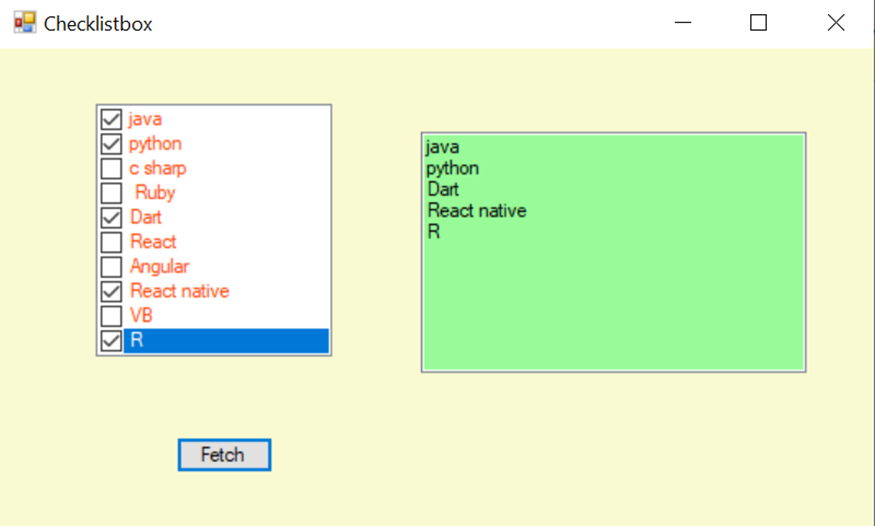
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Gridview
{
public partial class Check_listbox : Form
{
public Check_listbox()
{
InitializeComponent();
}
private void btn_fetch_Click(object sender, EventArgs e)
{
// listBox1.Items.Clear();
listBox1.Items.Clear();
foreach (String programs in checkedListBox1.CheckedItems)
listBox1.Items.Add(programs);
}
private void linkLabel1_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e)
{
Pageload pd = new Pageload();
pd.Show();
this.Hide();
}
}
}
Page Loader
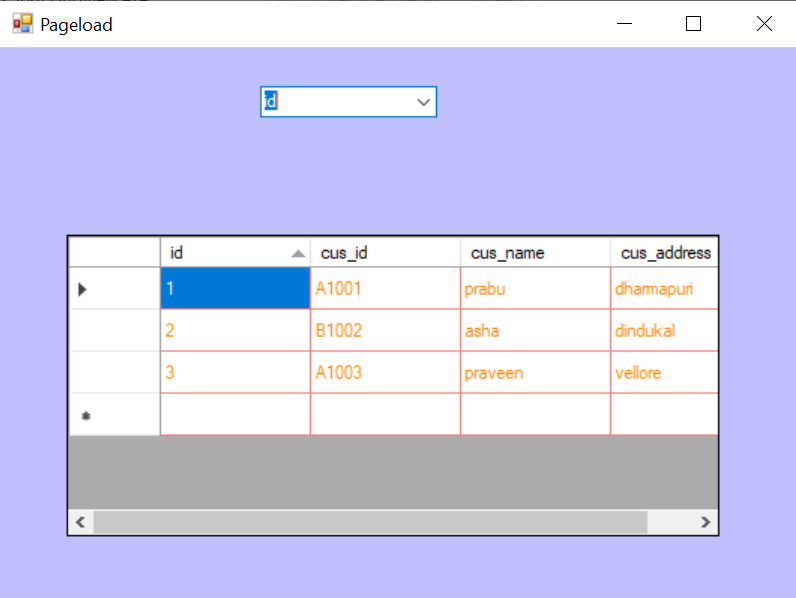
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Gridview
{
public partial class Pageload : Form
{
public Pageload()
{
InitializeComponent();
sort("id");
}
private void sort(String value)
{
SqlConnection con = new SqlConnection(@"Data Source=DESKTOP-UA2SORD;Initial Catalog=window_octbatch;Integrated Security=True");
con.Open();
SqlCommand cmd = new SqlCommand("sp_cusfetch", con);
cmd.CommandType = CommandType.StoredProcedure;
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
//dataGridView1.DataSource = ds.Tables[0];
DataView dv=new DataView(ds.Tables[0]);
dv.Sort = value;
dataGridView1.DataSource = dv;
con.Close();
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
sort(comboBox1.SelectedItem.ToString());
}
private void Pageload_Load(object sender, EventArgs e)
{
Form2 fm = new Form2();
fm.Show();
this.Hide();
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
}
}
Group Box
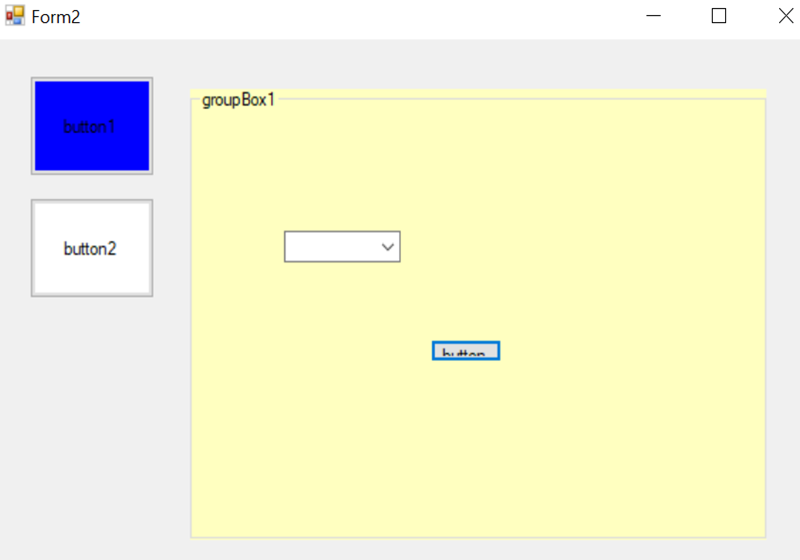
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsForm_octoberbatch23
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
groupBox1.Visible = true;
groupBox2.Visible = false;
button1.BackColor = Color.Blue;
button2.BackColor = Color.White;
}
private void button2_Click(object sender, EventArgs e)
{
groupBox1.Visible = false;
groupBox2.Visible = true;
button1.BackColor = Color.White;
button2.BackColor = Color.Blue;
}
private void Form2_Load(object sender, EventArgs e)
{
groupBox1.Visible = true;
groupBox2.Visible = false;
}
}
}
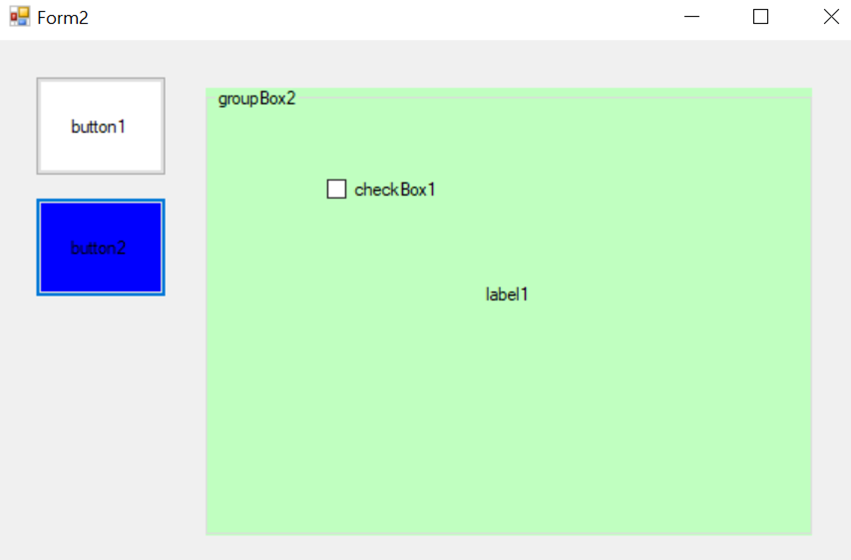